How can I print a circular structure in a JSON-like format?
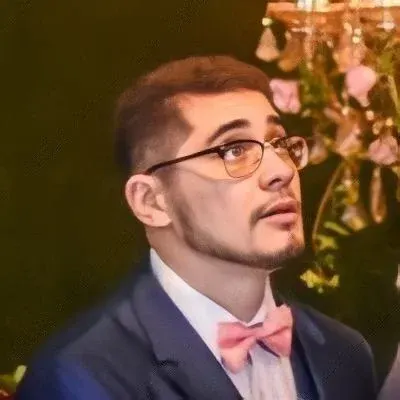
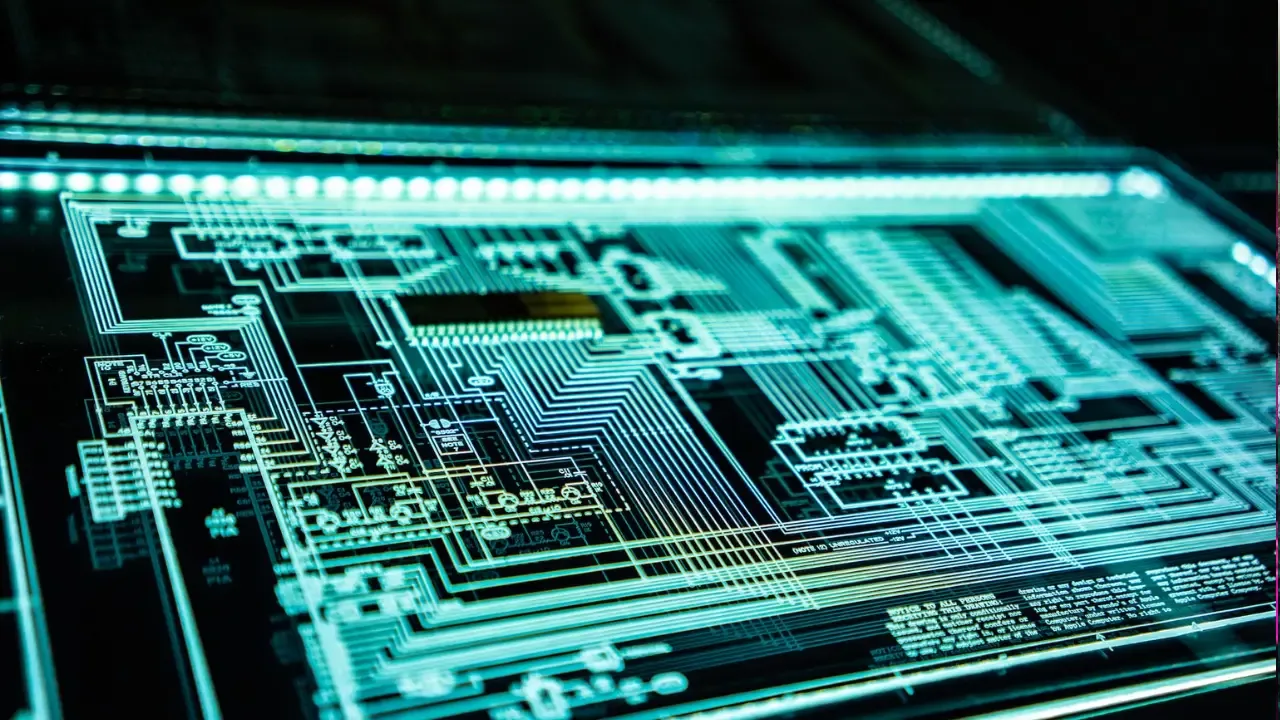
How to Print a Circular Structure in a JSON-like Format
Are you trying to convert a large object into JSON format but encountering circular references along the way? 😫 Don't worry, we've got you covered! In this guide, we'll walk you through common issues and provide easy solutions to print a circular structure in a JSON-like format. Let's dive in! 🚀
Understanding the Problem
When attempting to JSON.stringify()
an object with circular references, you might encounter two common errors:
TypeError: Converting circular structure to JSON
TypeError: cyclic object value
These errors occur because JSON format does not support circular references. A circular reference is when an object refers back to itself or to another object that ultimately loops back to it.
