How can I communicate between related react components?
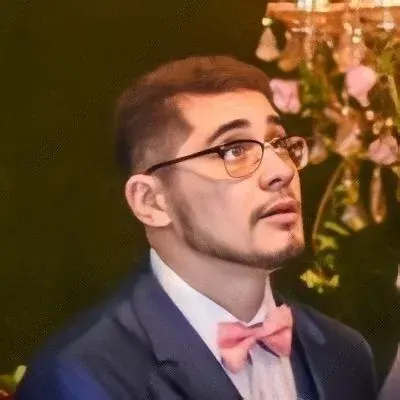
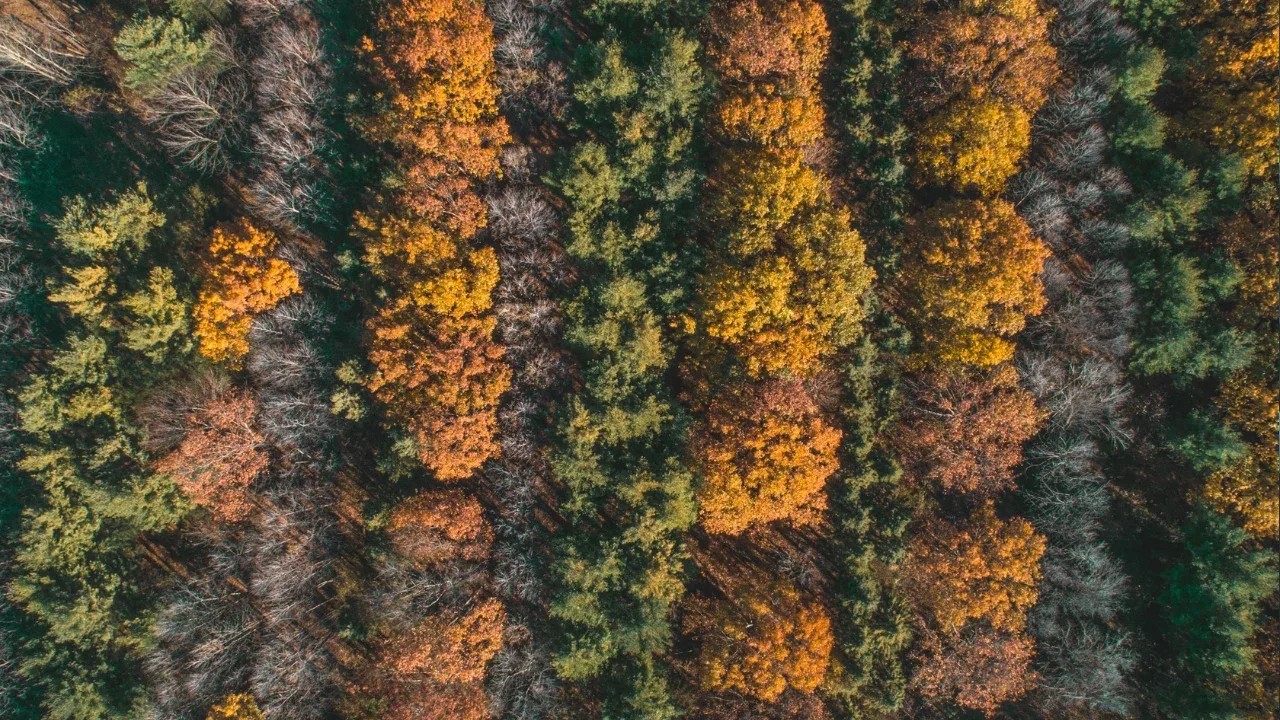
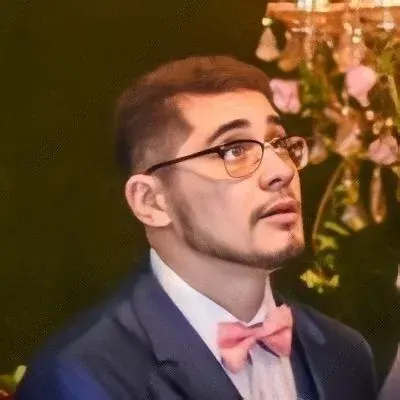
How to Communicate Between Related React Components? 🗣️
So, you're diving into the amazing world of ReactJS and you've encountered a common challenge - how do you make related components interact with each other?! 😱
Fear not, my fellow React enthusiasts! In this guide, we'll explore some easy solutions to help you establish efficient communication between your React components. By the end of this post, you'll be flexing your React muscles like a pro! 💪
The Problem: Inter-component Communication 💬
Let's take a look at the predicament you find yourself in. Your application consists of three components: <List />
, <Filters />
, and <TopBar />
. You want to trigger a method in <List />
whenever you change settings in <Filters />
. Sounds familiar, doesn't it? 🤔
Solution 1: Lifting State Up ⬆️
One of the fundamental concepts in React is the idea of lifting state up. Basically, you can manage shared state between components by moving it up to their closest common ancestor. In this case, you could create a state container in a higher-level component that holds the shared state between <List />
and <Filters />
. 📦
Here's how you can do it:
Create a parent component, let's call it
<App />
, that wraps<List />
,<Filters />
, and<TopBar />
.Implement the necessary state in
<App />
that needs to be shared between the components.Pass the state and any required functions as props to the child components.
Update the state in
<App />
whenever a change occurs in<Filters />
.<List />
can now access the updated state through props and re-render based on the changes.
// App.jsx
import React, { useState } from "react";
import List from "./List";
import Filters from "./Filters";
import TopBar from "./TopBar";
const App = () => {
const [filterValue, setFilterValue] = useState("");
const handleFilterChange = (value) => {
setFilterValue(value);
};
return (
<div>
<TopBar />
<Filters onFilterChange={handleFilterChange} />
<List filterValue={filterValue} />
</div>
);
};
export default App;
By lifting the shared state up to the parent component, you enable communication between <List />
and <Filters />
, keeping your components in sync. 🔄
