How can I check if an object is an array?
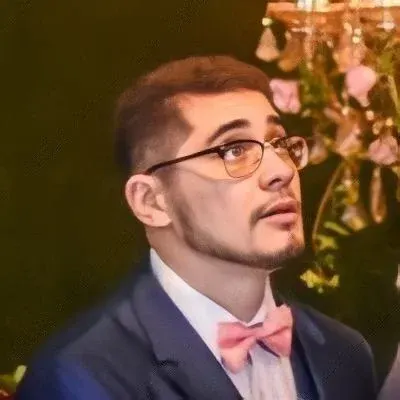
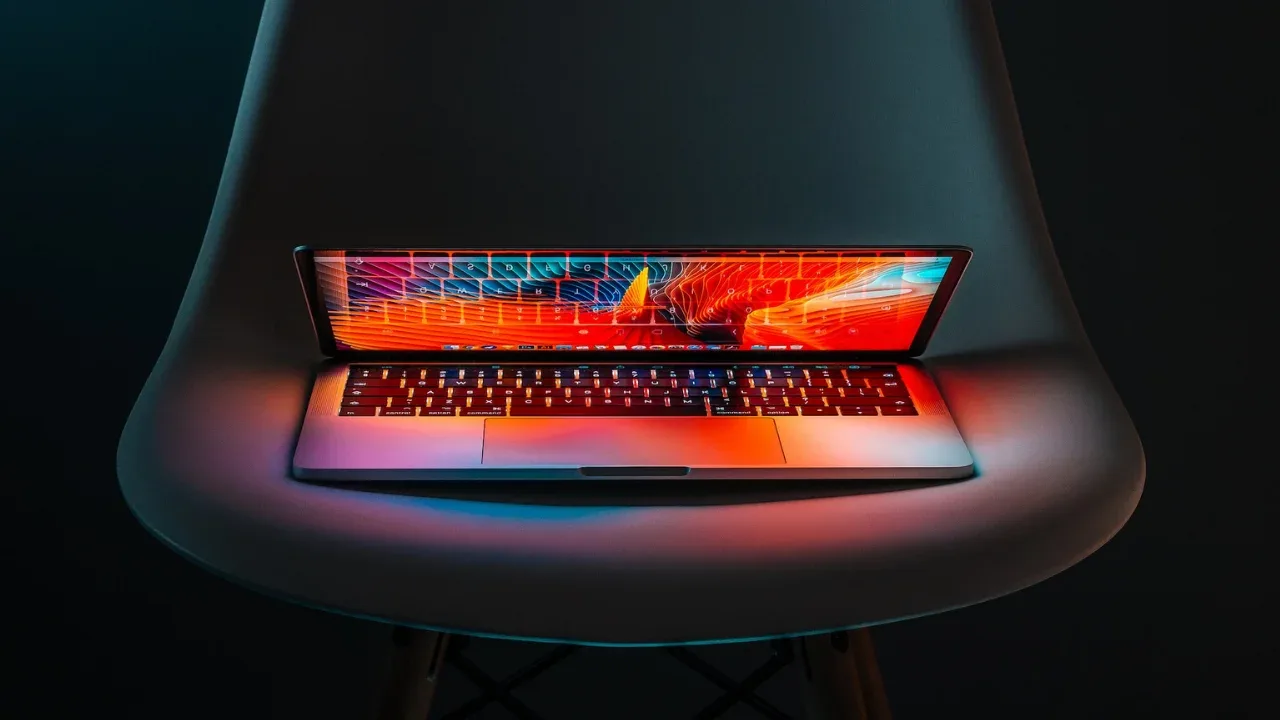
Is it an Array? 🧐
Are you working on a function that needs to be able to handle both a list of strings and a single string? Fear not! Checking whether a variable is an array is easier than you might think.
The Problem 🚩
You want to write a function that accepts either a list of strings or a single string. But if you receive a single string, you need to convert it to an array before looping over it. So how can you tell if the variable is already an array or not?
