How can I mock an ES6 module import using Jest?
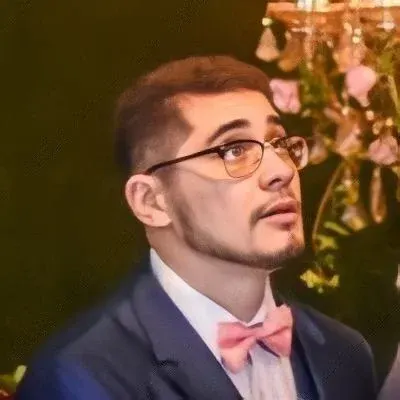
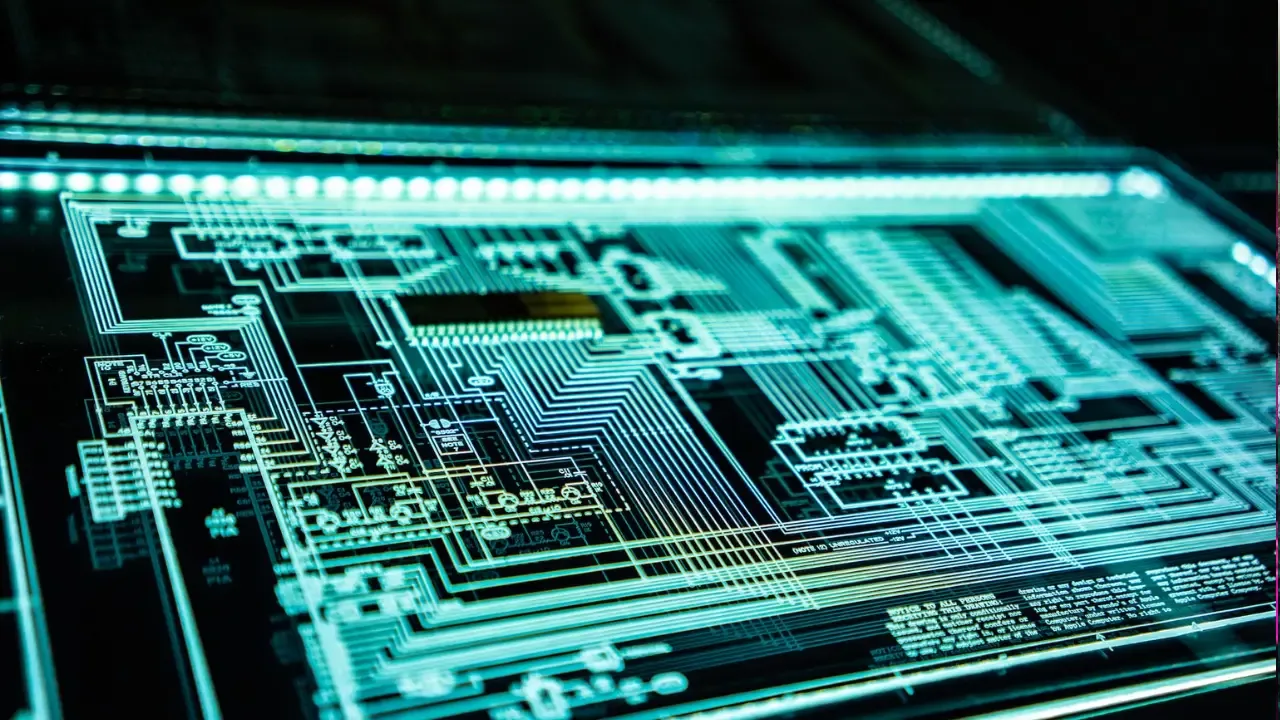
How to mock an ES6 module import using Jest? 🎭
Are you struggling to mock ES6 module imports in Jest? Don't worry, you're not alone! This common issue can be a real hair-puller, but fear not, I'm here to help you. In this article, I'll show you how to mock ES6 module imports using Jest, so you can test your code with ease.
The Challenge 💥
Let's start by understanding the problem. The challenge arises when you have a module that imports another module, and you want to mock that imported module to test the interactions between them. Traditionally, you might use Jasmine to accomplish this, but what about Jest?
The Solution 🚀
Fear not, because Jest provides a simple and elegant solution to mock ES6 module imports. Instead of manually replacing imports or using require
, Jest offers a built-in function called jest.mock()
that allows you to effortlessly mock dependencies.
