How can I extract a number from a string in JavaScript?
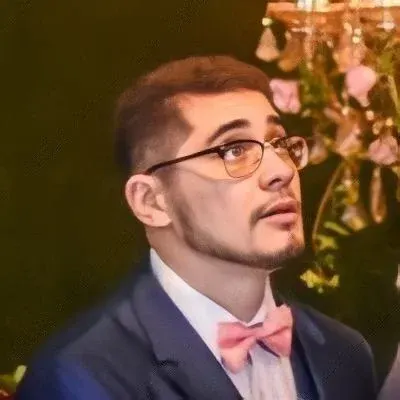
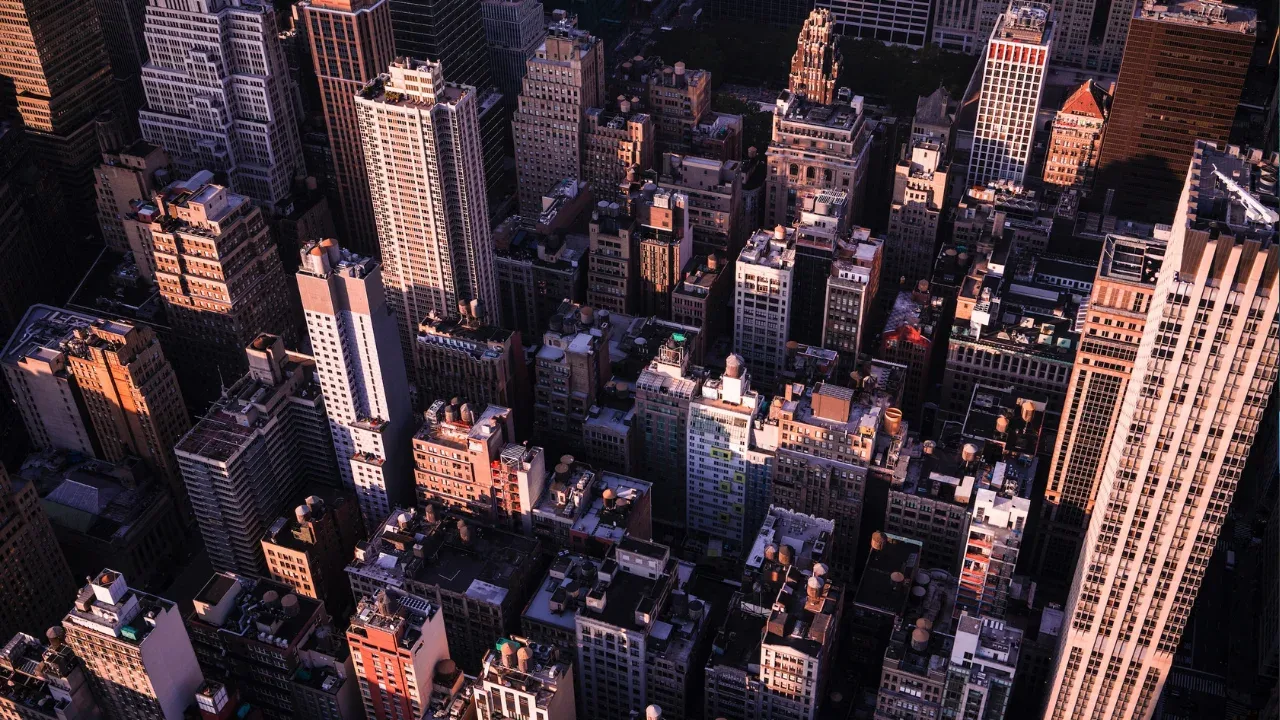
📝 Extracting a Number from a String in JavaScript: A Simple Guide
Hey there, fellow JavaScript enthusiast! 😄
Are you struggling with extracting a number from a string in JavaScript? Don't worry, you're not alone! Many developers have encountered this common issue and found it quite puzzling. But fear not, because today, I'm going to guide you through some easy solutions to help you extract that coveted number from your strings!
🧩 The Problem
Let's take a look at the context that brought about this question. Our friend has a string in JavaScript, like #box2
, and they desire to extract the number 2
from it. They started off their journey by attempting the following code:
var thestring = $(this).attr('href');
var thenum = thestring.replace(/(^.+)(\w\d+\w)(.+$)/i, '$2');
alert(thenum);
However, the result was not as expected. The alert still displayed #box2
, instead of just the number 2
. Our fellow developer specifically mentioned the need for the code to work with any length number attached at the end.
