How can I do string interpolation in JavaScript?
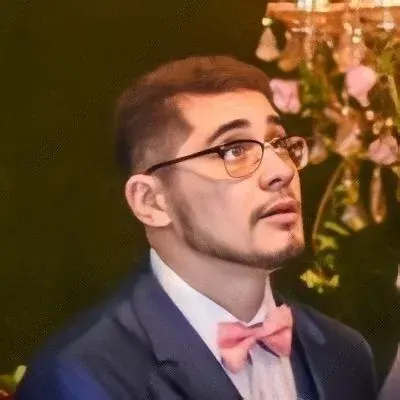
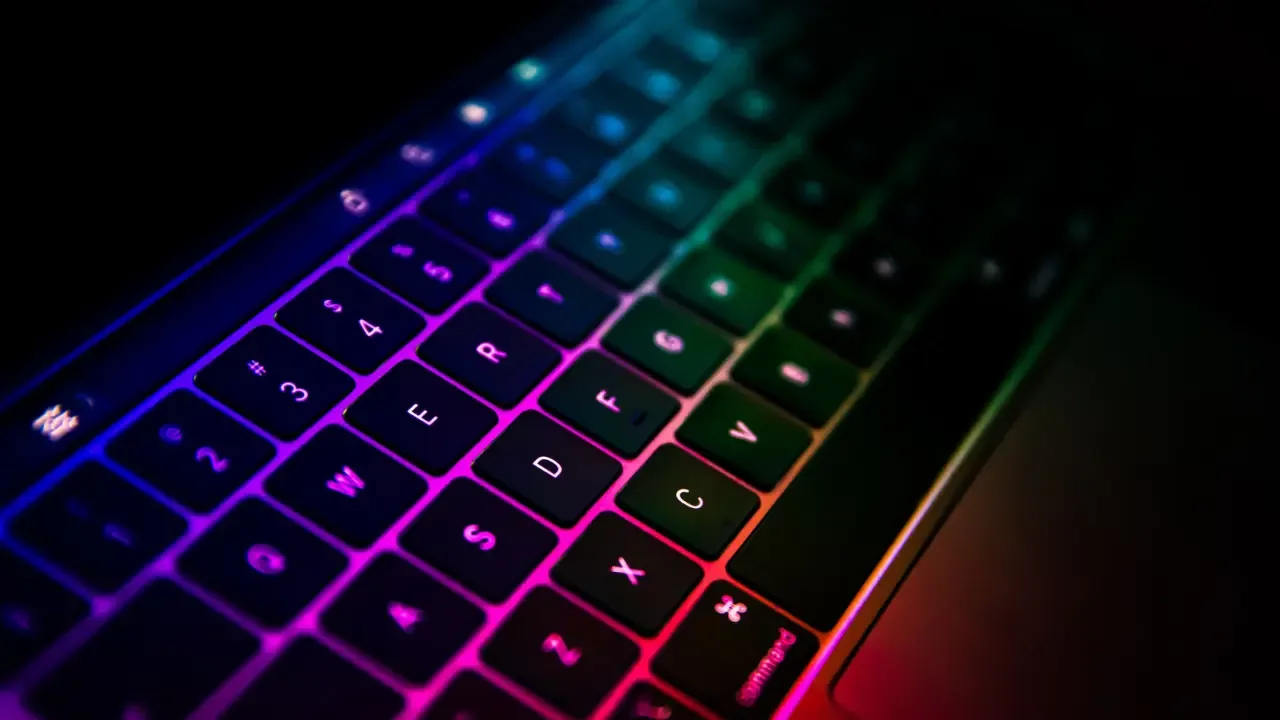
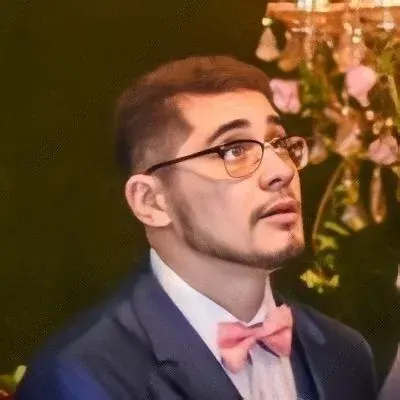
Easy String Interpolation in JavaScript: Injecting Variables like a Pro! 💥
Are you tired of the hassle of string concatenation in JavaScript? Do you wish there was an easier way to inject variables into your strings? Well, get ready to level up your coding game because we're about to dive into the world of string interpolation! 🚀
The Problem with String Concatenation
Let's take a look at the following code snippet:
var age = 3;
console.log("I'm " + age + " years old!");
In this example, we use the +
operator to concatenate the variable age
with the surrounding string. While this approach works, it can quickly become messy and error-prone, especially when dealing with complex strings or multiple variables.
Introducing String Interpolation
Fortunately, JavaScript offers a cleaner and more elegant solution called string interpolation. With string interpolation, you can directly embed variables into your strings without the need for concatenation. 🎉
Using Template Literals for String Interpolation
JavaScript introduced template literals as part of ECMAScript 2015 (ES6). Template literals are delimited by backticks (`) instead of single or double quotes.
Here's how you can rewrite our previous example using template literals:
var age = 3;
console.log(`I'm ${age} years old!`);
By enclosing the variable age
within ${}
within the template literal, JavaScript automatically evaluates the expression and injects the value into the string.
🎯 Pro Tip:
You can even perform computations or call functions within the ${}
expression! Let's say you want to display someone's age in dog years. Here's how you can achieve it:
var age = 3;
console.log(`I'm ${age * 7} dog years old!`);
Browser Support for Template Literals
Before going all-in with template literals, it's essential to verify if the browsers you are targeting support this feature. Most modern browsers, including Chrome, Firefox, Safari, and Edge, have excellent support for template literals. However, if you need to support older browsers like Internet Explorer, you may need to transpile your code using a tool like Babel to ensure compatibility.
Level Up Your String Interpolation Game!
Now that you know how to perform string interpolation using template literals, you can simplify your code and improve its readability. Say goodbye to tangled and convoluted strings and embrace the simplicity and power of string interpolation.
So, what are you waiting for? Start leveraging the magic of template literals today and code like a JavaScript ninja with ease! 💪✨
Don't forget to share your thoughts and experiences with string interpolation in the comments below. Have you encountered any challenges or found creative ways to use it in your projects? Let's start a conversation and learn from each other! 🤝🔥