The Solution 💡
To check if a variable is an array, you can use the Array.isArray()
method in JavaScript. This method returns true
if the variable is an array, and false
otherwise. Let's see an example:
function processInput(input) {
if (Array.isArray(input)) {
// It's an array, do something with it
console.log("Input is an array:", input);
} else {
// It's a single string, convert it to an array
const array = [input];
console.log("Input is a single string, converted to an array:", array);
}
}
// Testing the function
const list = ["apple", "banana", "orange"];
const singleString = "pineapple";
processInput(list); // Output: "Input is an array: ['apple', 'banana', 'orange']"
processInput(singleString); // Output: "Input is a single string, converted to an array: ['pineapple']"
Going the Extra Mile ⭐️
If you want to further ensure that the variable is an array, you can also check its constructor
property. Arrays in JavaScript are objects, and their constructor
property is set to Array
. Here's how you can use it:
if (Array.isArray(input) && input.constructor === Array) {
// It's definitely an array
}
Conclusion 👏
Checking if a variable is an array is crucial when developing functions that need to handle different input types. By using Array.isArray()
, you can easily determine whether the variable is an array or not, and take the appropriate actions.
So next time you find yourself wondering "Is it an array?", remember these handy tips. Happy coding! 🚀
Now it's your turn! Have you ever encountered a similar situation? Share your experiences in the comments below! Let's learn from each other. 👇
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
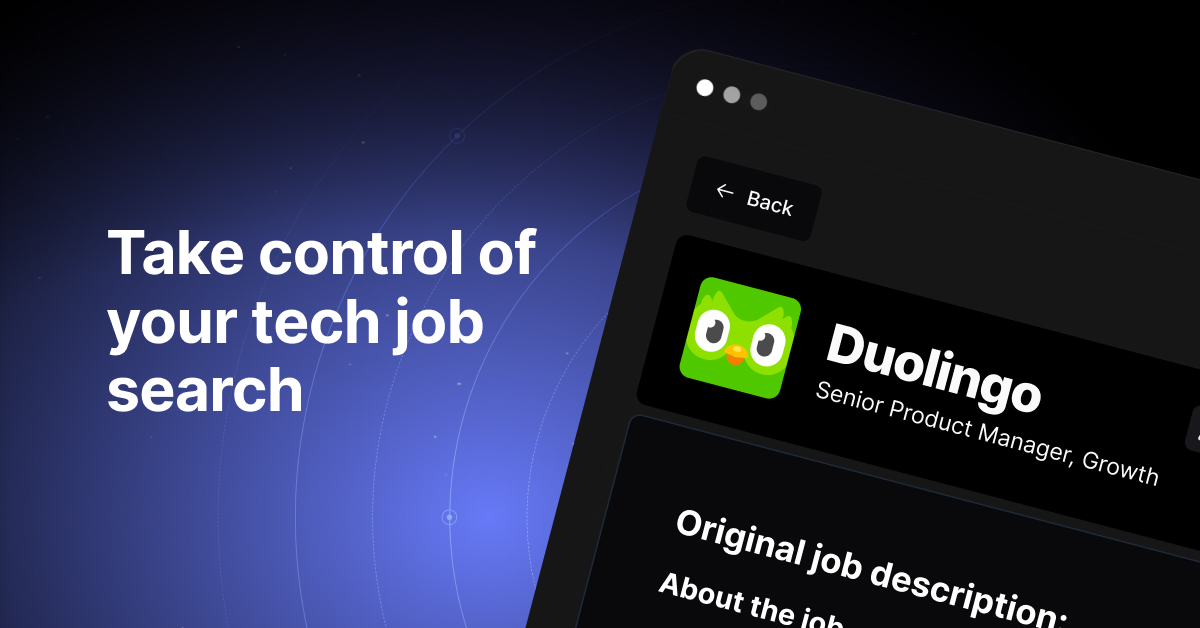