Solution 2: Context API 🌐
If your components are deeply nested and passing props all the way down becomes cumbersome, React's Context API comes to the rescue! The Context API allows you to share state without having to pass the props explicitly through intermediate components. 👐
Let's see how it works:
Create a context using the
createContext
function from React.Use the
Provider
component to wrap the parent component,<App />
, in this case.Set the shared state in the
value
prop of theProvider
.In child components, use the
useContext
hook to access the shared state.
// AppContext.jsx
import React, { createContext, useState } from "react";
export const AppContext = createContext();
const AppProvider = ({ children }) => {
const [filterValue, setFilterValue] = useState("");
const handleFilterChange = (value) => {
setFilterValue(value);
};
return (
<AppContext.Provider value={{ filterValue, handleFilterChange }}>
{children}
</AppContext.Provider>
);
};
export default AppProvider;
// App.jsx
import React from "react";
import List from "./List";
import Filters from "./Filters";
import TopBar from "./TopBar";
import AppProvider from "./AppContext";
const App = () => {
return (
<AppProvider>
<div>
<TopBar />
<Filters />
<List />
</div>
</AppProvider>
);
};
export default App;
// Filters.jsx
import React, { useContext } from "react";
import { AppContext } from "./AppContext";
const Filters = () => {
const { handleFilterChange } = useContext(AppContext);
const handleChange = (event) => {
handleFilterChange(event.target.value);
};
return (
<div>
<input type="text" onChange={handleChange} />
</div>
);
};
export default Filters;
By leveraging the Context API, you can seamlessly share state across multiple components without the hassle of prop drilling. 🎉
Conclusion and Call-to-Action 🚀
And there you have it! Two powerful strategies to communicate between related React components - lifting state up and using the Context API. These techniques will empower you to build robust and efficient React applications. 🏗️
Now, it's your turn to put your newfound knowledge into action! Try implementing these approaches in your own projects and see the magic happen. If you have any more questions or want to share your experiences, feel free to drop a comment below. Let's keep the conversation going! 💬