How can I get a character array from a string?
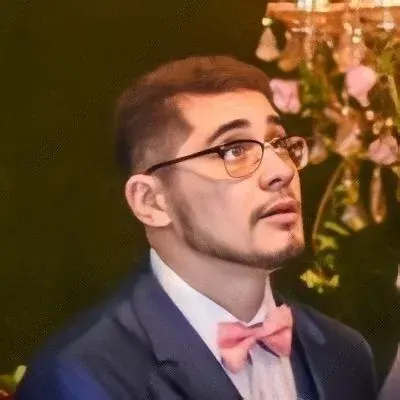
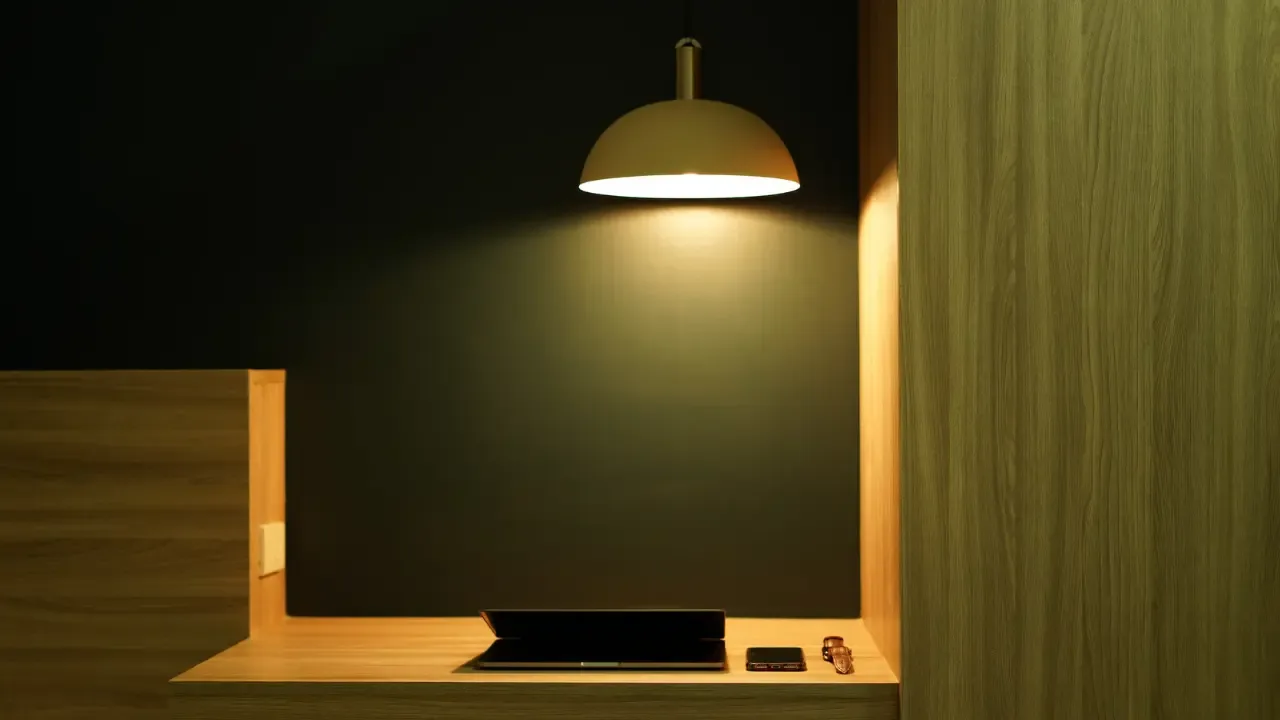
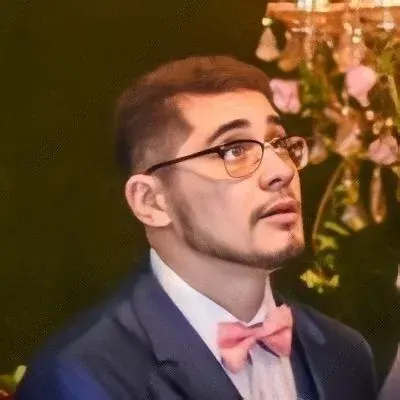
📚 Tech Savvy101 - Helping You Navigate the Tech World 🤖
The Easiest Way to Get a Character Array from a String in JavaScript! 🌟
Welcome back to Tech Savvy101, the blog where we make complex tech concepts easy to understand! Today, we'll address a common issue faced by many JavaScript developers: how to convert a string into a character array. 🤔
So, you want to transform a string like "Hello world!"
into an array ['H','e','l','l','o',' ','w','o','r','l','d','!']
? No worries, we've got you covered! Here are two simple solutions to tackle this problem:
Solution 1: Using the .split('')
method
One way to convert a string into a character array is by using the .split('')
method. This method splits a string into an array of substrings, using a specified character (in this case, an empty string ''
) as the separator. 🧩
Here's an example of how to use the .split('')
method to achieve the desired result:
const string = "Hello world!";
const charArray = string.split('');
console.log(charArray);
Voilà! 🎉 By calling the .split('')
method on your string, you'll get the desired character array as output. Easy, right? 😉
Solution 2: Using the Array.from()
method
Another way to achieve the same result is by using the Array.from()
method. This method creates a new array instance from an array-like or iterable object, which is perfect for converting a string into an array of characters. 🚀
Check out the following code snippet to see the Array.from()
method in action:
const string = "Hello world!";
const charArray = Array.from(string);
console.log(charArray);
Amazing! 🙌 With just one line of code, you can create your character array using the Array.from()
method. Isn't JavaScript awesome? 😎
Now that you have these easy solutions at your fingertips, go ahead and give them a try in your own code! 🛠️
✨ Call-to-action: Share Your Thoughts!
How do you usually convert a string into a character array? Do you have any other unique methods or tips to share? We'd love to hear from you! Leave a comment below and join the discussion. Let's learn from each other and make our coding journeys more enjoyable! 😄
That's all for today, folks! We hope you found this guide helpful and now feel confident in getting a character array from a string in JavaScript. Stay tuned for more useful tech tips and tricks on Tech Savvy101. Until next time! 💡
🖥️ Follow us on Twitter 📩 Subscribe to our newsletter for weekly tech updates!