How can I change an element"s class with JavaScript?
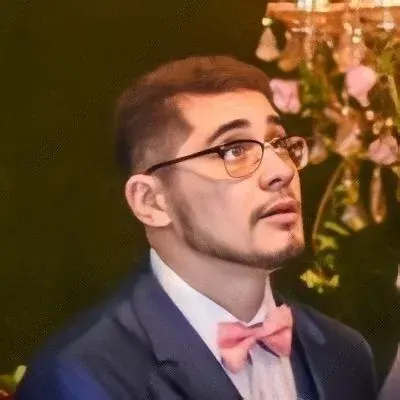
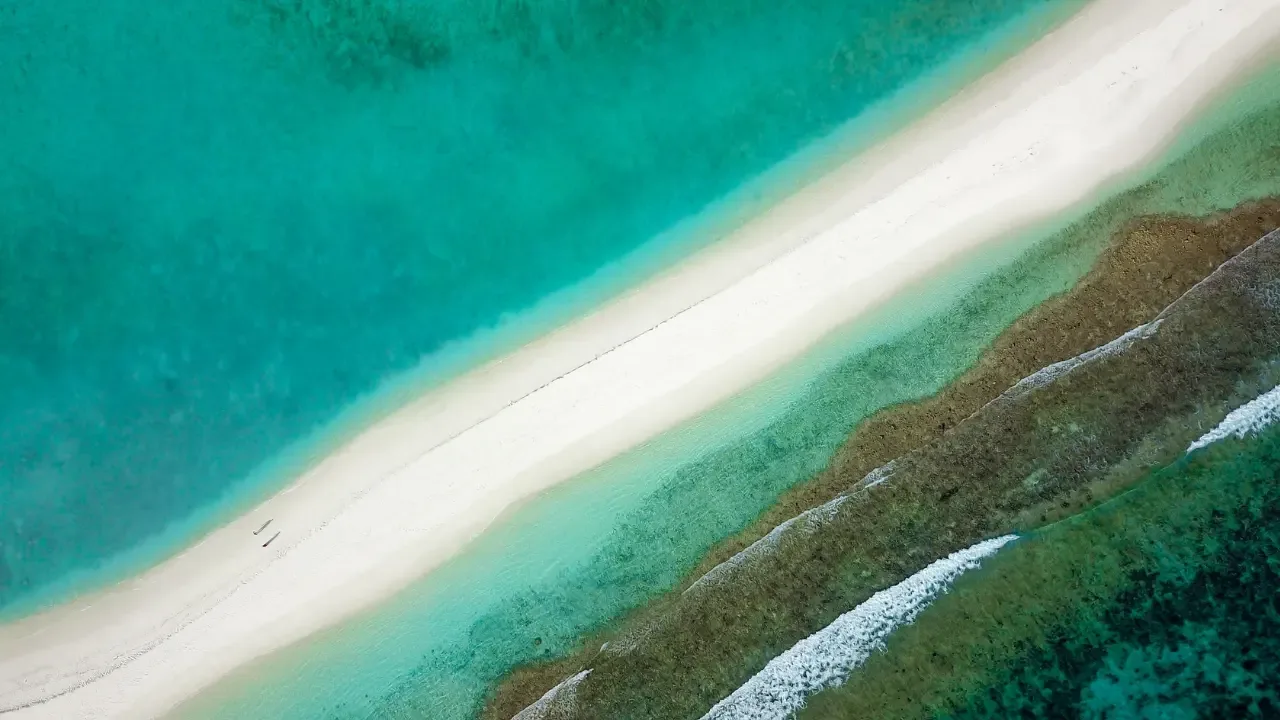
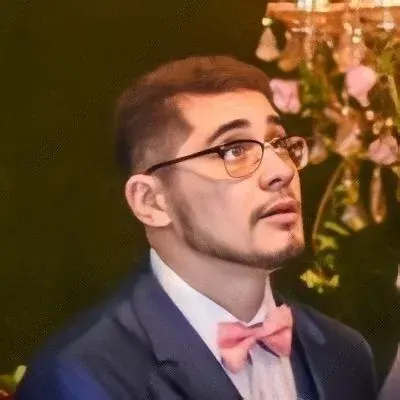
How to Change an Element's Class with JavaScript: A Simple Guide ๐จโ๐ป
Have you ever wondered how to dynamically change an element's class in your HTML using JavaScript? ๐ค Whether you want to apply different styles to an element based on user interaction or modify its behavior, understanding this concept is crucial for web developers. ๐ช
In this blog post, we'll explore several easy solutions that will enable you to effortlessly change an element's class with JavaScript. Whether you're a seasoned developer or just starting your coding journey, we've got you covered! Let's dive right in! ๐โโ๏ธ
Understanding the Problem
Before we jump into the solutions, let's quickly recap the problem we're trying to solve. Essentially, we want to be able to modify an element's class attribute in response to a particular event, such as a button click. This allows us to apply different styles, behavior, or functionality to the element dynamically. ๐จโ๏ธ
Solution 1: Using the classList
Property
One of the easiest and most intuitive ways to change an element's class is by utilizing the classList
property provided by JavaScript. This property grants us access to a set of methods that make manipulating classes a breeze. Here's an example:
const element = document.getElementById('myElement');
// Adding a class
element.classList.add('newClass');
// Removing a class
element.classList.remove('oldClass');
// Toggling a class
element.classList.toggle('active');
In the code snippet above, we first obtain a reference to the desired element using its id
with the getElementById()
method. Then, we can modify its class by utilizing the add()
, remove()
, and toggle()
methods, depending on our desired outcome. ๐
Solution 2: Directly Modifying the className
Property
Another approach you can take is to directly manipulate the className
property of the element. Although slightly less flexible than the previous solution, it is still a viable option and might be more straightforward for some cases. Take a look at this example:
const element = document.getElementById('myElement');
// Changing the class
element.className = 'newClassName';
By assigning a new class name to the className
property, you effectively change the element's class. Keep in mind that this will replace all existing classes with the new one, so use this method when you only need to set a single class. ๐ญ
Putting it All Together โจ
Now that you have two solid solutions at your disposal, it's time to put them to use! Experiment with both methods in your projects and select the one that best fits your needs. Remember, coding is all about creativity and problem-solving, so feel free to explore and customize these solutions as much as you want! ๐กโ๏ธ
Your Turn: Try it Out! ๐
We've covered the fundamental techniques for changing an element's class with JavaScript. Now it's your turn to apply what you've learned! Open up your code editor, create a new HTML file, and start experimenting with different events and classes. Share your creations with us in the comments below! ๐ฌ๐
Conclusion
Changing an element's class using JavaScript is a powerful tool in your web development arsenal. With the help of the classList
property or by directly modifying the className
property, you can effortlessly modify an element's behavior, style, or functionality based on user interactions. Remember, understanding these concepts opens up a whole new world of possibilities for you as a developer! Happy coding! ๐ปโจ