How can I loop through enum values for display in radio buttons?
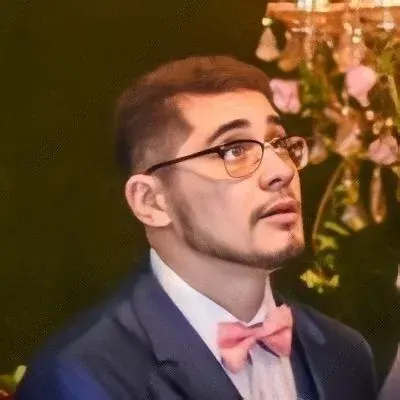
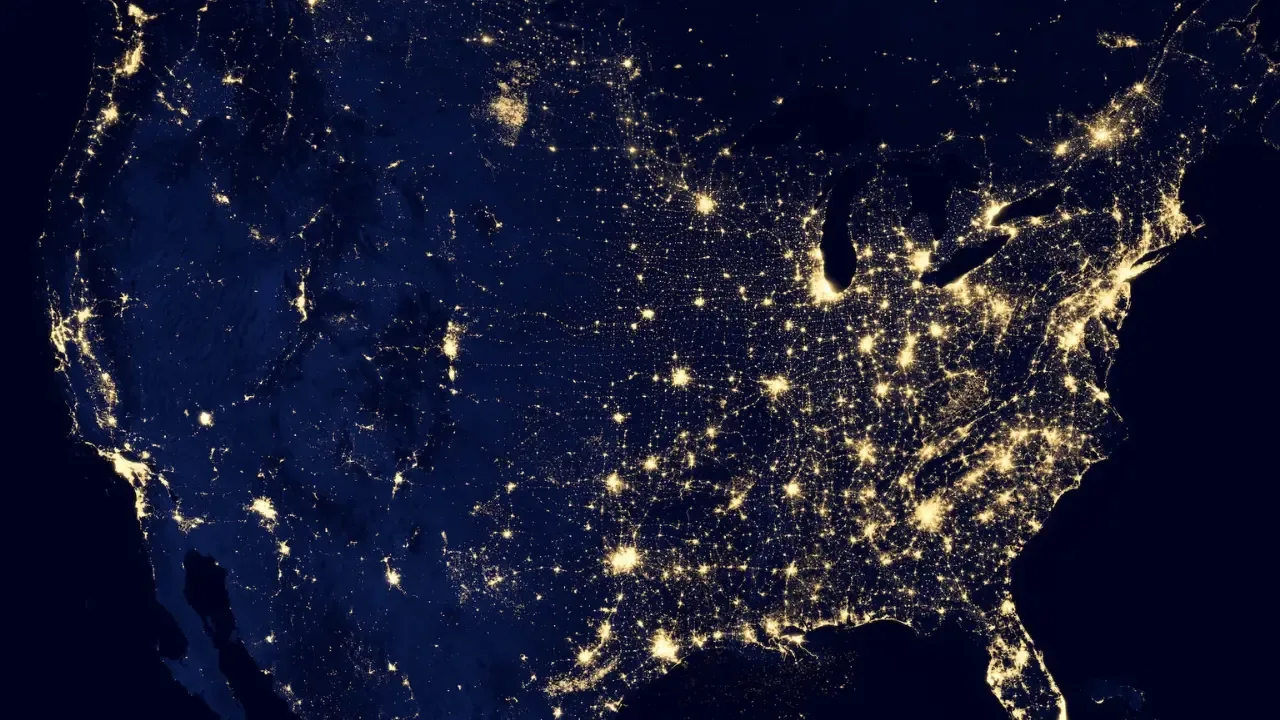
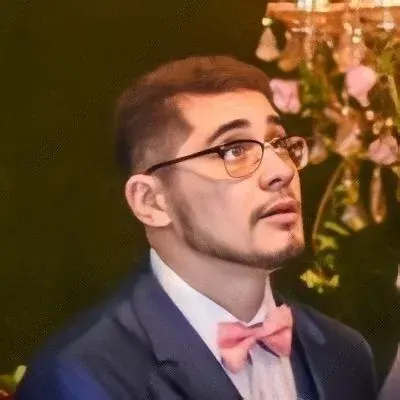
Looping Through Enum Values for Display in Radio Buttons
Have you ever found yourself needing to loop through the values of an enum to display them as options in a set of radio buttons? 🔄💡
If you're using TypeScript, you might have come across this issue before. But fear not, because in this blog post, we'll explore the common problems you may encounter and provide you with easy solutions. Let's dive in! 🤓👍
The Problem
Let's start by understanding the problem at hand. You have an enum called MotifIntervention
, which has four elements: Intrusion
, Identification
, AbsenceTest
, and Autre
. Your goal is to loop through these enum values and display them as options in a set of radio buttons.
However, when you implement a loop using TypeScript, you get unexpected results. Instead of getting the enum values, you see index numbers and the enum values combined. 🤔
The Solution
Here's how you can properly loop through the enum values in TypeScript and neatly display them as radio button options. 🎉
export enum MotifIntervention {
Intrusion,
Identification,
AbsenceTest,
Autre
}
export class InterventionDetails implements OnInit {
constructor(private interService: InterventionService) {
for (let motif in MotifIntervention) {
if (isNaN(Number(motif))) {
console.log(motif);
}
}
}
}
In the solution above, we use the for...in
loop to iterate over the properties of the enum. However, we add an additional check before printing the value to the console. By converting motif
to a number using Number(motif)
and checking if it is NaN
(Not a Number), we can filter out the index numbers and only display the enum values.
Putting It All Together
Now that you have the solution, you can implement it in your own code and see the desired results. Instead of getting index numbers, you'll only see the four enum values as expected. 🙌
However, keep in mind that this solution is specific to TypeScript. If you're using a different programming language, the approach may vary. Make sure to adapt the solution accordingly.
Take It Further
Are you excited to put this newfound knowledge to use? Great! Here's a challenge for you: take the solution we provided and implement it within your own project. Once you do, share your success story or any roadblocks you encountered in the comments below. Let's help each other learn and grow! 💪📈
Remember, understanding how to loop through enum values for display in radio buttons is a valuable skill to have in your developer toolkit. With this knowledge, you'll be able to create more dynamic and interactive user interfaces. So go ahead, give it a try, and happy coding! 😊💻
Have you ever struggled with looping through enum values before? Let me know in the comments below! Let's discuss and find solutions together. 💬👇