How can I guarantee that my enums definition doesn"t change in JavaScript?
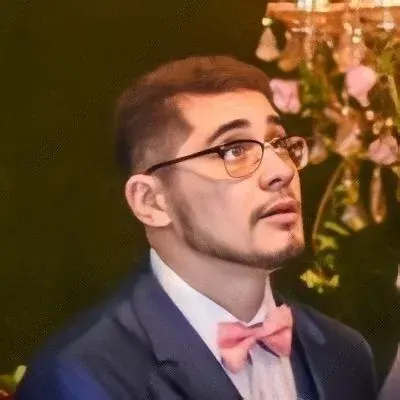
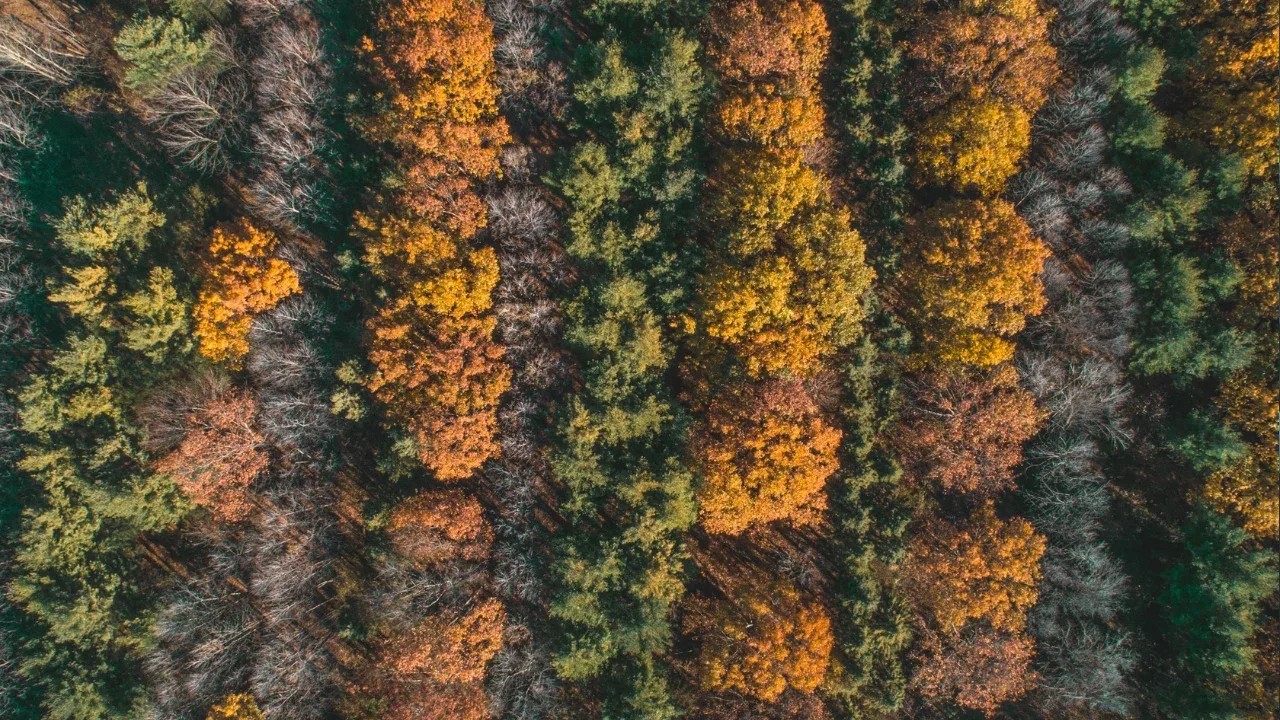
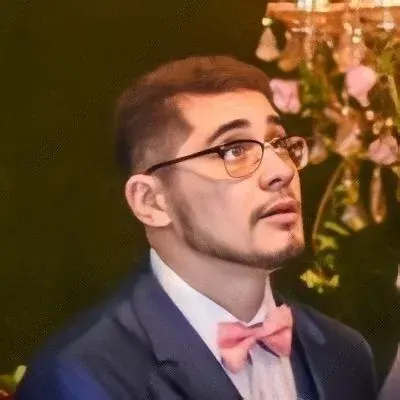
Title: 🌈 How to Create Immutable Enums in JavaScript 🚀
Have you ever found yourself needing to use enums in JavaScript, only to realize that they are not built-in like in some other programming languages? 😕 Fear not, because in this blog post, we will dive into the world of enums in JavaScript and discover how to create an immutable enum definition that won't change! 💪
The Scenario: 🤔
Let's imagine you are working on a project and you need to define a set of colors. You want to use enums to ensure type safety and improve code readability. 🎨 However, you are unsure of how to guarantee that your enum definition won't be modified accidentally.
The Challenge: 🧩
The code snippet you provided seems like a viable approach to defining an enum in JavaScript. By using an object with keys representing enum values, you can easily reference them in your code. 👍 However, this approach has one shortcoming: the object itself can be modified, which violates the immutability principle of enums. 😱
The Solution: 🌟
To create an immutable enum definition in JavaScript, we can use the Object.freeze()
method. This method prevents changes to an object's properties and ensures that our enums stay constant. Let's see how it works:
const ColorEnum = Object.freeze({
RED: 0,
GREEN: 1,
BLUE: 2
});
// Later on...
if (currentColor === ColorEnum.RED) {
// Do something awesome with red!
}
By using Object.freeze()
, we effectively lock down the ColorEnum
object, making it read-only. This way, any attempts to change or modify the enum will result in an error. 🚫😎
Encapsulating Enums: 🛡️
If you want to take the immutability of your enum a step further, you can encapsulate it within a module or a closure to avoid external access. This provides an extra layer of protection and helps maintain the integrity of your enum. 🚧
const colorEnum = (() => {
const enumValues = Object.freeze({
RED: 0,
GREEN: 1,
BLUE: 2
});
return {
getColorEnum: () => enumValues
};
})();
// Usage
const ColorEnum = colorEnum.getColorEnum();
if (currentColor === ColorEnum.RED) {
// Do something amazing with red!
}
In this example, enumValues
is encapsulated within a closure. The getColorEnum()
method acts as a getter for the enum, allowing access to the frozen enumValues
object. This approach enhances security and keeps our enum definition intact. 😄🔐
Conclusion and Call-to-Action: 📝💥
Creating immutable enums in JavaScript doesn't have to be a headache. By leveraging Object.freeze()
and encapsulation, you can ensure that your enum definition remains intact and unchangeable. 🙌
So, next time you need to define enums in JavaScript, remember to use Object.freeze()
and encapsulation for an extra layer of protection.
If you found this blog post helpful, feel free to share it with your fellow developers. Also, let us know in the comments how you have leveraged enums in your JavaScript projects! 🗣️💬
Keep coding and stay inspired! ✨👩💻👨💻