How can I get query string values in JavaScript?
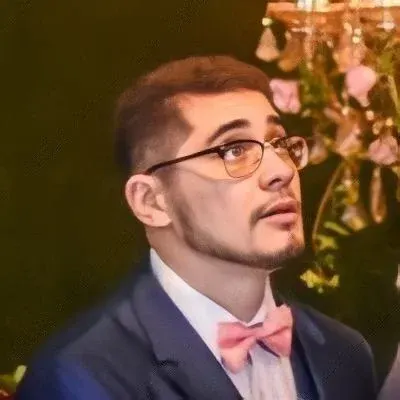
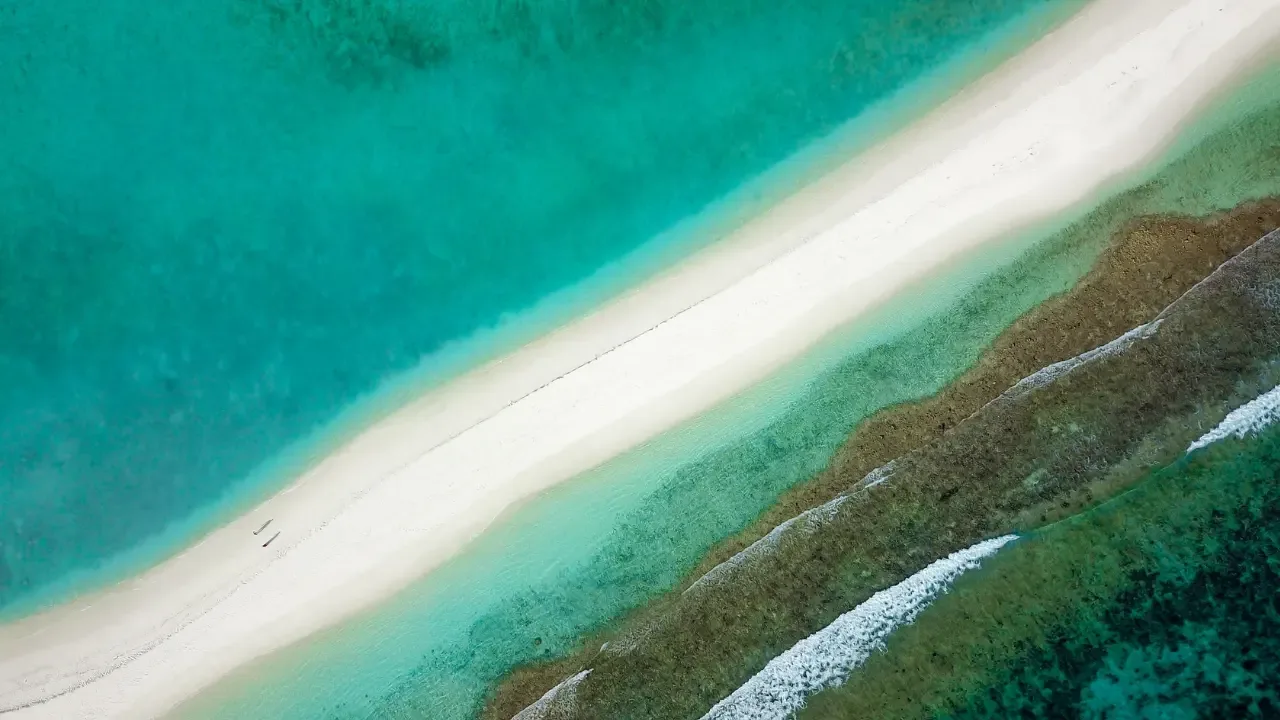
How to Get Query String Values in JavaScript: No Plugins Needed! 😎🔍
Query strings are parameters appended to the end of a URL, typically used for passing data between web pages. Retrieving and using these values in JavaScript can be a common issue for developers. But fear not! In this post, we'll explore a plugin-free way to effortlessly get query string values using JavaScript. 💪
The Problem: Getting Query String Values in JavaScript 💭❓
So, you have a URL with some query string parameters 😕:
https://example.com/page?name=John&age=25
And your mission is to extract the values of name
and age
programmatically. How can you achieve this without relying on any plugins (including jQuery)? 🤔
The Solution: JavaScript's Built-in URLSearchParams API 🚀🔍
JavaScript has a handy built-in feature called URLSearchParams
, which provides easy access to query string values. Let's dive into a step-by-step guide on how to use it effectively:
Step 1: Get the URL Search Params Object 📚
First, let's create a new instance of URLSearchParams
, passing the location.search
property as the argument:
const urlParams = new URLSearchParams(location.search);
Step 2: Retrieve Query String Values 💡
Once you have the urlParams
object, you can use various methods to extract the query string values. 🤓
Getting a Single Value:
To retrieve the value of a single query string parameter, use the get()
method:
const name = urlParams.get("name");
console.log(name); // Output: John
Getting Multiple Values:
