How can I get the full object in Node.js"s console.log(), rather than "[Object]"?
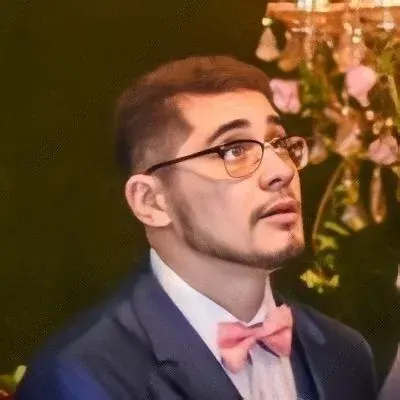
![Cover Image for How can I get the full object in Node.js"s console.log(), rather than "[Object]"?](https://images.ctfassets.net/4jrcdh2kutbq/376eUYzJAW7m4wV7PLqKoF/686c0d07d68f4a0bd05ecf1a1ec4bd01/Untitled_design__17_.webp?w=3840&q=75)
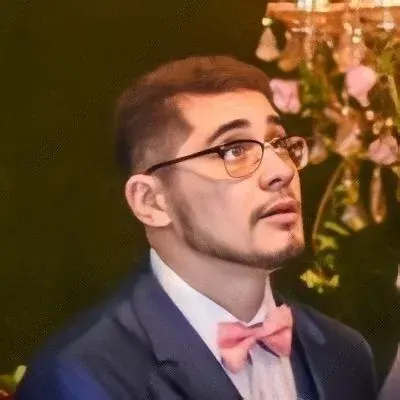
📝 Why is Node.js console.log() showing '[Object]' instead of the full object?
If you're working with complex objects in Node.js and using console.log()
to display their content, you may come across the frustrating situation where the output shows [Object]
instead of the actual data. This can be especially troublesome when you need to debug or understand the inner workings of the object.
🔍 The problem explained
The reason behind this behavior is that console.log()
in Node.js has a default depth limit when printing objects. This depth limit specifies how many levels deep the logging should go before truncating the output and replacing it with [Object]
. In the provided example, the object myObject
has a property f
that exceeds this depth limit, leading to the incomplete display.
In simpler terms, Node.js doesn't show the complete content of nested objects beyond a certain depth, resulting in a less informative output during debugging.
🛠️ Solving the issue
Thankfully, there are a couple of straightforward ways to overcome this limitation and get the full object displayed in the console.
1. Use the util.inspect() method
Node.js provides a utility module called util
, which includes a handy function called inspect()
. This function can be used to inspect objects and display them with a higher depth limit.
To achieve this, you need to require the util
module and modify your code as follows:
const util = require('util');
// ...
console.log(util.inspect(myObject, { depth: null }));
By passing { depth: null }
as the second argument to util.inspect()
, you instruct Node.js to display the complete object without any depth limitations. Running the above code will output the whole object, including the content of property f
.
2. Use JSON.stringify() for a quick fix
If you're looking for a quick fix without diving into the intricacies of the util
module, you can convert the object to a JSON string using JSON.stringify()
before logging it. While this method doesn't provide the same level of detail as util.inspect()
, it gives you a readable representation of the complete object.
Here's how you can apply this approach:
console.log(JSON.stringify(myObject, null, 2));
Running the above code will stringify the object and display it in a well-formatted JSON-like structure, including the entire content of property f
.
📣 Active engagement with readers
I hope this guide helped you understand why Node.js console.log() shows [Object]
and how to obtain the full object in your logs. Remember that debugging is an essential part of development, and having access to complete object information can greatly assist you in finding issues and building robust applications.
Now it's your turn! Have you encountered this problem before? What method do you prefer for displaying complex objects in the console? Share your thoughts, experiences, and additional tips in the comments below. Let's learn and grow together! 👇😄