Here's how you can do it:
// myModule.js
import dependency from './dependency';
export default (x) => {
dependency.doSomething(x * 2);
}
// myModule-test.js
import myModule from '../myModule';
import * as dependency from '../dependency'; // Mocked import
describe('myModule', () => {
it('calls the dependency with double the input', () => {
const spy = jest.spyOn(dependency, 'default'); // Spy on the default export mock
myModule(2);
expect(spy).toHaveBeenCalledWith(4);
});
});
By using the wildcard import syntax * as dependency
, we can mock the entire module and access its exports as properties on the dependency
object. This allows us to easily spy on the default export using jest.spyOn()
and assert that it was called correctly.
Isn't that neat? You no longer have to resort to messy require
statements or compromise the structure of your code. Jest makes it easy to mock ES6 module imports and focus on testing your logic.
Handling Default Exports 🌟
"But what if the function inside dependency.js
is a default export?" you might ask. Fear not, my friend, for Jest has got you covered! Mocking default exports is just as simple as mocking named exports.
// myModule-test.js
import myModule from '../myModule';
import * as dependency from '../dependency'; // Mocked import
jest.mock('../dependency', () => {
return {
__esModule: true,
default: jest.fn(), // Mock the default export
};
});
describe('myModule', () => {
it('calls the dependency with double the input', () => {
const spy = jest.spyOn(dependency, 'default'); // Spy on the default export mock
myModule(2);
expect(spy).toHaveBeenCalledWith(4);
});
});
By using the jest.mock()
function with an inline implementation, we can mock the default export as a Jest function (jest.fn()
). This allows us to spy on the default export and assert its behavior just like we did with named exports.
Your Turn! ✍️
Now that you know how to mock ES6 module imports using Jest, it's time to put your newfound knowledge into action! Start leveraging the power of Jest's mocking capabilities to write robust tests for your code.
Have you encountered any other testing challenges or found clever ways to mock dependencies? Share your experiences and tips in the comments below. Let's learn from each other and level up our testing game!
Happy testing! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
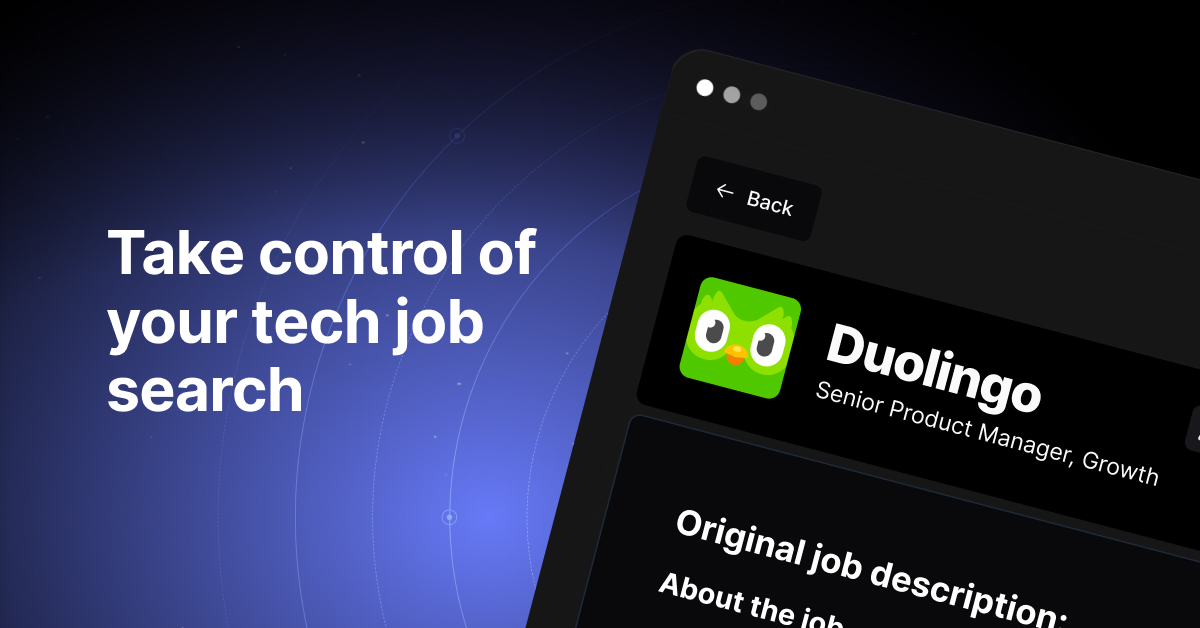