If a query string parameter occurs multiple times, you can use the getAll()
method to get an array of all its values:
const hobbies = urlParams.getAll("hobby");
console.log(hobbies); // Output: ["reading", "coding", "hiking"]
Getting All Parameters:
To retrieve all query string parameters, use the keys()
and values()
methods:
for (const param of urlParams.keys()) {
console.log(param); // Output: name, age, hobby
}
for (const value of urlParams.values()) {
console.log(value); // Output: John, 25, ["reading", "coding", "hiking"]
}
Step 3: Handle Missing or Invalid Query Parameters ⚠️🔑
Sometimes, a query string parameter might be missing or invalid, potentially causing issues in your code. To handle such scenarios, you can employ conditional statements to provide fallback values or perform appropriate error handling. 🛠️
const name = urlParams.get("name") || "Anonymous";
Wrap Up and Take Action! 🎉🚀
You made it! Now you know a plugin-less way to effortlessly extract query string values using JavaScript's URLSearchParams
API. 🙌
Next time you encounter query string parameters in your web development journey, remember these steps:
Get the URLSearchParams object using
new URLSearchParams(location.search)
.Retrieve the values using
get()
,getAll()
,keys()
, andvalues()
methods.Handle missing or invalid parameters with conditional statements.
Feel free to try out this technique in your own projects and let us know how it goes! 😄🚀
👉 Remember to share this blog post with your fellow developers who might find this information useful. Sharing is caring! ❤️
✨ Happy coding! ✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
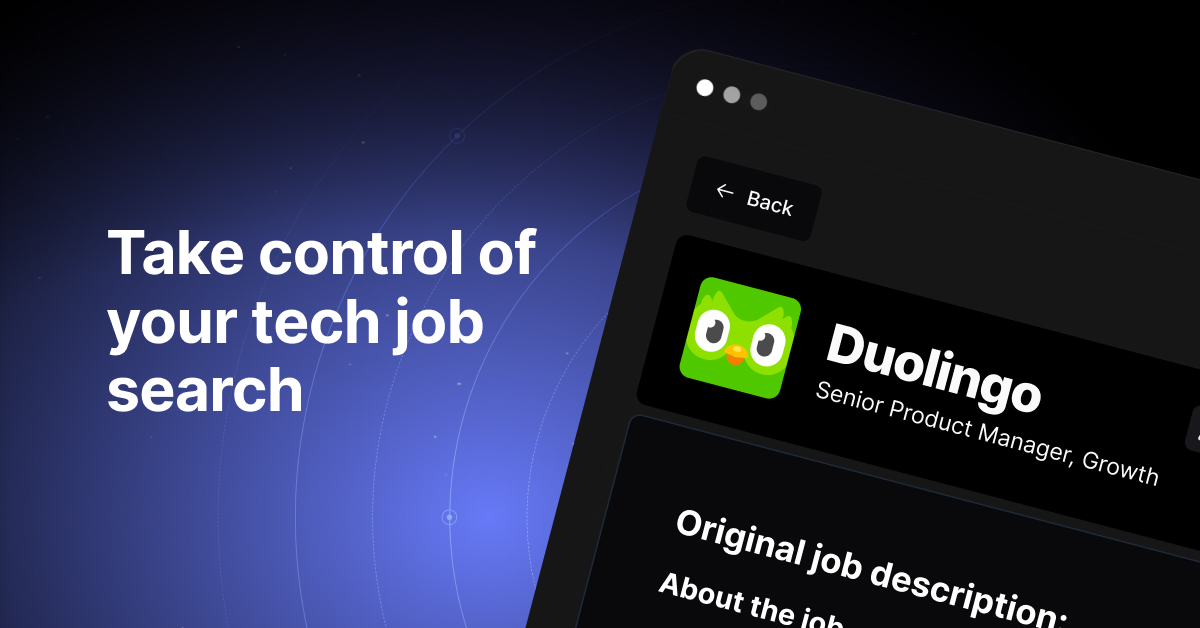