How can I detect changes in location hash?
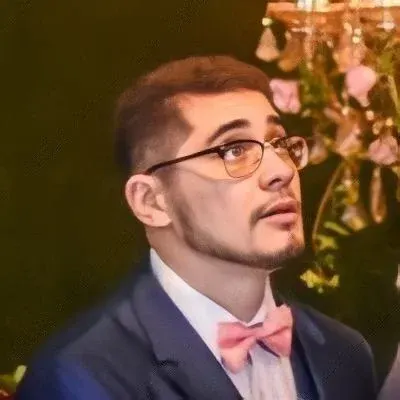
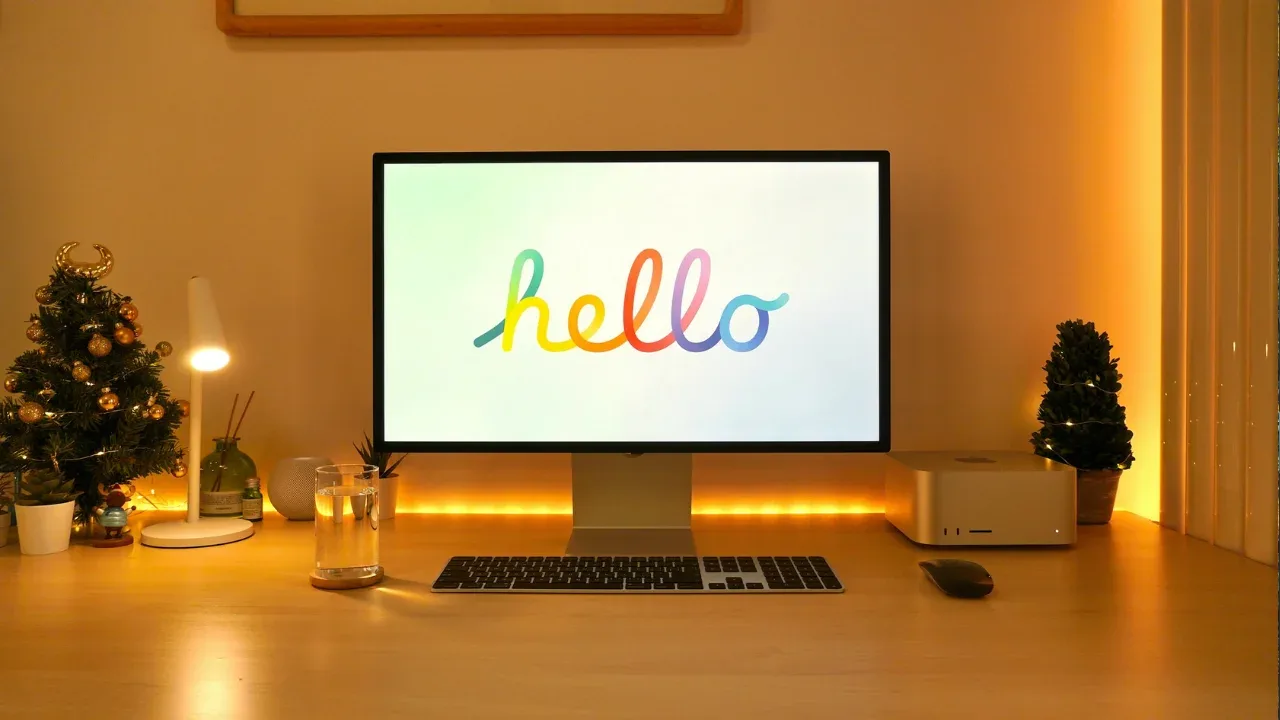
How to Detect Changes in Location Hash 😎
If you're using Ajax and the hash to navigate in your web application, you might have encountered an issue where you need to detect changes in the location hash dynamically. Whether it's checking for changes when the document loads or when the user clicks the back button, we've got you covered! 🙌
The Problem 🤔
Let's say you have a web page with hash-based navigation, like this:
<a href="http://example.com/blah"><strong>#123</strong></a> to <a href="http://example.com/blah"><strong>#456</strong></a>
You want to be able to detect when the hash value changes, such as going from #123
to #456
, so you can take appropriate actions in your JavaScript code.
