How can I merge properties of two JavaScript objects dynamically?
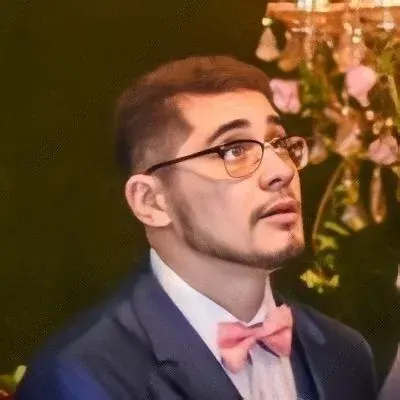
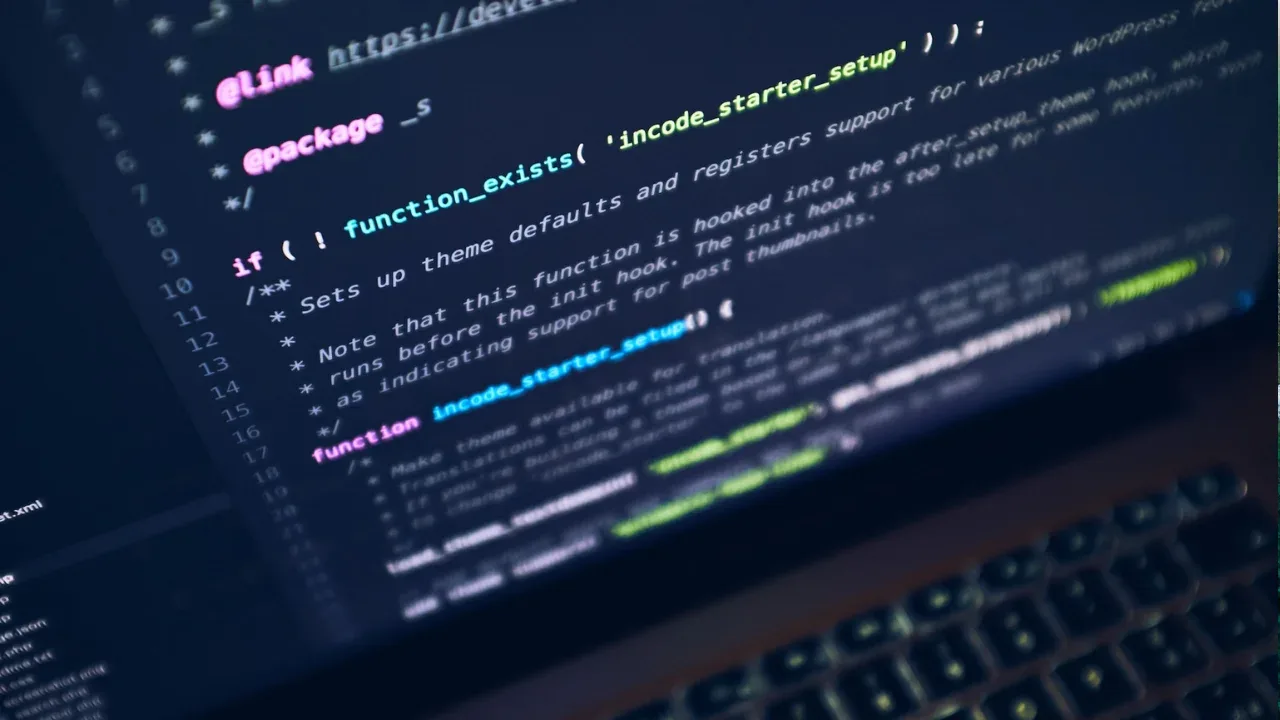
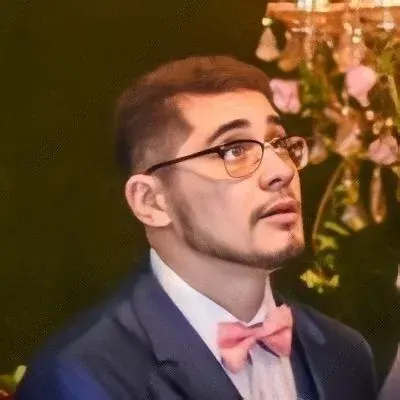
Combining Properties of JavaScript Objects Dynamically
Are you struggling to merge properties from two JavaScript objects dynamically? 🤔 Don't worry, we've got you covered! In this guide, we'll walk you through a simple solution to combine properties from two objects at runtime. Let's dive right in! 💪
The Challenge
Let's say you have two JavaScript objects: obj1
and obj2
. You need to merge the properties of obj2
into obj1
dynamically. In other words, you want to combine the properties of both objects, so that obj1
contains all the properties from obj2
.
The Solution
Fortunately, you don't need to reinvent the wheel here. JavaScript provides an easy way to merge properties using the Object.assign()
method. 🚀
var obj1 = { food: 'pizza', car: 'ford' };
var obj2 = { animal: 'dog' };
Object.assign(obj1, obj2);
console.log(obj1);
Running the code above will give you the desired output:
{ food: 'pizza', car: 'ford', animal: 'dog' }
Wow, that was easy! 👏 Object.assign()
takes the properties from obj2
and merges them into obj1
. The best part is, it's not limited to just two objects; you can merge as many objects as you want. Isn't that awesome? 😎
The Limitations
Here's something to keep in mind: Object.assign()
performs a shallow merge, which means it only copies the values of properties. If you have nested objects or arrays, they will be assigned by reference. So, modifying a nested object in obj2
will also affect obj1
.
If you don't need recursion or to merge functions like our questioner, Object.assign()
is the perfect solution for you! ✨
Take It Further
If you need a deep merge, where nested objects or arrays are cloned instead of assigned by reference, you can use third-party libraries like Lodash or jQuery to achieve that. These libraries provide more advanced merging capabilities, making your life as a developer even easier!
Conclusion
Merging properties from two JavaScript objects dynamically is a breeze with the Object.assign()
method. Remember, use it when you need a simple shallow merge without recursion or function merging. If you need to go deeper, explore the power of third-party libraries.
Now it's your turn to try it out! ✌️ Give it a go and let us know your thoughts in the comments below. And as always, happy coding! 🚀