How can I access and process nested objects, arrays, or JSON?
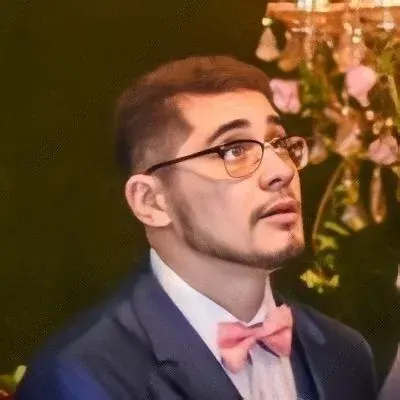
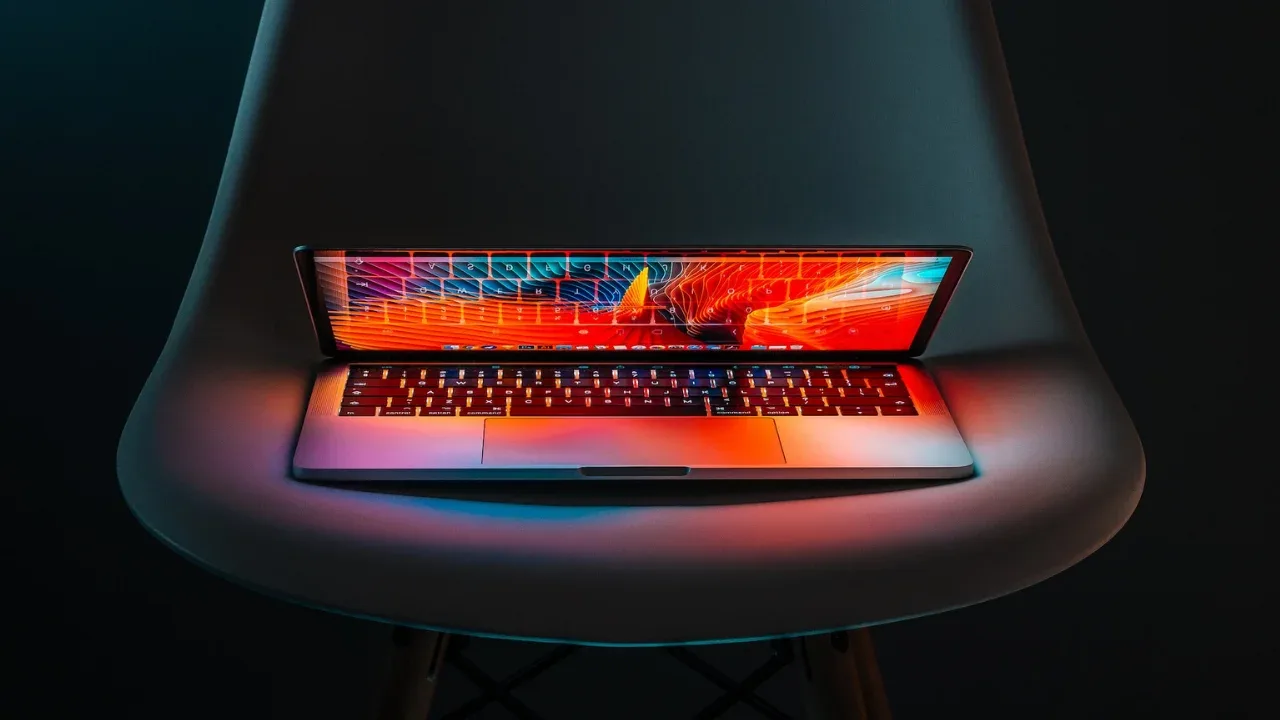
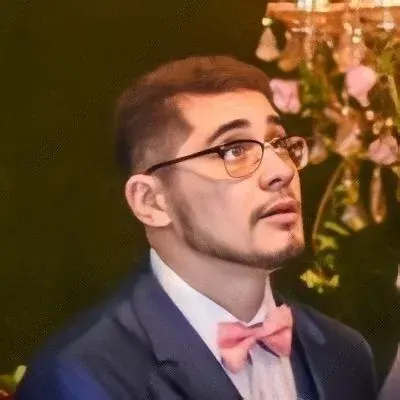
Accessing and Processing Nested Objects, Arrays, and JSON 🔄📂🔍
Have you ever found yourself struggling to extract specific values or keys from a nested data structure like objects within arrays or JSON? If so, you're not alone! Many developers face this common challenge when working with complex data structures. But fear not! In this blog post, we'll dive deep into the world of nested objects, arrays, and JSON, and come out with easy solutions to access and process them. Let's get started!
The Scenario 🌟
Imagine you have a nested data structure like this:
var data = {
code: 42,
items: [{
id: 1,
name: 'foo'
}, {
id: 2,
name: 'bar'
}]
};
You want to access the name
of the second item in the items
array. But how exactly can you do that? Let's find out!
Accessing Nested Objects 🧭
To access nested objects, you need to chain the dot notation or square brackets to navigate through each level. Here's how you can access the name
of the second item in the items
array:
var itemName = data.items[1].name;
console.log(itemName); // Outputs: 'bar'
In this example, we first access the items
array using data.items
. Then, we navigate to the second item in the array using [1]
(remember, array indices start from 0). Finally, we access the name
property using .name
. Voila! You have successfully accessed the desired value.
Processing Nested Arrays 🌌
Processing nested arrays involves a similar approach as with nested objects. You need to navigate through each level using square brackets. Let's say you want to iterate over all the items in the items
array and log their names:
data.items.forEach(function(item) {
console.log(item.name);
});
By using the forEach
method, we loop through each item in the items
array and access the name
property of each item. You can perform any desired operations within the loop, such as filtering, mapping, or transforming the array elements.
Handling JSON Data 🌐
JSON (JavaScript Object Notation) is widely used for data interchange. When working with JSON, you can use the JSON.parse()
method to convert a JSON string to a JavaScript object, enabling easier manipulation.
var jsonString = '{"name": "John", "age": 30, "city": "New York"}';
var jsonObj = JSON.parse(jsonString);
console.log(jsonObj.name); // Outputs: 'John'
In this example, we parse a JSON string using JSON.parse()
and assign the resulting JavaScript object to jsonObj
. Now, you can access the properties of the JSON object just like any other JavaScript object.
Problem Solved! 🎉
Congratulations! You've successfully learned how to access and process nested objects, arrays, and JSON data. Now, you can confidently tackle even the most complex data structures and extract the information you need. So go ahead and apply these techniques to your own projects!
If you have any further questions or want to share your own tips and tricks, feel free to leave a comment below. Happy coding! 💻💡
Visit our Tech Blog for more helpful guides and tutorials! Don't forget to share this post and spread the knowledge! 😄🔗🚀