How can I display a JavaScript object?
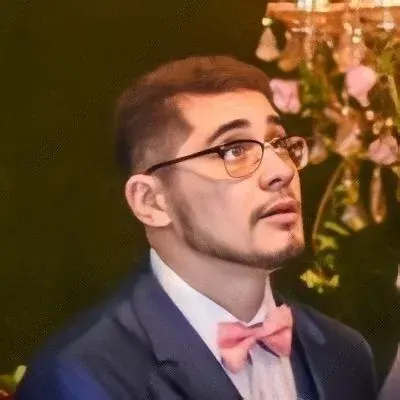
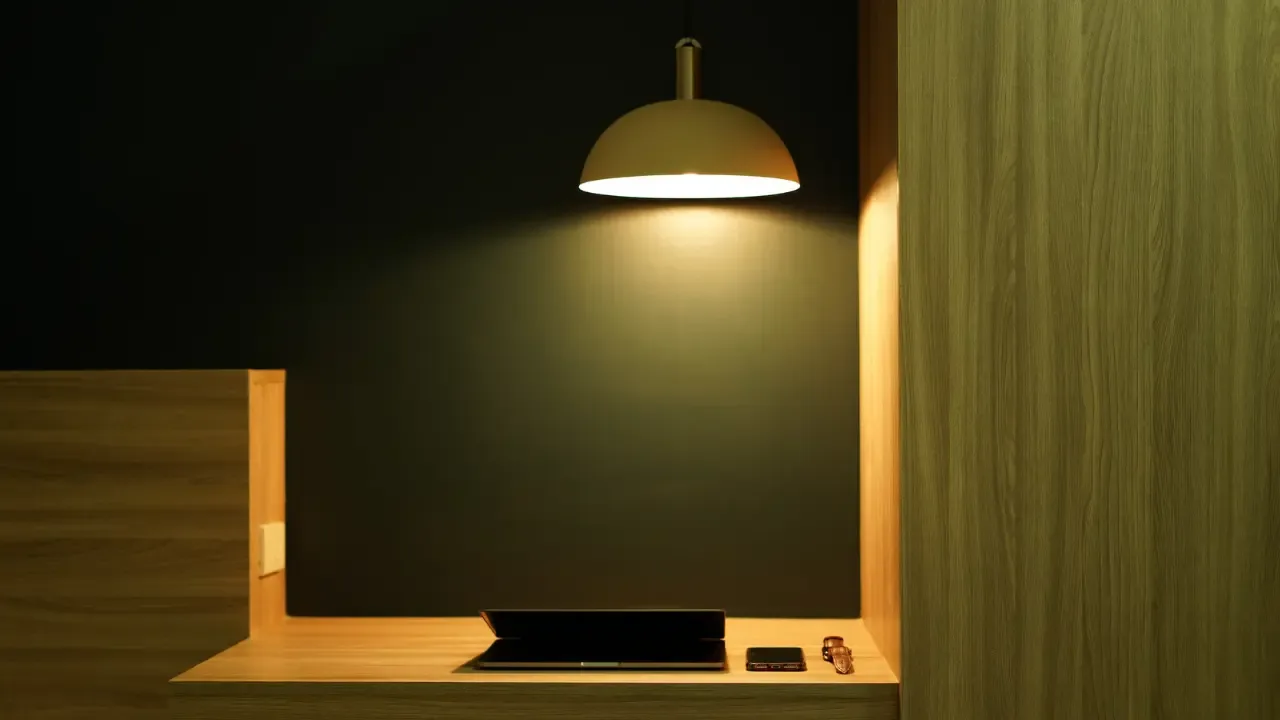
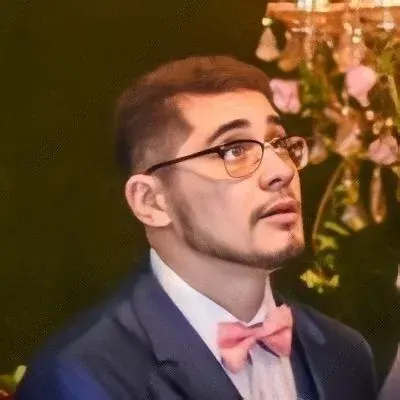
Show me what you got: Displaying JavaScript Objects the Cool Way! 😎
So, you want to display a JavaScript object in a string format, just like when we use the trusty alert
function to display a variable? Well, my friend, you've come to the right place! 😄
The Problem: How do I display a JavaScript object?
You may have found yourself scratching your head, wondering how to display the content of a JavaScript object in a neat string format. You've tried using alert
, but it only works for simple variables, not complex objects. Fear not, because we've got some super cool solutions for you! 🚀
Solution #1: JSON.stringify() to the Rescue
The first solution to your problem is the mighty JSON.stringify()
function. It's like a superhero that transforms your object into a JSON string representation. Let's take a look at an example:
const myObj = { name: "John", age: 25, city: "New York" };
const jsonString = JSON.stringify(myObj);
console.log(jsonString); // Outputs: {"name":"John","age":25,"city":"New York"}
Voila! 🎉 Now you can display your JavaScript object as a string! Just remember that JSON.stringify()
has some limitations when it comes to handling circular references or functions.
Solution #2: Custom Object-to-String Conversion
If you want more control over how your JavaScript object is displayed as a string, you can define a custom conversion method. This method is called toString()
and it allows you to define the string representation of the object. Here's an example:
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.toString = function() {
return `Person: ${this.name} (Age: ${this.age})`;
};
const john = new Person("John", 25);
console.log(john.toString()); // Outputs: "Person: John (Age: 25)"
Boom! 💥 You just added some custom swag to your object display!
Call-to-Action: Join the Object Display Revolution!
Now that you've learned some kick-ass ways to display JavaScript objects, it's time to take action! Go ahead and try these solutions in your own code. Experiment, customize, and show off your object display skills! And don't forget to share your awesome results with us in the comments below! 🔥
So what are you waiting for? Start displaying those objects like a pro and let the code world be amazed! Happy coding! 😄✨