How can I convert the "arguments" object to an array in JavaScript?
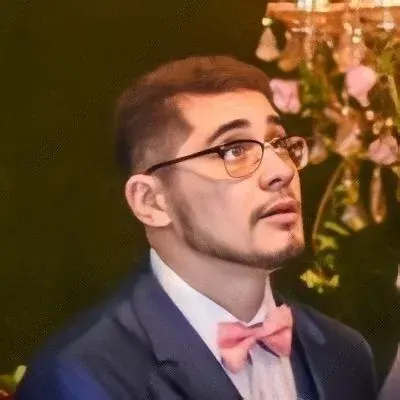
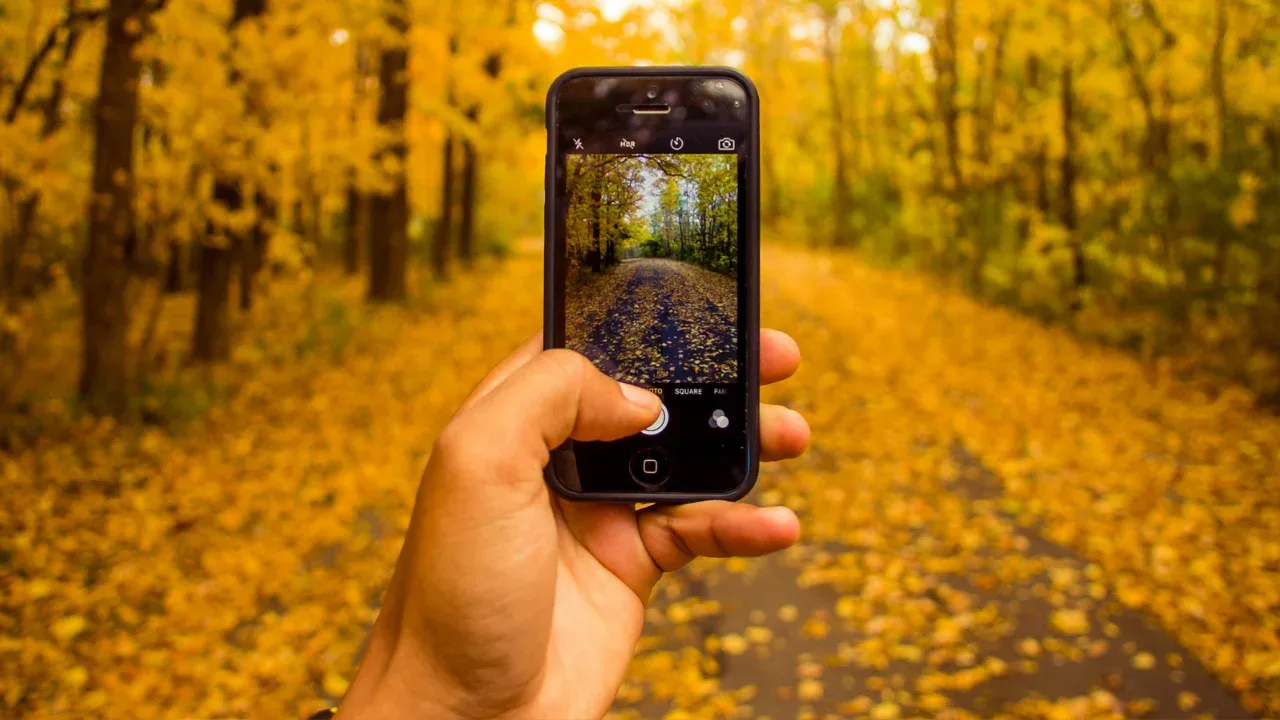
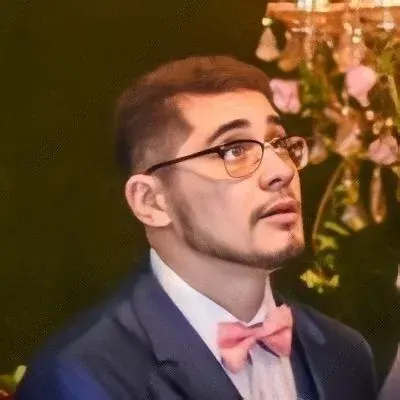
How to convert the π "arguments" object to an array in JavaScript?
π Hey there! So you're looking to β¨convert the "arguments" object to an array in JavaScript, huh? It's not as straightforward as you might hope, but fear not! I've got you covered with some easy solutions. Let's get started! π
The Scoop on the "arguments" Object
First things first, let's quickly go over what the π€ "arguments" object in JavaScript is all about. It's a bit of a π¦ unicorn - it behaves like an array in most situations, but it's not π«actually an array object.
The "arguments" object does not have the useful functions from Array.prototype
like forEach
, sort
, filter
, and map
. That's a bummer, right? π
Solution #1: The Classic For Loop
One way to convert the "arguments" object to an array is by using a simple for loop. It might feel a bit old-fashioned, but hey, it gets the job done! πͺ
Here's an example of a function that sorts its arguments using this approach:
function sortArgs() {
var args = [];
for (var i = 0; i < arguments.length; i++)
args[i] = arguments[i];
return args.sort();
}
Sure, it works, but let's be honest, it's not exactly the most elegant solution out there. We're looking for something better, something built-in! π
Solution #2: The Spread Operator
π Luckily, there is a π built-in way to convert the "arguments" object to an array using the spread operator. π
By using the spread operator (...
), you can extract the individual elements of the "arguments" object and create a new array. Fancy, huh? π
Let's take a look at an example:
function sortArgs(...args) {
return args.sort();
}
Voila! With just one line of code, you've transformed the "arguments" object into a proper array. Time to celebrate! π
Time for Action! π
Now that you know these two solutions, it's time to put them into practice! Choose the one that suits your needs best and give it a go.
Have any other cool solutions up your sleeve? Share them with us! We'd love to hear your thoughts and see how you tackle this problem. π¬
Wrapping Up π
Converting the "arguments" object to an array in JavaScript might seem like a tricky task, but with the right approach, it's totally doable. You've learned two solutions: the classic for loop and the fancy spread operator. π
Next time you encounter the "arguments" object and need to utilize some array functions like forEach
, sort
, filter
, or map
, you'll know exactly what to do. No more frustration, my friend! π
So go ahead and give it a try! Happy coding! π»β¨