The Scenario
Let's consider the following scenario provided by the question:
var obj = {
a: "foo",
b: obj
}
Here, the object obj
has a circular reference, as the property b
refers back to the obj
itself. When attempting to stringify this object using JSON.stringify()
, we want to obtain the following result:
{"a":"foo"}
The Solution
To solve this problem, we need to remove any circular references from the object before stringifying it. We can achieve this by using a custom replacer function when calling JSON.stringify()
.
The custom replacer function will be invoked for each property in the object being stringified. Inside the replacer function, we can check if the value of a property is itself or a reference to another property that ultimately points back to itself. If such a circular reference is detected, we can simply return undefined
to remove that property from the stringified output.
Here's how we can implement this solution:
function stringifyWithoutCircular(obj) {
const seen = new Set(); // To keep track of seen objects
return JSON.stringify(obj, function(key, value) {
if (typeof value === 'object' && value !== null) {
if (seen.has(value)) {
return undefined; // Remove circular references
}
seen.add(value);
}
return value;
});
}
const obj = {
a: "foo",
b: obj
};
const result = stringifyWithoutCircular(obj);
console.log(result); // Output: {"a":"foo"}
In this solution, we created the stringifyWithoutCircular
function that takes in an object as input and uses JSON.stringify()
with a custom replacer function. The replacer function keeps track of seen objects in the seen
set and removes any circular references encountered.
Conclusion
By using a custom replacer function, we can easily handle circular references while printing a circular structure in a JSON-like format. Now you can confidently convert your complex objects into JSON format without worrying about those pesky circular reference errors!
We hope this guide was helpful to you. If you have any further questions or need additional assistance, please don't hesitate to let us know in the comments below. Happy coding! 😄👨💻
🌟💬 Call to Action 💬🌟
Did you find this guide useful in solving your circular structure JSON printing troubles? If so, don't forget to share it with your fellow developers who might also be struggling with this issue. Together, we can make JSON serialization a breeze! 💪🤝
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
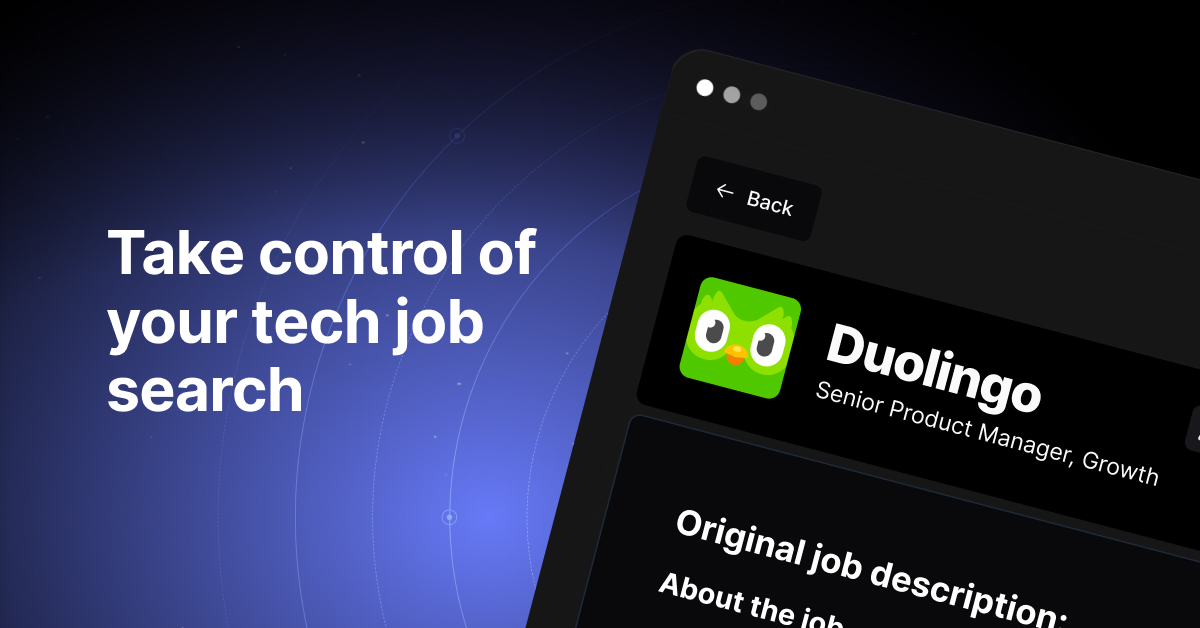