How can I get jQuery to perform a synchronous, rather than asynchronous, Ajax request?
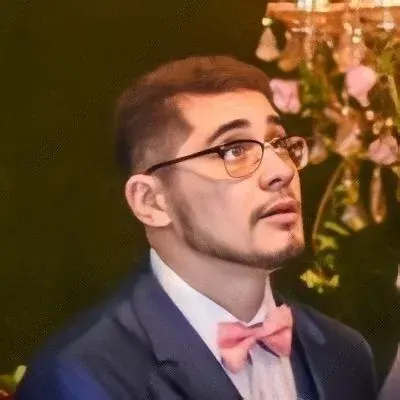
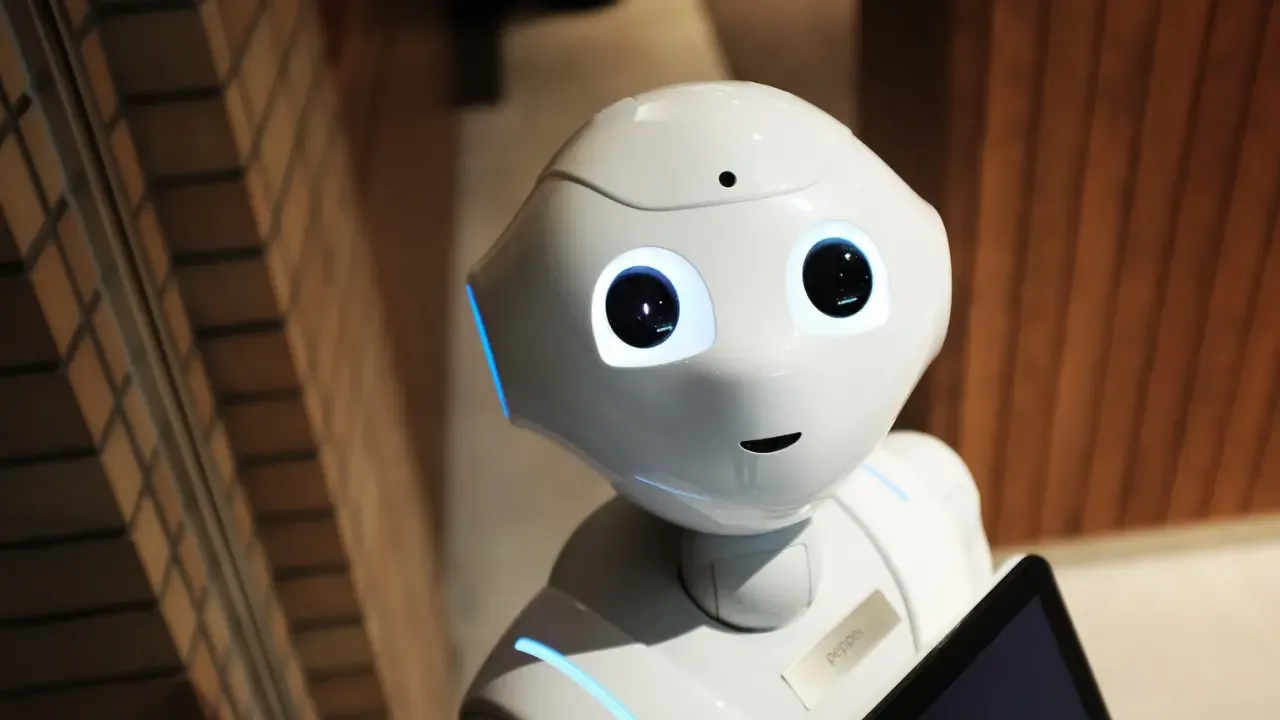
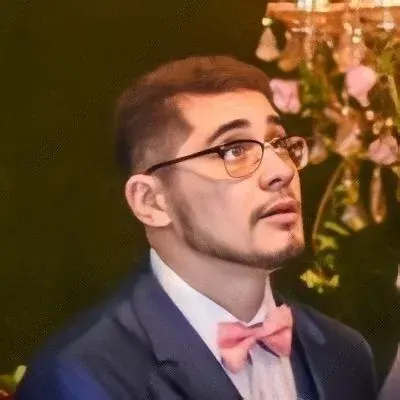
How to Make Synchronous Ajax Requests with jQuery 🔄🌐
Are you struggling to make your Ajax requests synchronous using jQuery? 🤔 Don't worry, we've got you covered! In this guide, we'll walk you through the common issues and easy solutions to get jQuery to perform synchronous Ajax requests. Let's dive in! 🏊♀️💥
The Problem Scenario 😩
Imagine you have a JavaScript widget with various extension points, and one of them is the beforecreate
function. This function is responsible for determining whether an item should be created or not.
You've decided to incorporate an Ajax call using jQuery within this beforecreate
function. However, you've encountered a roadblock. When the Ajax call is made asynchronously, the function returns before the response is received, making it difficult for you to prevent the item from being created. 😓
You find yourself in need of a solution to perform synchronous Ajax requests, which will allow you to control the flow and return false
in the mother function. So, how can you achieve this with jQuery or any other in-browser API? Let's find out! 🔍💡
The Solution 🚀
To make your Ajax requests synchronous, all you need to do is set the async
property to false
in your jQuery Ajax request. This tells jQuery to wait until the response is received before moving on to the next line of code. Here's how you can implement it:
beforecreate: function (node, targetNode, type, to) {
var result = jQuery.ajax({
url: 'http://example.com/catalog/create/' + targetNode.id,
data: { name: encode(to.inp[0].value) },
async: false
}).responseJSON;
if (result.isOk == false) {
alert(result.message);
return false;
}
}
By setting async: false
, the jQuery.ajax
function will block the execution of the subsequent lines of code until the response is received. This allows you to handle the response synchronously within your function, preventing the item from being created if necessary. 😎
Don't Forget the Caveats! ⚠️
While synchronous Ajax requests can be useful in certain situations, it's important to be aware of their potential drawbacks. Since synchronous requests halt the execution of code until the response is received, it may cause your application to appear unresponsive or freeze temporarily. ⏳❄️
It's generally recommended to use asynchronous requests whenever possible, as they provide a better user experience and improved performance. However, if synchronous requests are absolutely necessary for your specific use case, be sure to consider the potential impact on the overall user experience.
Your Turn to Take Action! ✅📢
Now that you know how to make synchronous Ajax requests with jQuery, it's time to put your newfound knowledge into action! 🙌 Try implementing this solution in your own code and see the magic happen.
If you encounter any issues or have questions along the way, feel free to leave a comment below. Our tech community is here to support you! 💪👩💻👨💻
And don't forget to share this blog post with your fellow developers who might find it useful! Sharing is caring, after all. 😉🚀
Happy coding! 💻💥