How can I add a custom HTTP header to ajax request with js or jQuery?
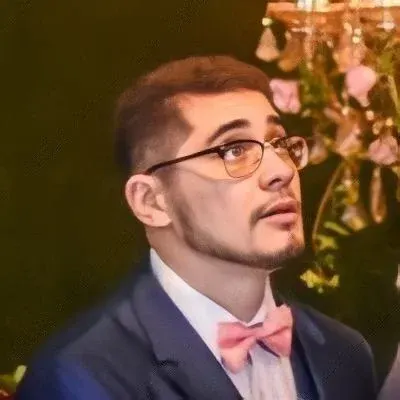
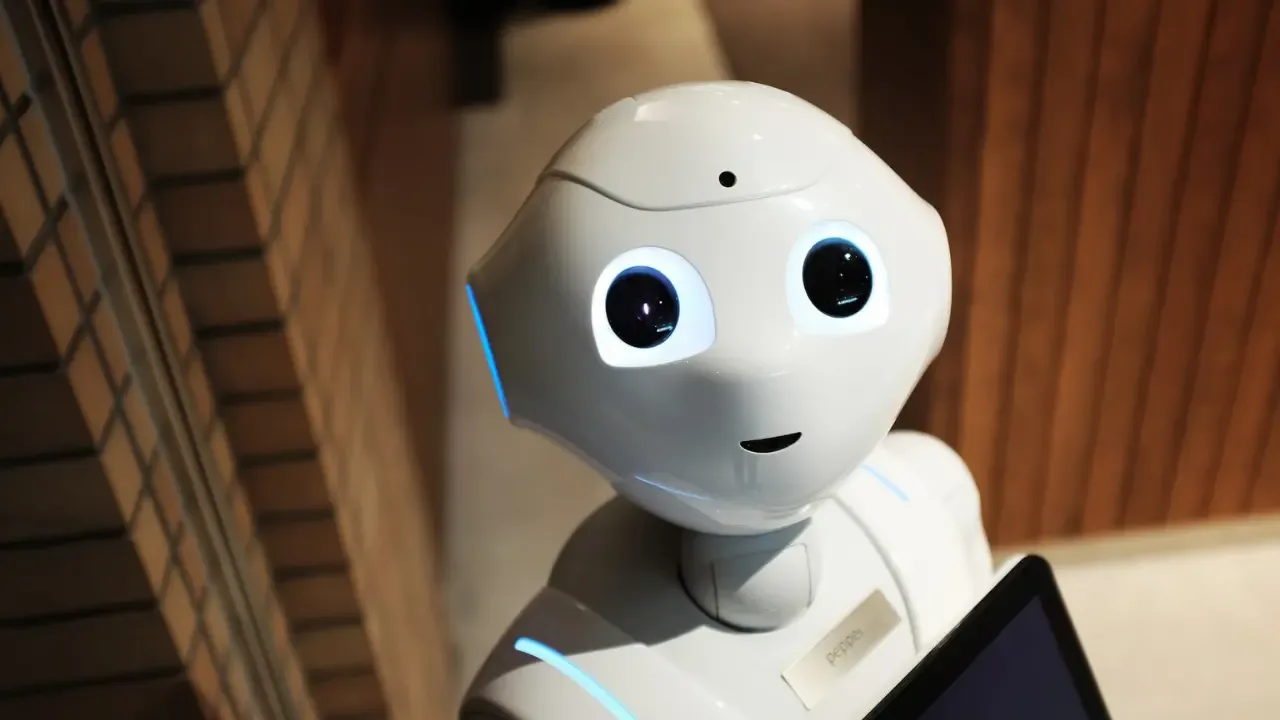
🎉📝 Tech Talk: Adding a Custom HTTP Header to AJAX Request with JS or jQuery 🎉📝
Hey there, tech enthusiasts! 👋 In today's blog post, we'll dive into the world of custom HTTP headers 📦 and explore how we can add them to AJAX requests using JavaScript and jQuery. So, if you've been wondering how to tackle this challenge, you've come to the right place! Let's get started. 💪
So, you want to add or create a custom HTTP header to your AJAX request, huh? Well, fret not! We've got you covered. 😎
Here's a simple code snippet to add a custom HTTP header using pure JavaScript:
var xhttp = new XMLHttpRequest();
xhttp.open("GET", "your-url-here", true);
xhttp.setRequestHeader("Custom-Header", "your-header-value-here");
xhttp.send();
In the code above, we create a new XMLHttpRequest object using JavaScript. Then, we use the setRequestHeader()
method to set our custom header by providing the header name and its value. Finally, we send the AJAX request using the send()
method. Voila! 🌟
But wait, there's more! We know many of you love using jQuery for its simplicity and elegance. So, here's how you can add a custom HTTP header using jQuery:
$.ajax({
url: "your-url-here",
type: "GET",
beforeSend: function(xhr){
xhr.setRequestHeader("Custom-Header", "your-header-value-here");
},
success: function(data){
// Handle the response here
}
});
In the jQuery snippet above, we utilize the beforeSend
option provided by the $.ajax()
method. This option allows us to modify the XMLHttpRequest object before it is sent. Within this function, we use the setRequestHeader()
method to set our custom header.
