How can I detect pressing Enter on the keyboard using jQuery?
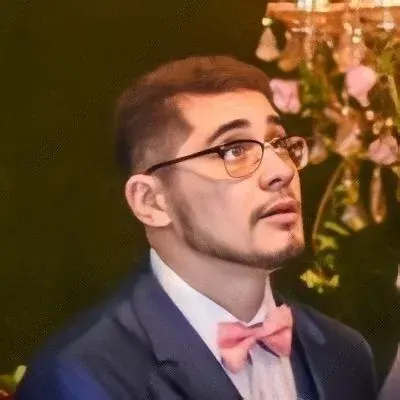
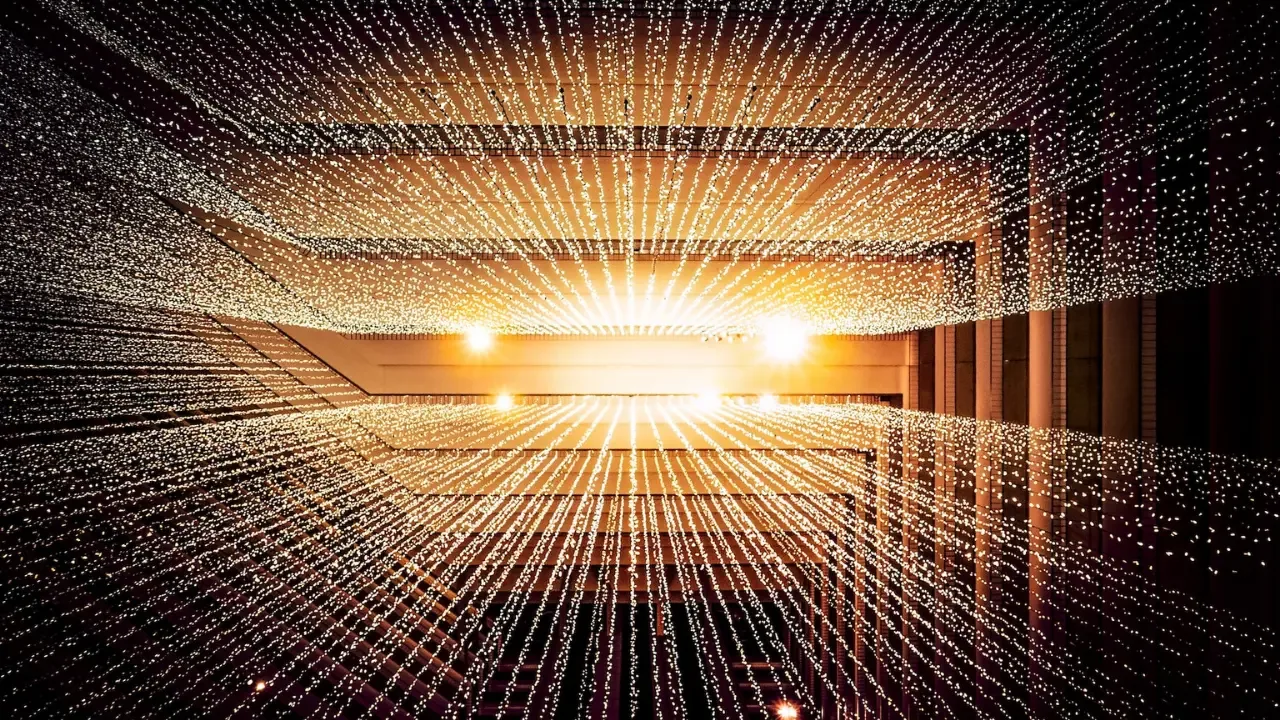
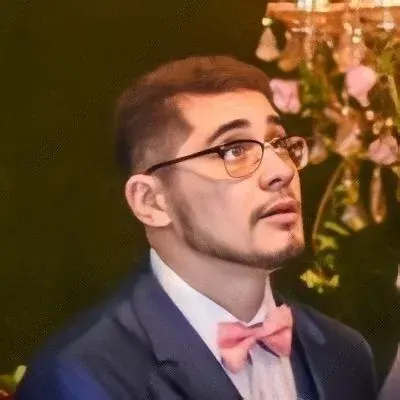
πHey there! So you want to detect when the user presses the mighty Enter key on their keyboard using everyone's favorite JavaScript library, jQuery! No worries, I got you covered! π©πͺ
First of all, let me assure you that you don't need any fancy plugins for this task. jQuery has your back with its built-in method called keypress()
. You can use this method to detect when any key is pressed, including the Enter key. ποΈπ
To start, let's take a closer look at the syntax of the keypress()
method. It takes a callback function where you can place your code to be executed when a key is pressed. Let's see an example:
$(document).keypress(function(event) {
if (event.which === 13) {
// Perform your desired action here
}
});
In this code snippet, we're attaching the keypress()
method to the document
object. Inside the callback function, we're checking whether the pressed key has a key code of 13. If it does, it means the Enter key was pressed! π
Now, I hear you asking, "But what about browser compatibility? Can I rely on this method?" Well, my friend, I'm glad you brought that up! ππ€
The keypress()
method works well in most modern browsers. However, there's a slight difference in behavior between different browsers when it comes to detecting special keys like Enter. Some browsers treat keypress events differently, while others use different event types altogether. But don't worry, we have a solution! πβ¨
To ensure maximum compatibility, you can use the keyup()
method in addition to keypress()
. When the Enter key is pressed, both methods will fire events, allowing you to cover all your bases. Let's see it in action:
$(document).on('keypress keyup', function(event) {
if (event.type === 'keypress' && event.which === 13 ||
event.type === 'keyup' && event.keyCode === 13) {
// Perform your desired action here
}
});
In this example, we're using the on()
method to attach both the keypress
and keyup
events to the document
object. We then check which event was fired and match the key code appropriately.
π‘ Pro Tip: If you want to detect Enter key presses on a specific input element, just replace $(document)
with the selector for that element, like $('#my-input')
.
Okay, now it's your turn to give it a try! Implement the code examples I provided, and you'll be well on your way to detecting Enter key presses like a boss! πβ¨οΈ
Once you've mastered this technique, the possibilities are endless. You can use it to submit forms, trigger searches, or create cool interactive experiences! So go ahead, experiment, and have fun with it!
Don't forget to share your experiences or ask any more tech-related questions in the comments below. Let's code together and make the web a better place! π»π
Happy coding! π©βπ»π₯