How can I export Excel files using JavaScript?
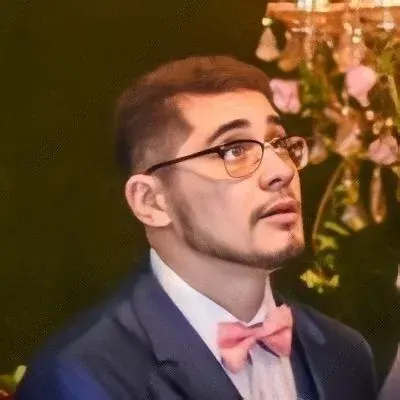
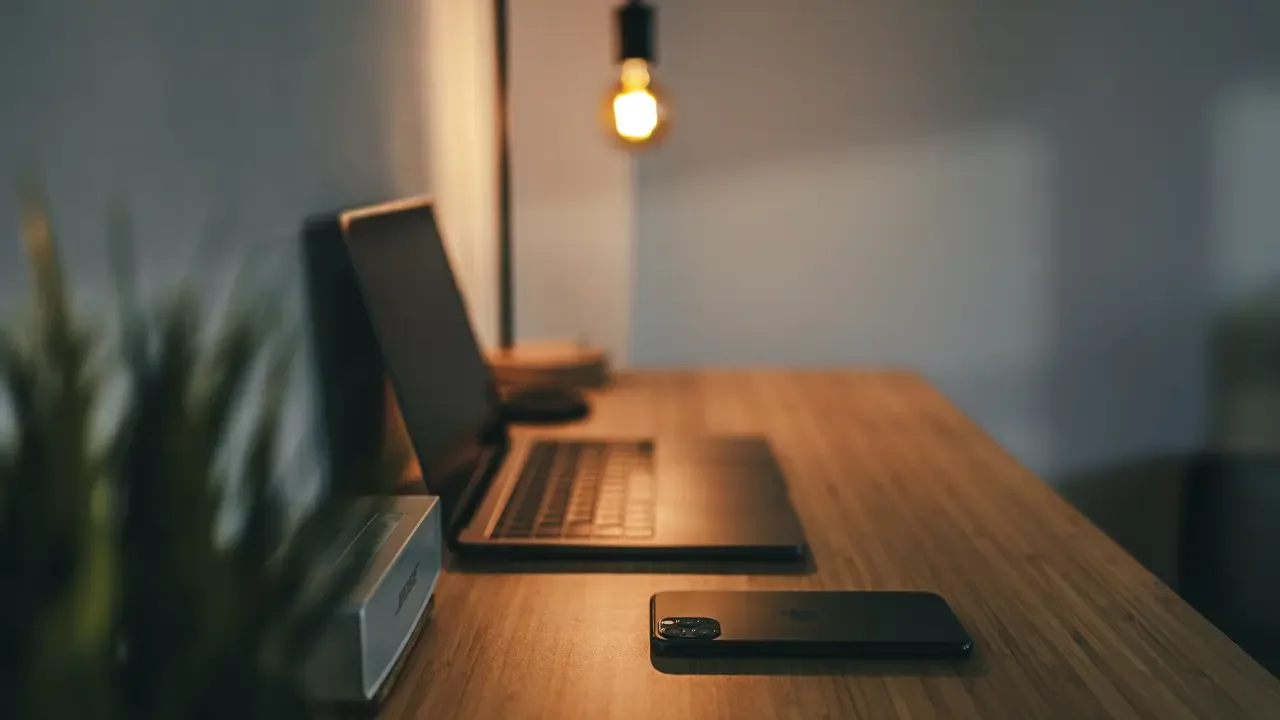
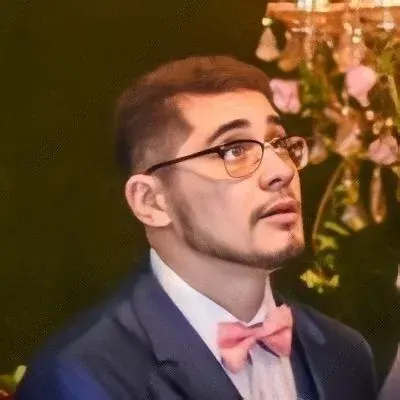
Exporting Excel Files Using JavaScript: A Simple Guide
Are you tired of manually exporting CSV or Excel files from your web application? 🤔 Do you wish there was an easier way to generate these files using JavaScript? Well, you're in luck! In this blog post, we'll explore various methods to export Excel files using JavaScript.
The Challenge: Generating Excel/CSV Files via JavaScript
One common requirement in web development is the ability to generate Excel or CSV files dynamically. This could be useful when presenting data from a database or creating reports. Luckily, there are several approaches you can take to achieve this. Let's explore some of them. 💪
Method 1: Using a Library
One of the easiest ways to export Excel files using JavaScript is by utilizing a library. These libraries provide ready-to-use functions and components, saving you time and effort. Here are a few popular options:
1. SheetJS
SheetJS is a powerful JavaScript library that allows you to read, write, and manipulate Excel files. It supports both client-side and server-side environments and provides extensive functionality for exporting data to various file formats, including XLSX and CSV.
To use SheetJS, you'll need to include the library in your project and utilize its APIs to generate the desired Excel file. Check out the official documentation for code examples and detailed instructions.
2. Handsontable
Handsontable is another fantastic library for creating interactive Excel-like tables in JavaScript. It provides a rich set of features, including the ability to export data to Excel or CSV files. Handsontable supports both client-side and server-side rendering and offers straightforward APIs to facilitate the exporting process.
To get started with Handsontable, head over to their website and explore their documentation. You'll find clear examples and guidance on how to export your data effortlessly.
Method 2: Pure JavaScript Approach
If you prefer to avoid using external libraries, there's still a way to export Excel files using pure JavaScript. Although it requires some manual work, this approach provides full control over the export process.
Generate the Excel/CSV content using JavaScript. You can construct the content as a string or an array of values.
Create an
a
element in the DOM and set itshref
attribute to adata:application/octet-stream
URL. This URL will contain the generated Excel/CSV content.Set the
download
attribute of thea
element to specify the file name for the downloaded file.Use the
.click()
method on thea
element to trigger the file download.
Here's an example code snippet demonstrating this approach:
const content = "Name, Age, Email\nJohn Doe, 25, john@example.com\nJane Smith, 30, jane@example.com";
const fileName = "data.csv";
const link = document.createElement("a");
link.href = "data:application/octet-stream," + encodeURIComponent(content);
link.download = fileName;
link.click();
This code will generate a CSV file with the specified content and initiate its download upon execution. You can customize the content
and fileName
variables according to your requirements.
📢 Call to Action: Share Your Experience!
Now that you know how to export Excel files using JavaScript, it's time to put this knowledge into practice. Try out the different methods we discussed and find the one that suits your needs the best. Don't forget to share your experience and let us know which approach worked for you!
If you have any questions or face any challenges during the implementation, feel free to leave a comment below. We'd be more than happy to assist you.
Happy coding! 💻🎉