How can I check for "undefined" in JavaScript?
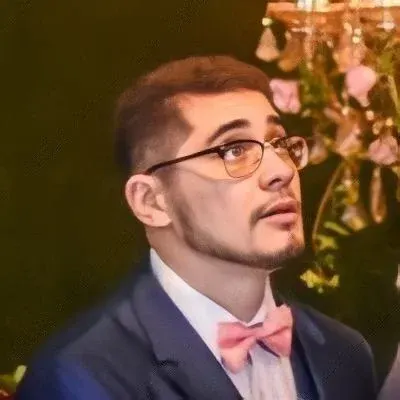
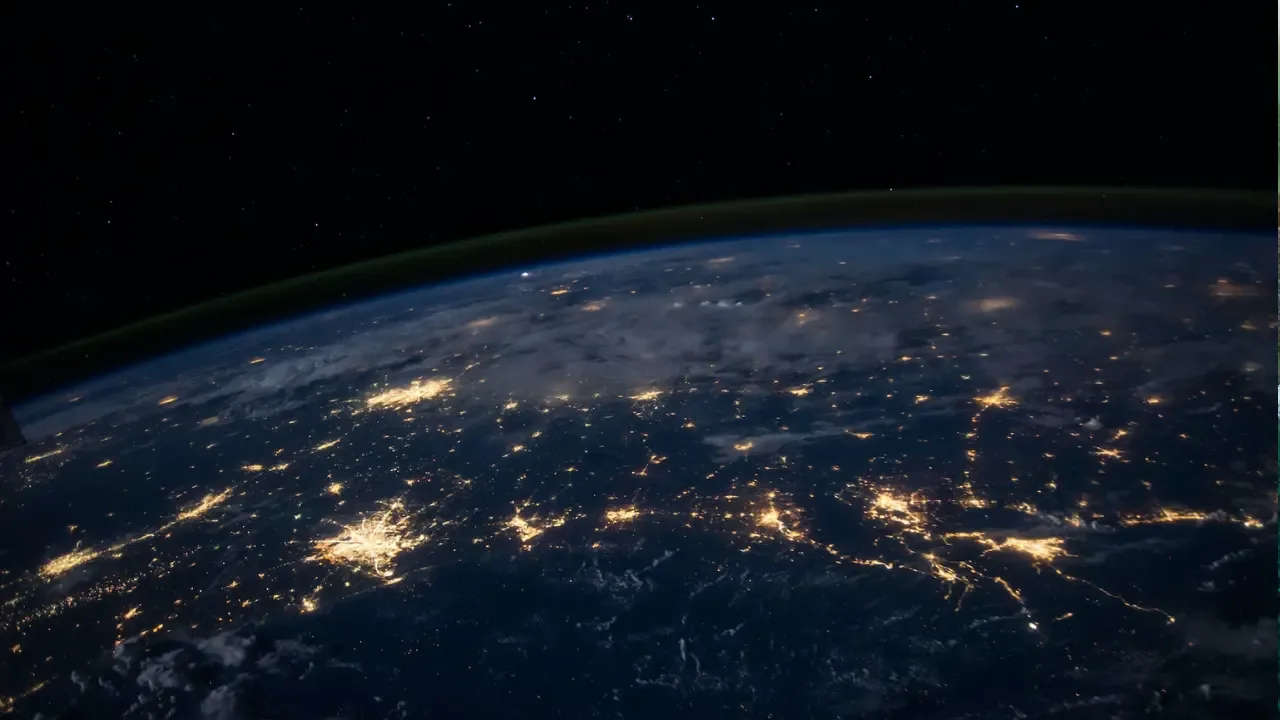
Understanding "undefined" in JavaScript: Easy Solutions to Check and Handle It
š Hey there JavaScript enthusiasts! š
JavaScript is a powerful language that allows us to create amazing web applications. However, handling undefined values can sometimes be a bit tricky. Fear not, though! In this blog post, we will explore common issues associated with checking for "undefined" in JavaScript and provide easy solutions to overcome them. So let's dive in! šŖ
The Quest to Check for "undefined"
if (window.myVariable)
if (typeof(myVariable) != "undefined")
if (myVariable)
When it comes to checking if a variable is undefined, JavaScript offers multiple approaches. Let's break down each of the above methods and discuss their pros and cons.
Method 1: if (window.myVariable)
This approach relies on checking the variable's existence in the global scope using the window
object. It assumes that if a variable is defined, it will exist in the global scope. While this method might work in some cases, it has a major drawback.
š« The Drawback: If the variable is only defined within a function or a specific scope, this check might not accurately determine if the variable is truly undefined.
