How can I get form data with JavaScript/jQuery?
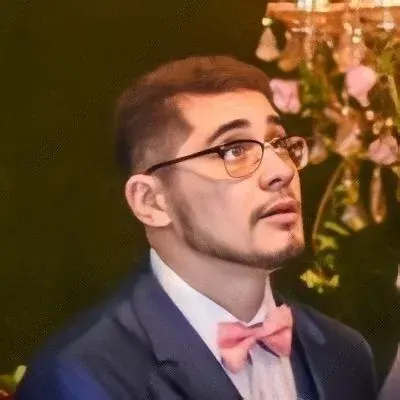
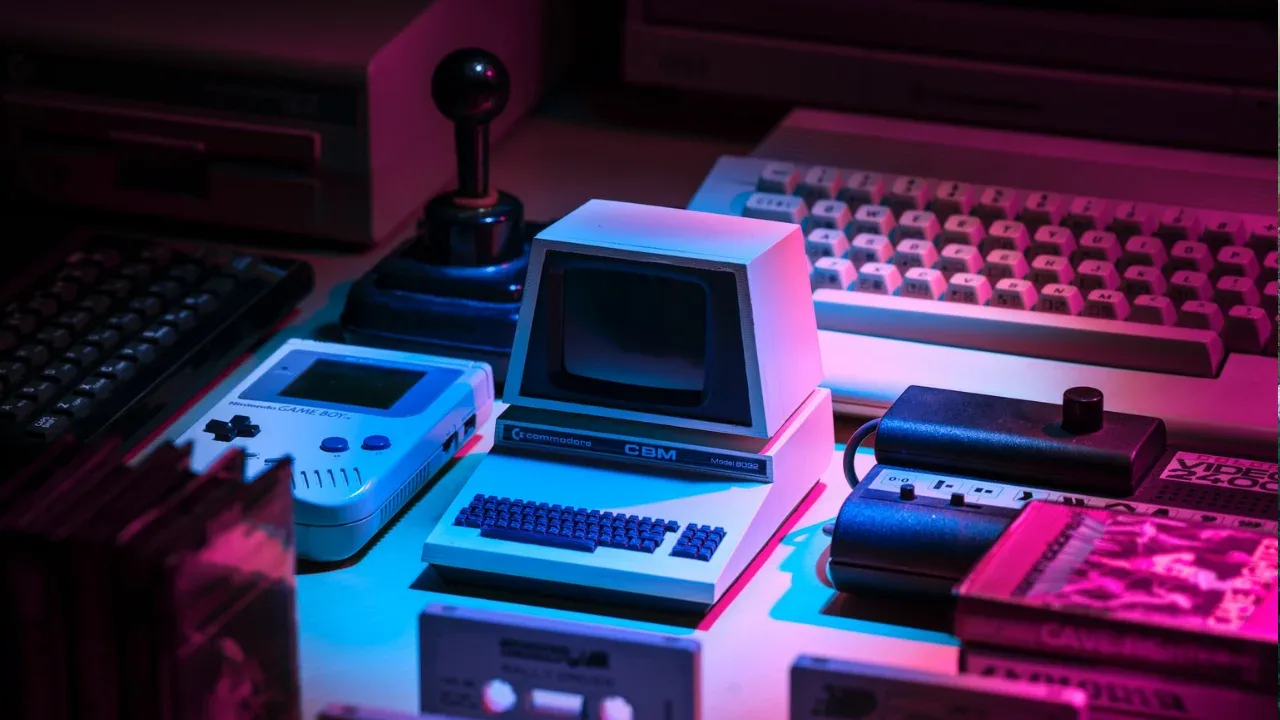
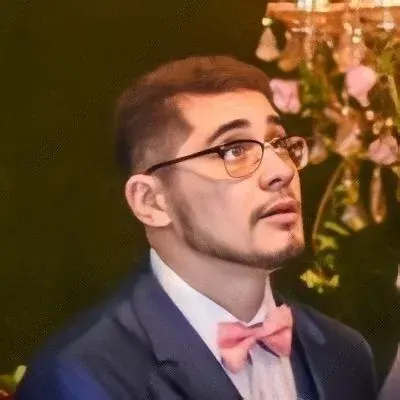
📝 Blog Post: How to Get Form Data with JavaScript/jQuery 🤔
Welcome, tech-savvy friends! 🌟 Today we're diving into the marvelous world of JavaScript/jQuery to address a common question: How can one fetch form data effortlessly? Whether you're an aspiring developer or a seasoned pro, this guide will equip you with the knowledge you need to conquer form data retrieval. Let's get started! 💪🔥
The Quest for Easy Form Data 🕵️♂️
Imagine you have a snazzy form on your web page, and you're itching to extract that crucial data without breaking a sweat 🌬️. You may ask, "Is there a sleek, one-line solution to acquire form data effortlessly, like the classic HTML-only way of submission?" 🤔 Good news, my friend! We've got you covered!
Here's an example of a form snippet to help us understand the desired output:
<form>
<input type="radio" name="foo" value="1" checked="checked" />
<input type="radio" name="foo" value="0" />
<input name="bar" value="xxx" />
<select name="this">
<option value="hi" selected="selected">Hi</option>
<option value="ho">Ho</option>
</select>
</form>
The expected output is:
{
"foo": "1",
"bar": "xxx",
"this": "hi"
}
Now, let's see why a seemingly simple solution might not cut it and explore a more robust alternative! 💡
Unraveling the Simplicity Fallacy 🕳️
One might attempt this simplistic solution using jQuery to iterate through the form inputs:
$("#form input").each(function () {
data[theFieldName] = theFieldValue;
});
While this approach is simple, it falls short in correctly including textareas, selects, radio buttons, and checkboxes. So, how can we solve this puzzle once and for all? 🤓
Cracking the Code! 🎉
Hold onto your seatbelts, folks! We have a high-powered algorithm that will reliably fetch form data without missing a beat! Here's our solution:
var formData = {};
$("form :input").each(function() {
var element = $(this);
var name = element.attr("name");
var value = element.val();
formData[name] = value;
});
console.log(formData);
In this code snippet, we start by initializing an empty formData
object. Using the powerful $
selector in jQuery, we target the :input
elements within the form
. This includes text inputs, textareas, select dropdowns, checkboxes, and radio buttons 💫.
We then iterate over each element, extracting its name
attribute and value
. Finally, we assign the name
and value
to the formData
object using the bracket notation.
🗝️ The Key Takeaways:
Utilize the
$
selector in jQuery to target:input
elements within theform
tag.Extract the
name
attribute andvalue
of each form element.Assign the
name
andvalue
to a JavaScript object for further processing or display.
And there you have it, my curious coder! With our elegant solution, you'll be capturing form data with ease 🎯.
Engage with Us! 💬
We'd love to hear your thoughts and experiences with fetching form data using JavaScript/jQuery!🙌
Have you encountered any challenges while grabbing form data on your projects?
Do you have alternative solutions that you'd like to share with our community?
Let's continue the conversation in the comments below! 👇
Keep exploring, keep coding! 🚀