How can I convert a comma-separated string to an array?
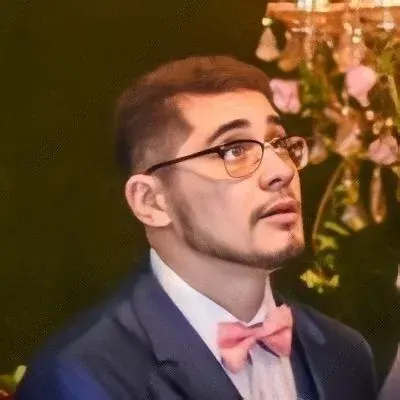
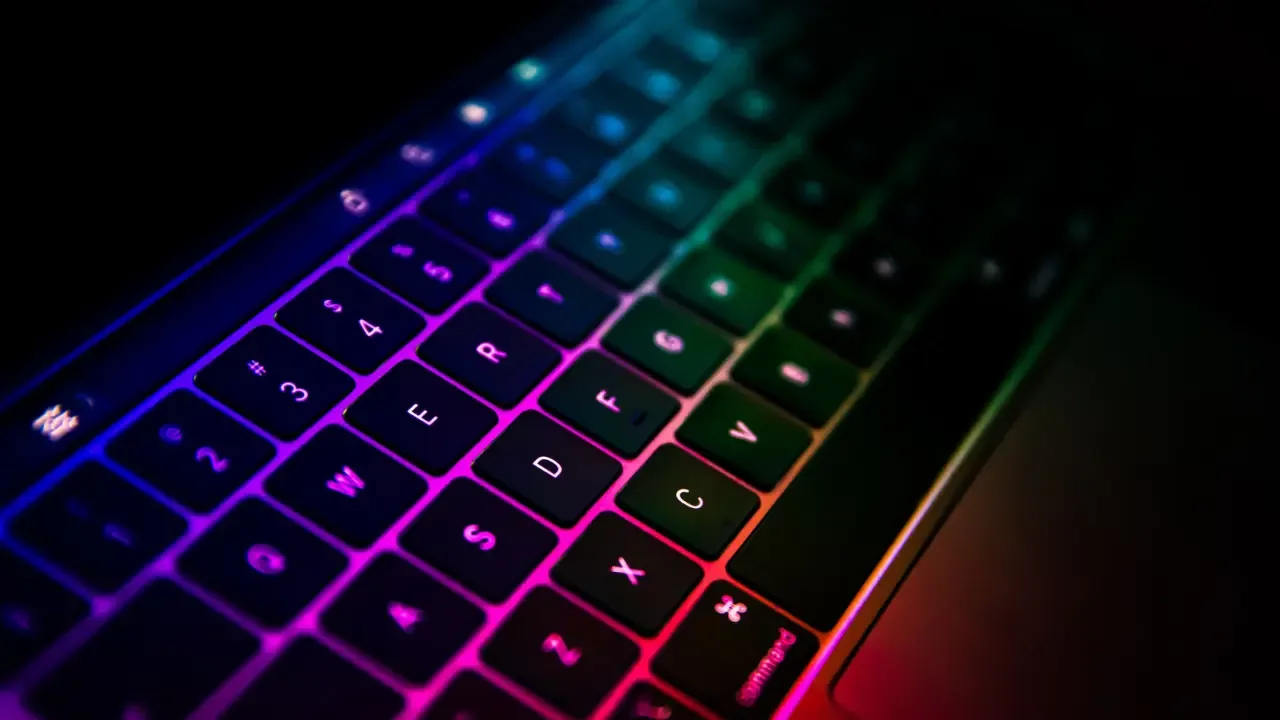
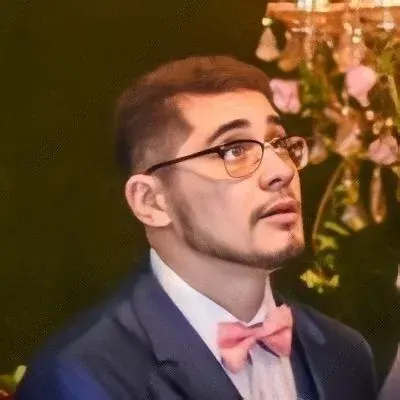
šš HOW TO CONVERT A COMMA-SEPARATED STRING TO AN ARRAY šš
šāØ Are you tired of dealing with comma-separated strings when you really just want an array? Look no further! In this guide, we'll show you easy and efficient ways to convert a comma-separated string into an array. Let's dive in! šāØ
š The Key Issue: You have a comma-separated string and you need to convert it into an array for further manipulation.
š” Easy Solution: There are a couple of ways to tackle this problem. Let's explore two popular options:
1ļøā£ Using the split()
method:
The split()
method is a convenient built-in function that splits a string into an array of substrings based on a specified separator. In our case, the comma is the separator.
Here's an example code snippet:
var str = "January,February,March,April,May,June,July,August,September,October,November,December";
var array = str.split(",");
console.log(array);
Running the code above will give you the desired output:
["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]
2ļøā£ Using the split()
method with map()
:
If you want to go a step further and trim any leading or trailing spaces in each substring, you can combine the split()
method with the map()
method. This allows you to apply a trimming function to each element of the resulting array.
Here's an example code snippet:
var str = "January, February, March, April, May, June, July, August, September, October, November, December";
var array = str.split(",").map(item => item.trim());
console.log(array);
Running the code above will give you the desired output:
["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]
The trim()
method removes any leading and trailing spaces from a string, ensuring that your array elements are clean and consistent.
šš„ Your Turn: Now that you know how to convert a comma-separated string to an array, it's time to put it into action! šāØ Try it out in your own code, and see how this method simplifies your workflow.
š„š Engage with Us: If you have any questions, suggestions, or fun ways you've used this technique in your projects, feel free to share them in the comments below! We'd love to hear from you and learn about your experiences. Let's connect and grow together! š¤šŖ
šāļø So, what are you waiting for? Start converting those comma-separated strings to arrays and unleash the power of data manipulation in your projects today! Happy coding! š©āš»šØāš»š»
āØšāØ