How can I beautify JSON programmatically?
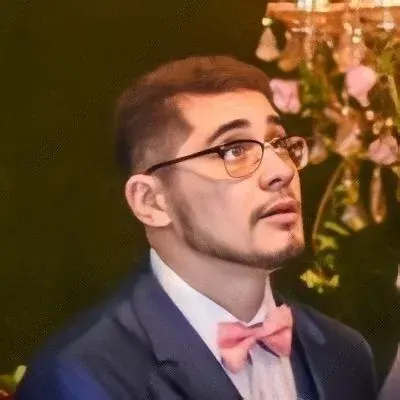
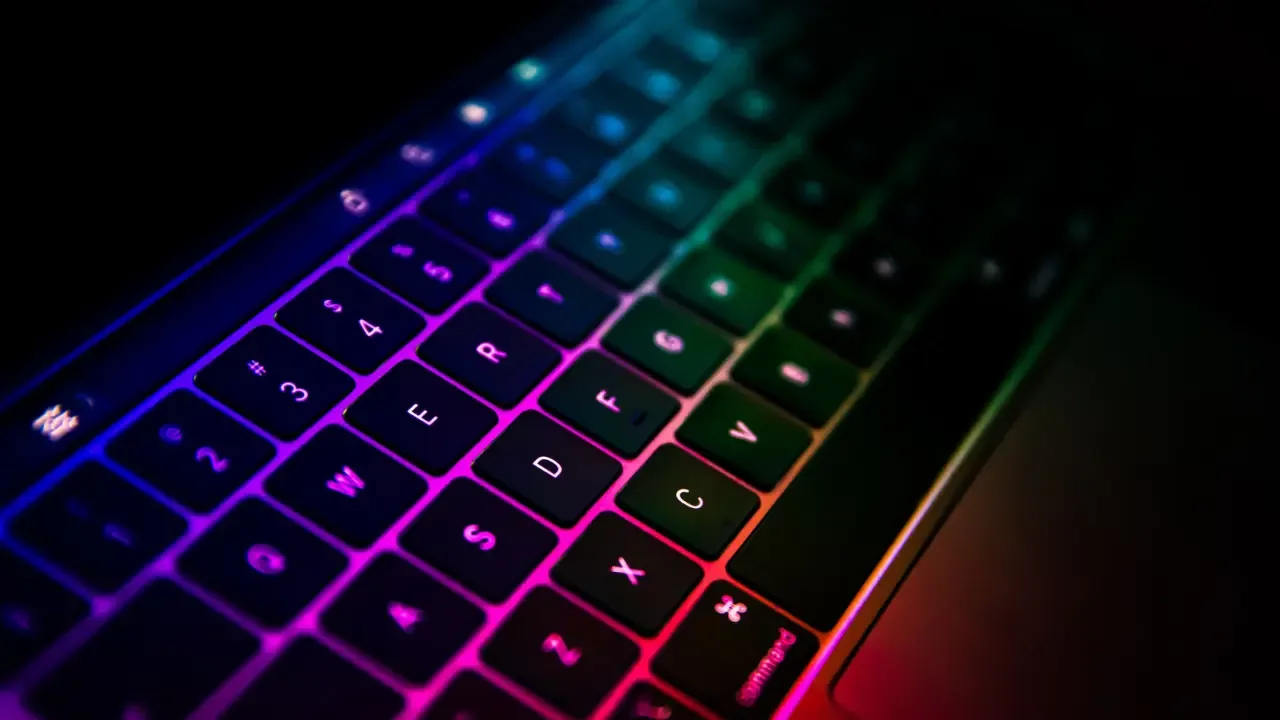
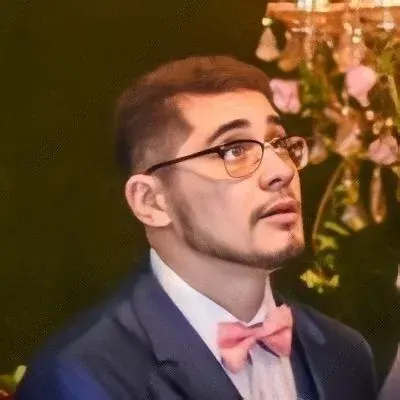
📝 Beautifying JSON Programmatically: Making Your Code Look Good!
Are you tired of these unruly JSON strings cluttering up your code? Yeah, me too! But don't worry, I've got your back! In this blog post, I'll show you how to beautify JSON programmatically using JavaScript. By the end of this guide, your JSON will be looking so fine, you won't recognize it!
The Problem
Imagine this scenario: You've just received a humongous JSON object from your API, and it looks like a messy jumble of characters. It's nearly impossible to read and decipher what's going on. 😫
You want to transform this ugly JSON string into a beautifully formatted, easy-to-read version. Something like this:
{
"name": "Steve",
"surname": "Jobs",
"company": "Apple"
}
The Solution
To solve this conundrum, you need the help of a "JSON Beautifier" – a tool that can programmatically format your JSON into a visually pleasing layout. Luckily, JavaScript has a built-in method called JSON.stringify()
which can do just that!
Here's how you use it:
const jsonObj = {
"name": "Steve",
"surname": "Jobs",
"company": "Apple"
};
const beautifiedJson = JSON.stringify(jsonObj, null, 2);
console.log(beautifiedJson);
By passing jsonObj
as the first argument and adding null, 2
as the second and third arguments while calling JSON.stringify()
, we achieve the desired result. The null
here is for a replacer function (not needed for beautification purposes) and the 2
is the number of spaces for indentation. You can change the indentation value as per your preference.
Putting It Into Action
Let's see this in action! Imagine you have a function called beautifyJson()
which applies the beautification process to any JSON object:
function beautifyJson(jsonObj) {
return JSON.stringify(jsonObj, null, 2);
}
const messyJson = {
"name": "Steve",
"surname": "Jobs",
"company": "Apple"
};
const beautifulJson = beautifyJson(messyJson);
console.log(beautifulJson);
When you run this code, you'll get the desired result: a beautifully formatted JSON object that is easy on the eyes. 🌟
Share Your Beautified JSON!
Now that you know how to beautify JSON programmatically using JavaScript, it's time to put it into practice! Take your messy JSON strings and transform them into clean, organized code. Share your before-and-after JSON examples in the comments section below and let's see who can create the prettiest JSON! 🎉
Happy coding! 💻
What are your favorite tools or techniques for working with JSON in JavaScript? Share your insights in the comments below and let's make the world of JSON a more beautiful place!