Method 2: if (typeof(myVariable) != "undefined")
This method uses the typeof
operator to check if the type of the variable is not "undefined". It is a more reliable approach as it specifically targets the type of the variable. However, it does have a potential pitfall to consider.
ā ļø The Pitfall: If you accidentally misspell the variable name in the check, it will create a new global variable instead of checking the intended variable.
Method 3: if (myVariable)
This method seems simple and concise, right? It relies on JavaScript's truthy/falsy concept. If the variable is defined and has a truthy value, the condition will be true. However, beware of using it without proper precautions.
š„ The Danger: If the variable is undefined, using this method will throw an error, halting the execution of your code.
The Ultimate Solution: A Combination of Methods
To ensure better reliability and avoid unintended consequences, we can combine methods 2 and 3. Here's an improved approach:
if (typeof myVariable !== "undefined" && myVariable)
This combination covers both scenarios. It first checks if the variable's type is not "undefined" using typeof
. Then, it ensures the variable has a truthy value without triggering an error.
Handling Undefined Values in Practice
Now that we know how to check for "undefined," let's see how we can handle it in practical situations.
Example 1: Assigning a Default Value
const myVariable = someFunctionThatMightReturnUndefined() || defaultValue;
This example demonstrates using the logical OR operator (||
) to assign a default value to a variable if it is undefined. If the function returned an undefined value, the variable will be assigned the defaultValue
.
Example 2: Safe Access to Object Properties
const propertyValue = myObject?.nestedObject?.property;
The optional chaining operator (?.
) allows us to safely access nested properties of an object. If any intermediate property is undefined, the expression will short-circuit and return undefined instead of throwing an error.
Engage With Us!
We hope this guide has cleared up any confusion you had about checking for "undefined" in JavaScript. š
Now it's your turn to take action! Have you encountered any unique situations involving "undefined" in JavaScript? Share your stories and creative solutions in the comments below. Let's learn and grow together as a community! š
Stay tuned for more exciting JavaScript tips and tricks. Happy coding! š»āØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
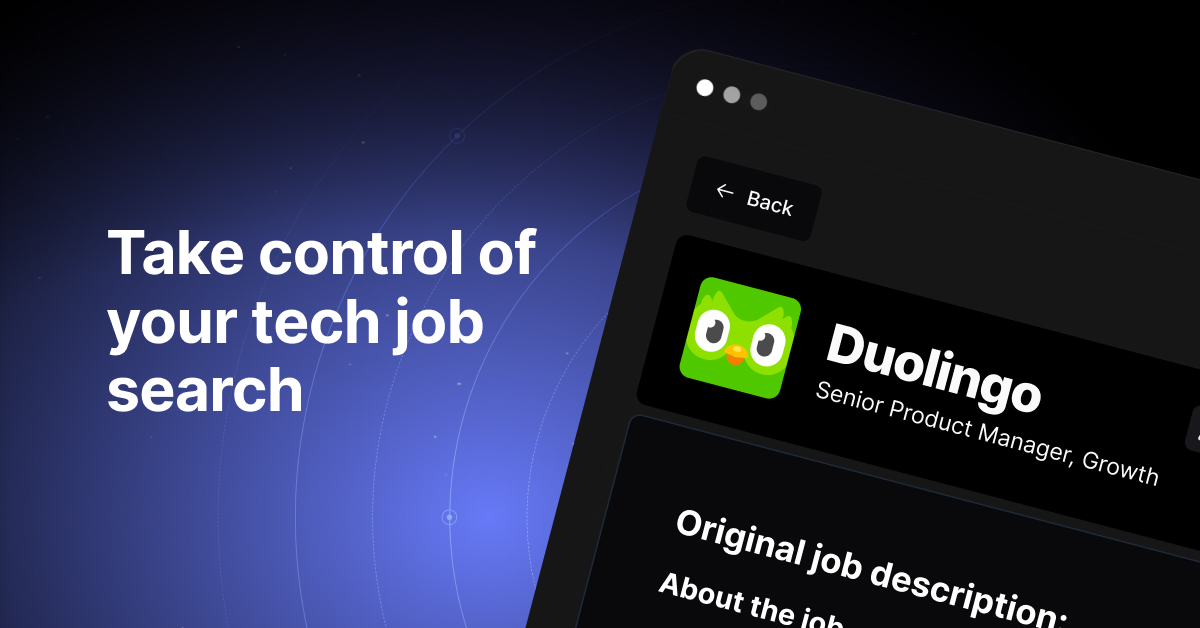