How can I access the contents of an iframe with JavaScript/jQuery?
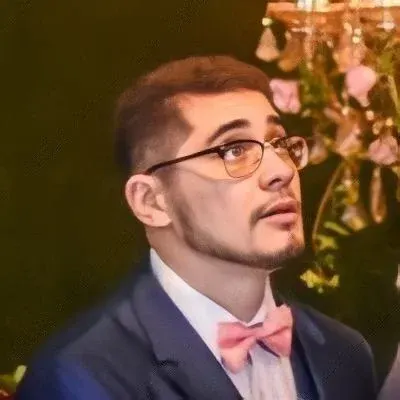

🌐🔍 Accessibility Issue: How to Access the Contents of an Iframe with JavaScript/jQuery?
Image from Unsplash
Hi there, tech enthusiasts! 👋
Ever come across a situation where you wanted to manipulate the HTML inside an iframe using JavaScript/jQuery? 🤔 Well, you're not alone! This question often trips many developers up.
Today, we'll dive into this common issue and provide some easy solutions to help you access the contents of an iframe effortlessly. So, let's get started! 🚀
The Challenge: Context and Permission Issues 🕵️♀️
The original question mentioned setting the context of a jQuery function to the document of an iframe. The approach looked promising, but it turned out that the variables in frames['nameOfMyIframe']
were undefined. 😮 On top of that, even after waiting for the iframe to load, permission errors prevented accessing the variables.
Solution 1: Wait Until the Iframe is Fully Loaded 🔄
To overcome the undefined
variables and permission errors, we need to ensure that the iframe has completed loading before accessing its contents. Here's a simple yet effective solution using vanilla JavaScript:
var iframe = document.getElementById('nameOfMyIframe');
iframe.onload = function() {
// Access the iframe contents here
var iframeDocument = iframe.contentDocument || iframe.contentWindow.document;
// Use iframeDocument to manipulate the HTML
};
By using the onload
event, we wait for the iframe to be fully loaded. Then, we can access its contents using either iframe.contentDocument
or iframe.contentWindow.document
.
Solution 2: Use jQuery to Simplify the Process 💡
If you prefer using jQuery, you can achieve the same result with a few modifications. Here's an example:
$(function() {
var iframe = $('#nameOfMyIframe')[0];
$(iframe).on('load', function() {
// Access the iframe contents here
var iframeDocument = iframe.contentDocument || iframe.contentWindow.document;
// Use iframeDocument to manipulate the HTML
});
});
In this code snippet, we use the jQuery functions $(function() {})
to ensure that the code runs when the document is ready. Then, we listen for the load
event on the iframe element and access its contents using the same logic as Solution 1.
Call to Action: Share Your Experience! 💬
Now that you have the solutions at your disposal, go ahead and try them out! Do you have any other methods for accessing iframe contents? Share your experience and help the coding community by leaving a comment below. Let's learn from one another! 🌟
That's a wrap for today's blog post! We hope you found it helpful and that it shed some light on this common issue. If you enjoyed this article, make sure to share it with your coding buddies or on your favorite social media platform. Spread the knowledge! 🙌
Thanks for reading, and happy coding! 😄💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
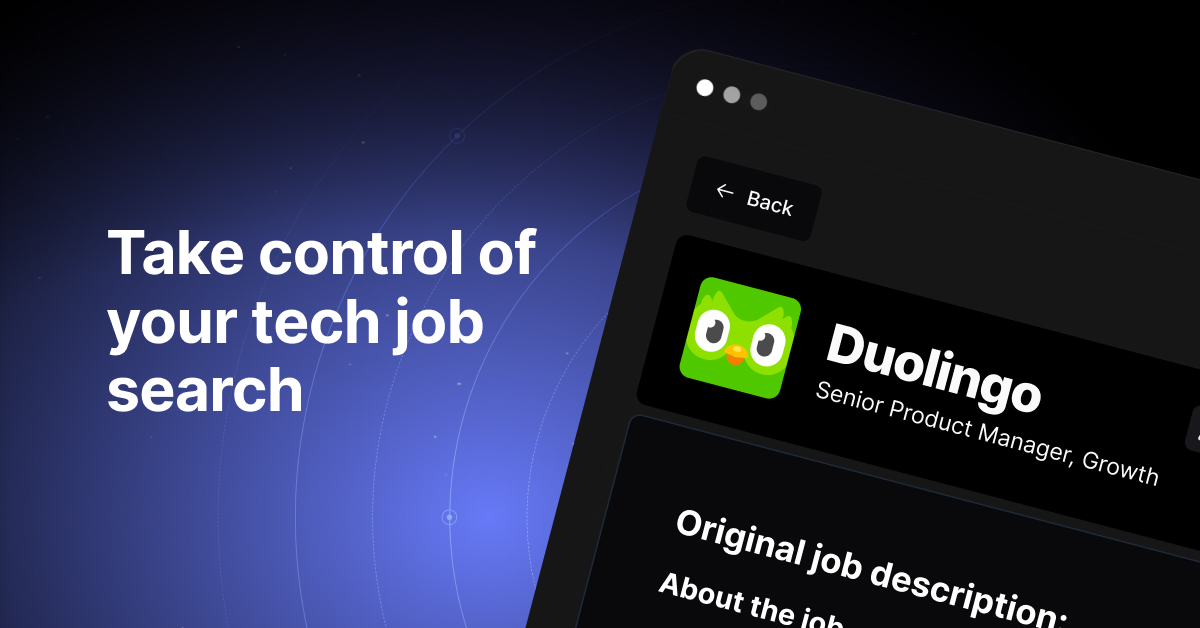