The Solution ✅
Using the hashchange
Event Listener 🎧
To detect changes in the location hash, you can leverage the hashchange
event listener provided by the window
object. It allows you to execute a callback function whenever the hash changes.
Here's an example of how to use it:
window.addEventListener("hashchange", function() {
// Code to be executed when the hash changes
});
Now, every time the hash changes, the function inside the event listener will be triggered, giving you the opportunity to perform actions based on the new hash value.
Checking the Hash Value on Page Load 📄
If you also need to check the initial hash value when the document loads, you can simply execute the same code outside of the event listener:
// Check the initial hash value
console.log(window.location.hash);
// Add event listener to detect hash changes
window.addEventListener("hashchange", function() {
// Code to be executed when the hash changes
});
Example Implementation 🌟
Let's see a practical example to put everything into context:
function handleHashChange() {
console.log("Hash changed:", window.location.hash);
// Additional code to handle the hash change
}
// Check the initial hash value
console.log("Initial hash:", window.location.hash);
// Add event listener to detect hash changes
window.addEventListener("hashchange", handleHashChange);
In this example, we define a function handleHashChange()
that logs the new hash value when it changes and performs any additional actions you need. We also log the initial hash value on page load.
Conclusion and Call-to-Action 🚀
Detecting changes in the location hash is crucial for building dynamic web applications. By using the hashchange
event listener, you can effortlessly keep track of hash changes and trigger custom actions accordingly.
So go ahead, try implementing this solution in your project and see how it transforms your hash-based navigation! If you have any questions or want to share your experience, leave a comment below. Happy coding! 💻🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
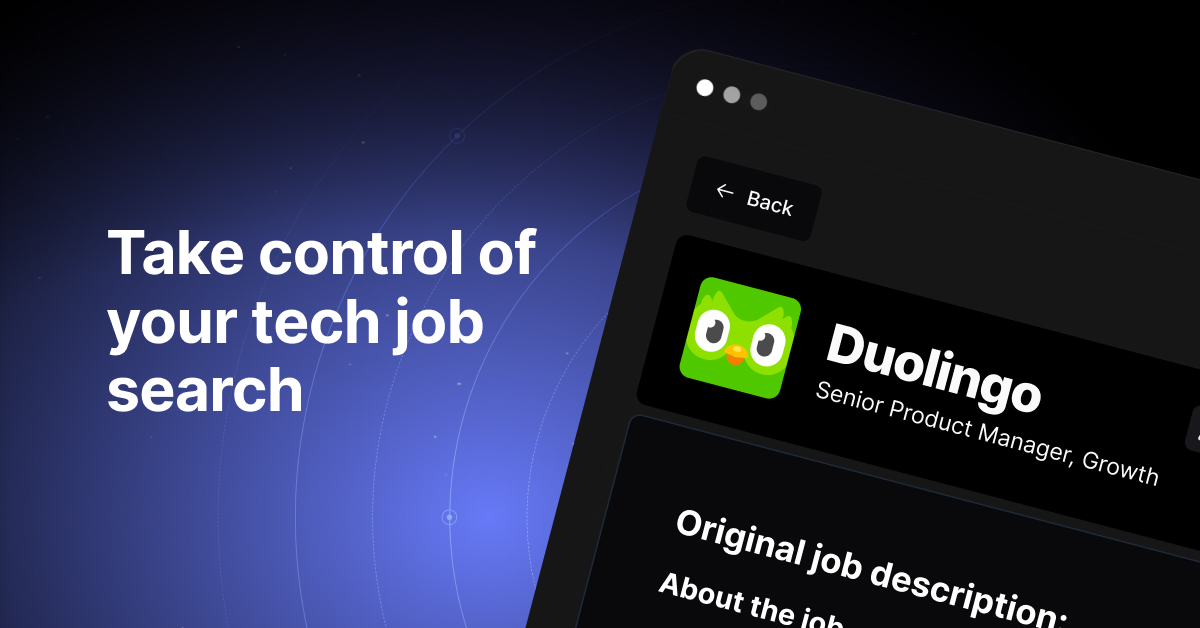