Now that you have two different approaches for setting custom HTTP headers, it's time to put them to good use! But, before you do, let's address a common issue many developers encounter.
⚠️ Common Pitfall: Cross-Origin Resource Sharing (CORS) ⚠️ When making AJAX requests, you might encounter issues related to Cross-Origin Resource Sharing (CORS). This security feature prevents scripts from making requests to a different origin than the one that served the script. To overcome CORS restrictions, you need to configure your server to allow these requests. This involves setting appropriate response headers on the server-side.
Once you've tackled the CORS hurdle, it's time to seize the opportunities that custom headers offer you. Whether it's passing authentication tokens, API keys, or any other custom data, custom headers empower you to tailor your requests to your specific needs. 🚀
So, what are you waiting for? Go ahead and add your custom HTTP headers to your AJAX requests using either JavaScript or jQuery. The possibilities are endless! 💡
Remember, mastering these techniques opens up a whole new realm of possibilities and solutions for your projects. We hope this guide has been valuable to you!
👉 Now, it's your turn! Have you ever encountered challenges while working with custom HTTP headers? Share your experiences, tips, and tricks in the comments below. Let's learn from each other and grow together! 🌟✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
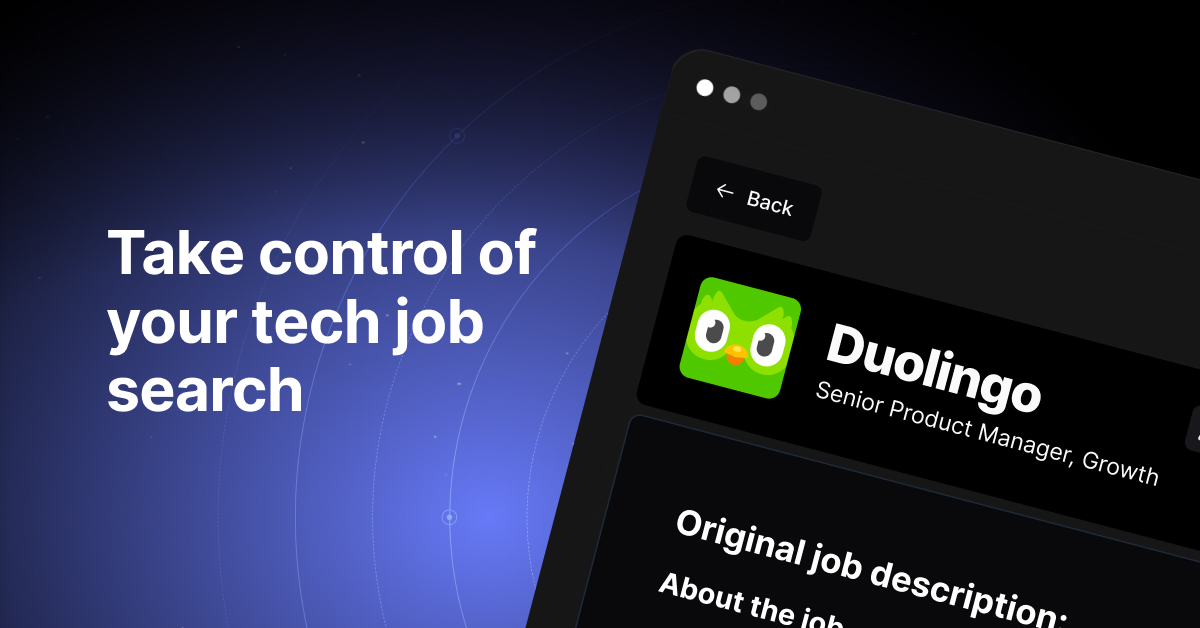