Let's dive into some easy solutions to this problem! 💪
🔍 Solution #1: Using Regular Expressions
The code attempted by our friend does utilize a regular expression. However, there is a small tweak we can make to achieve the desired result. Instead of capturing the entire string, including the hashtag and the number, we can simply capture the number itself.
var thestring = $(this).attr('href');
var thenum = thestring.match(/\d+/);
alert(thenum[0]);
By using the match()
function and the regular expression \d+
, we can find and extract all the numbers in the string. Since the match()
function returns an array, we can access the extracted number using the index [0]
.
🧰 Solution #2: Utilizing JavaScript String Methods
Another approach to extracting numbers from a string is by utilizing JavaScript's built-in String methods. Here's a simple solution using the replace()
and parseInt()
functions:
var thestring = $(this).attr('href');
var thenum = parseInt(thestring.replace(/\D/g, ''));
alert(thenum);
In this solution, the replace()
function with the regular expression \D/g
removes all non-digit characters from the string. Then, the parseInt()
function converts the resulting string into an actual number.
⚡️ Engage and Share Your Thoughts!
I hope these two solutions have helped you overcome the challenge of extracting a number from a string in JavaScript! Remember, there's often more than one way to tackle a problem in programming, so feel free to explore and experiment with different approaches.
If you have any questions, suggestions, or an alternative solution, I'd love to hear about it! Share your thoughts in the comments section below and let's engage in a meaningful discussion. 😊🎉
Happy coding! 🚀
Note: This blog post was inspired by a question on a coding forum. If you have a tech-related question or topic you'd like me to cover, feel free to drop it in the comments or message me! Remember, learning is a two-way street.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
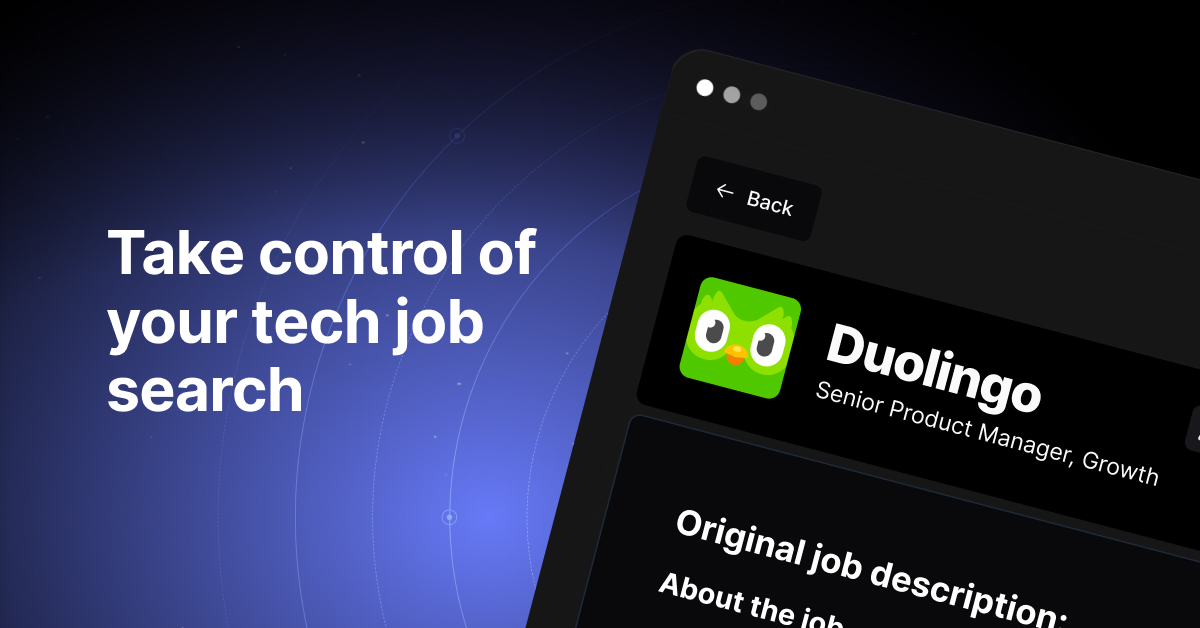