How can I convert a string to boolean in JavaScript?
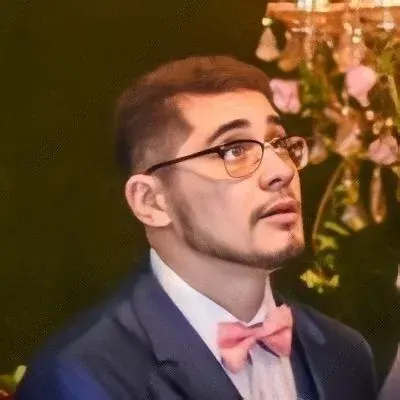
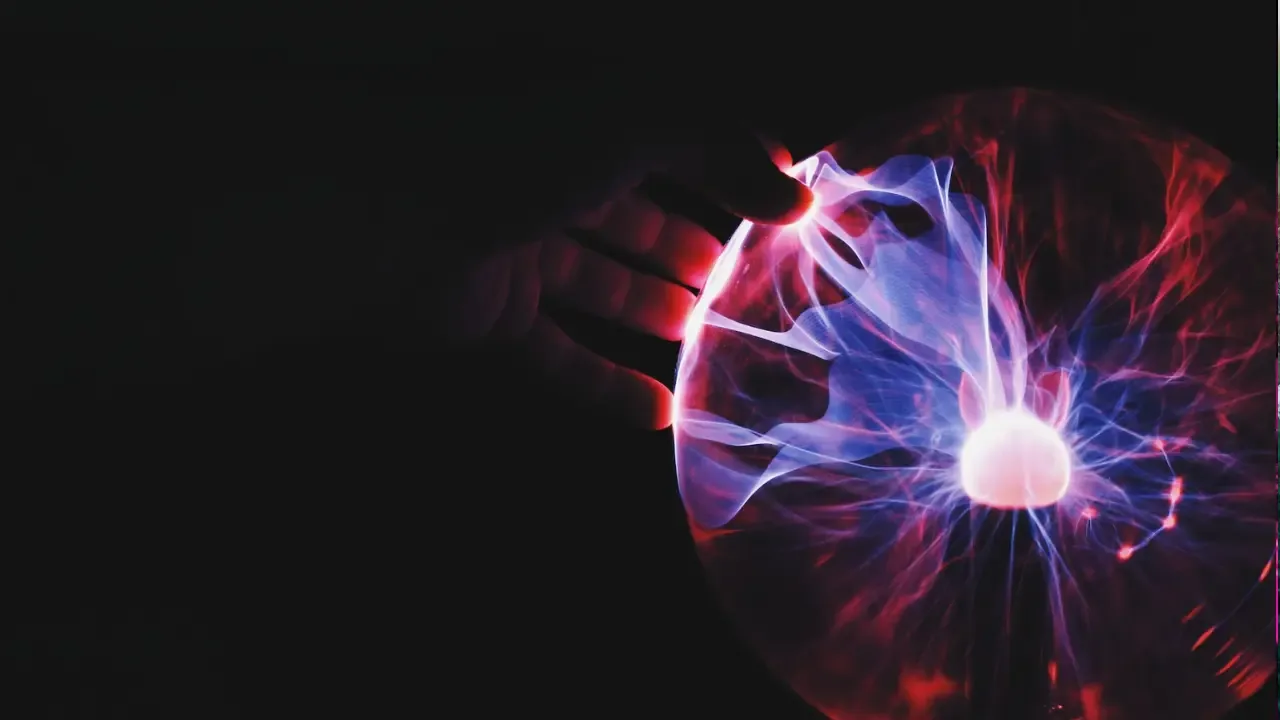
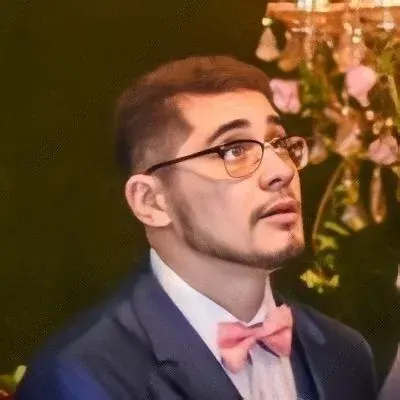
Converting a String to Boolean in JavaScript 🔄🔀🔃
Have you ever encountered a situation where you needed to convert a string representing a boolean value into an actual boolean type in JavaScript? If you have, you're not alone! Many developers face this common challenge when working with form inputs, APIs, or dynamic data.
In this blog post, we'll explore the common issues faced when converting a string to a boolean in JavaScript and provide some easy solutions to simplify your coding life. Let's dive in!
The Problem: String Representation of Booleans in JavaScript ⚠️❓🔢
Imagine you have a hidden form in your HTML, and some of its fields represent boolean values. When updating these fields based on a user's selection, the boolean values might be dynamically populated as strings. This can lead to confusion and potential bugs when you later need to perform boolean operations or comparisons with these values. The question is: how can you convert these string representations back into booleans?
The Traditional Solution: Comparing String Values 🔀🔜✅
The approach you mentioned in the context of the question is to compare the string value to its expected representation. For example, if your string value is 'true', you can convert it to a boolean like this:
var myValue = document.myForm.IS_TRUE.value;
var isTrueSet = myValue == 'true';
While this works, it can be error-prone and cumbersome, especially when dealing with multiple boolean values or string representations. It relies on the assumption that the string value will always be 'true' or 'false' without any variations or inconsistencies.
A Better Solution: Using the JSON.parse()
Method 🌟📝💡
Fortunately, JavaScript provides us with a more elegant and reliable solution using the JSON.parse()
method. This method takes a string argument and attempts to parse it as valid JSON. By leveraging this method, we can convert a string representing a boolean value into a real boolean type effortlessly.
Here's an example of how you can use JSON.parse()
to convert a string to a boolean:
var myValue = document.myForm.IS_TRUE.value;
var isTrueSet = JSON.parse(myValue);
By simply passing the string variable (myValue
) to JSON.parse()
, we get a boolean value without any string comparisons. This solution is not only clearer, but it also handles variations like uppercase 'TRUE' or 'FALSE' and whitespace discrepancies.
Handling Invalid Inputs: Error Handling and Default Values 🚫🔄🙌
It's important to consider scenarios where the string input doesn't represent a valid boolean value, such as 'yes' or 'no'. In these cases, JSON.parse()
will throw a SyntaxError
exception.
To handle such scenarios gracefully, you can enclose the conversion logic within a try-catch block. By doing so, you can catch any errors and provide a default value or perform alternative actions:
var myValue = document.myForm.IS_TRUE.value;
var isTrueSet;
try {
isTrueSet = JSON.parse(myValue);
} catch (error) {
// Handle the error
console.error('Invalid boolean string:', myValue);
// Provide a default value or perform alternative actions
isTrueSet = false;
}
By implementing error handling, you make your code more robust and ensure that it can handle unexpected inputs gracefully.
Call-to-Action: Enhance Your JavaScript Skills 📚🚀🔥
Now that you've learned how to convert a string to a boolean in JavaScript, it's time to apply this knowledge in your own projects! Experiment with different string representations, explore other ways to handle invalid inputs, and share your experiences with the JavaScript community.
If you found this blog post helpful, don't forget to share it with your fellow developers. Let's spread the knowledge and make the coding world a better place!
Happy coding! 😄👩💻👨💻