How can I access the value of a promise?
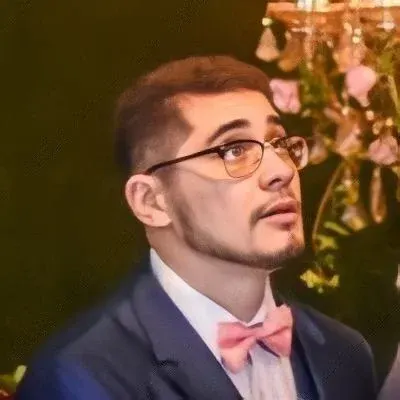

How to Access the Value of a Promise? 🤔💭
Promises are a common concept in JavaScript that allow us to handle asynchronous operations and make our code more organized and readable. However, understanding how to access the value of a promise can sometimes be confusing. 🤷♂️
Let's dive into the example you provided from Angular's documentation and break it down step by step. By the end of this guide, you'll have a clear understanding of how to access the value of a promise! 🚀
Understanding Promise Chaining 🌟🔗
In the example you mentioned, the code snippet is using promiseA.then()
to create a chain of promises. This technique is known as "promise chaining." By calling .then()
on a promise, you can attach a callback function, which will be executed when the promise either resolves or rejects.
Interpreting the Example 💡
Let's take a closer look at the snippet provided in the question:
promiseB = promiseA.then(function(result) {
return result + 1;
});
// promiseB will be resolved immediately after promiseA is resolved and its value
// will be the result of promiseA incremented by 1
When promiseA
resolves, the success callback function specified within .then()
is called with the resolved value as an argument. In this case, the success callback takes in result
as the parameter. The function then returns result + 1
.
Accessing the Value of PromiseB 🎁
Now comes the exciting part! Since promiseB
is created by chaining .then()
to promiseA
, it is also a promise object. So, to access the resolved value of promiseB
, we need to use another .then()
.
promiseB.then(function(value) {
console.log(value); // Output: the value of promiseA incremented by 1
});
In this code snippet, the success callback function within .then()
receives the resolved value of promiseB
. In our example, this will be the result of promiseA
incremented by 1. You can access and use this value within the callback function.
Handling Errors 🚨
It's worth noting that promise chaining provides an elegant solution for error handling as well. By chaining a .catch()
after all the .then()
callbacks, you can catch and handle any errors that occur during the promise chain.
Putting It All Together 🤩
To summarize, you can access the value of a promise by using .then()
to chain promises together. Each .then()
call allows you to specify a success callback function, which receives the resolved value of the previous promise. By returning a value within the success callback, you can pass data along the chain.
Your Turn to Explore 🚀
Now that you have a better grasp on accessing the value of a promise, why not try experimenting with your own examples? Get creative and see how promise chaining can make your code more efficient and manageable!
Feel free to share your findings and any questions you might have in the comments section below. Let's keep the conversation going! 💬
🙌✨ Happy coding everyone! ✨🙌
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
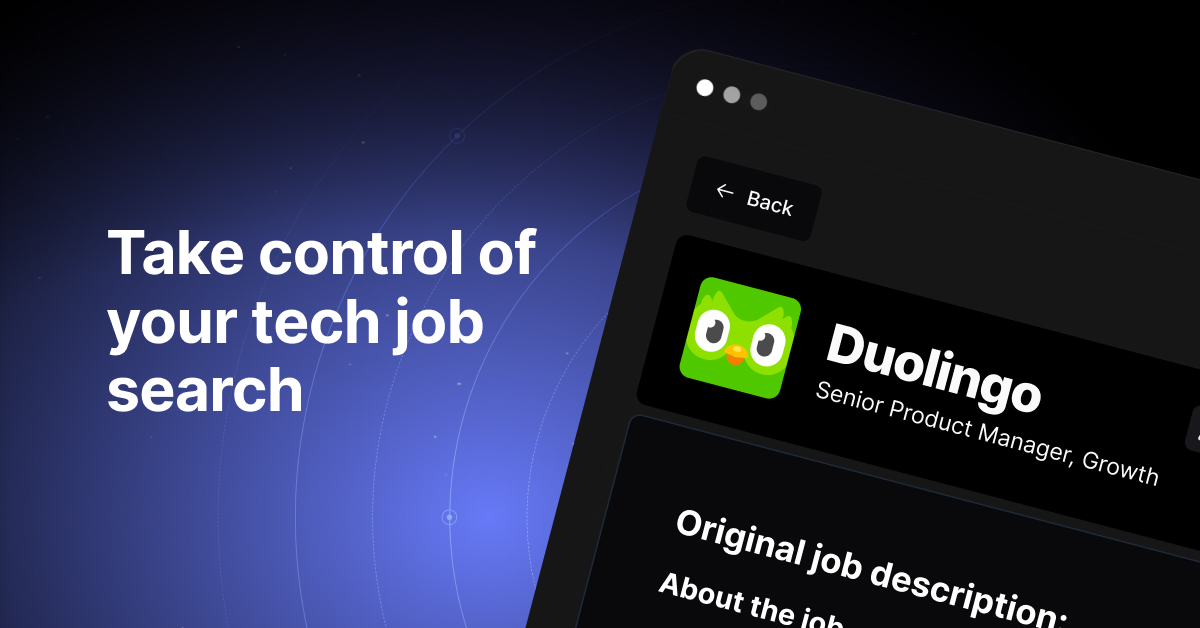