How can I make an AJAX call without jQuery?
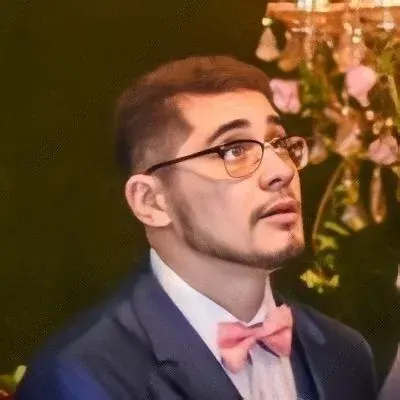
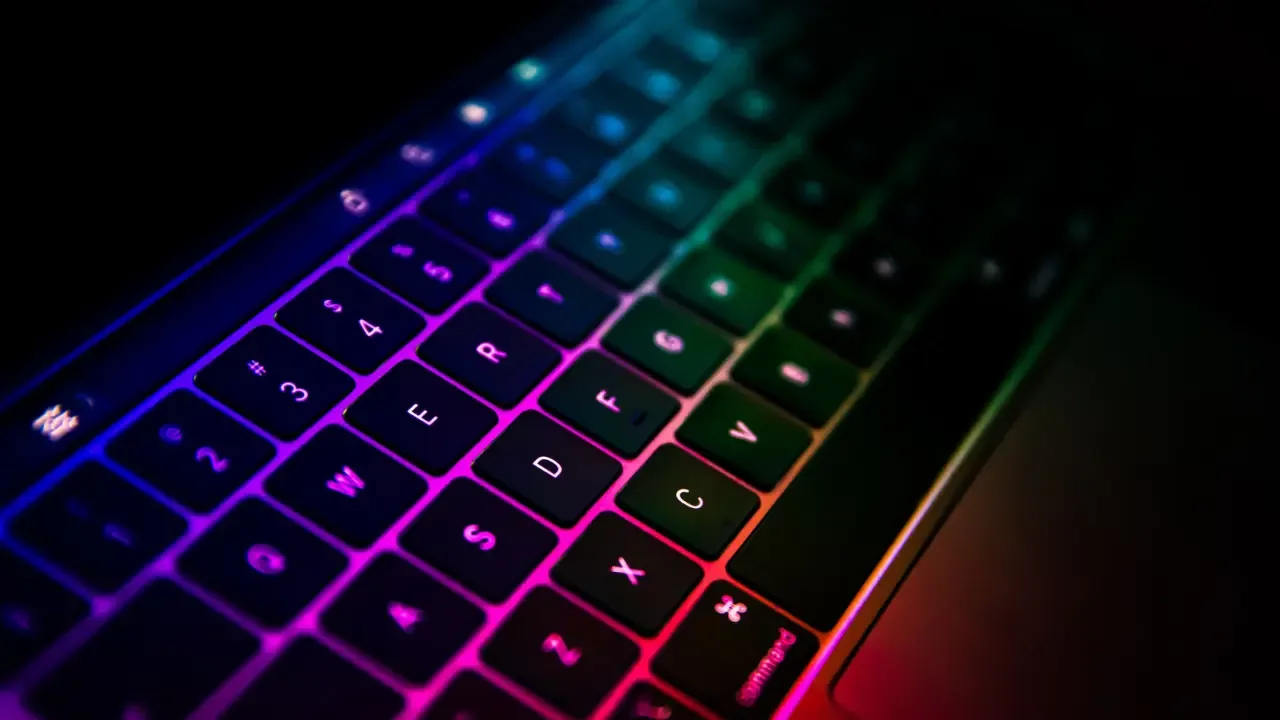
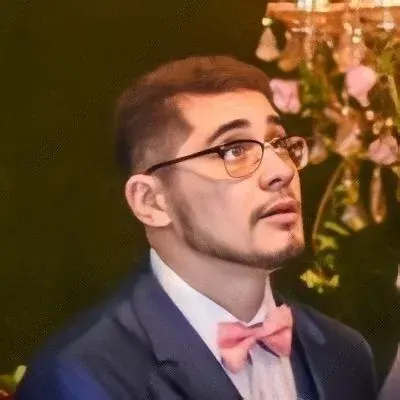
How to Make an AJAX Call without jQuery 💻📞
Are you tired of relying on jQuery for all your AJAX needs? Want to embrace the pure power of JavaScript? Look no further! In this blog post, we'll explore how you can make an AJAX call using vanilla JavaScript. No jQuery required! Let's dive in! 🌊
The Common Challenge 🎯
A common challenge faced by developers is making AJAX calls without relying on the jQuery library. While jQuery offers a convenient and easy-to-use AJAX API, some projects may prefer to avoid the additional library dependency or seek a more lightweight solution.
The Pure Power of JavaScript 🚀
Thankfully, JavaScript provides its own way to make AJAX requests using the XMLHttpRequest
object. This native object is supported in all modern browsers and allows you to interact with remote servers and retrieve data without any external libraries.
The Solution 🌟
Here's a simple example of how you can make an AJAX call using vanilla JavaScript:
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://api.example.com/data', true);
xhr.onload = function () {
if (xhr.status >= 200 && xhr.status < 400) {
var data = JSON.parse(xhr.responseText);
// Handle the retrieved data
}
};
xhr.send();
In this example, we create a new XMLHttpRequest
object and configure it with the desired HTTP method and URL. We also define an onload
event handler to handle the response from the server.
Once the request is configured, we call the send()
method to initiate the AJAX call. Upon successful retrieval of data (HTTP status code between 200 and 399), we can process the response using JSON.parse()
or perform any other required actions.
Engage with the Community! 🙌
Now it's your turn! Try making AJAX calls without jQuery in your next JavaScript project. Embrace the power of pure JavaScript and unleash your creativity! If you face any challenges or have alternative approaches to share, leave a comment below and let's discuss. Together, we can explore the endless possibilities of modern web development! ✨
So, what are you waiting for? Go ahead, make that AJAX call, and let's revolutionize the way we interact with remote servers! 💪
Happy coding! 🎉🔥