Handle file download from ajax post
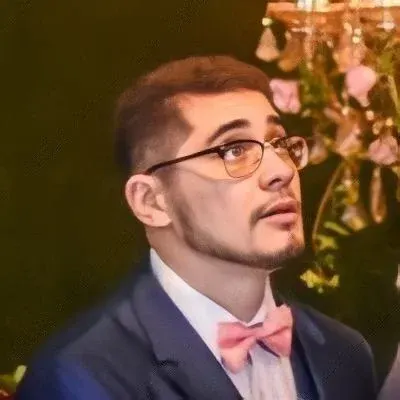
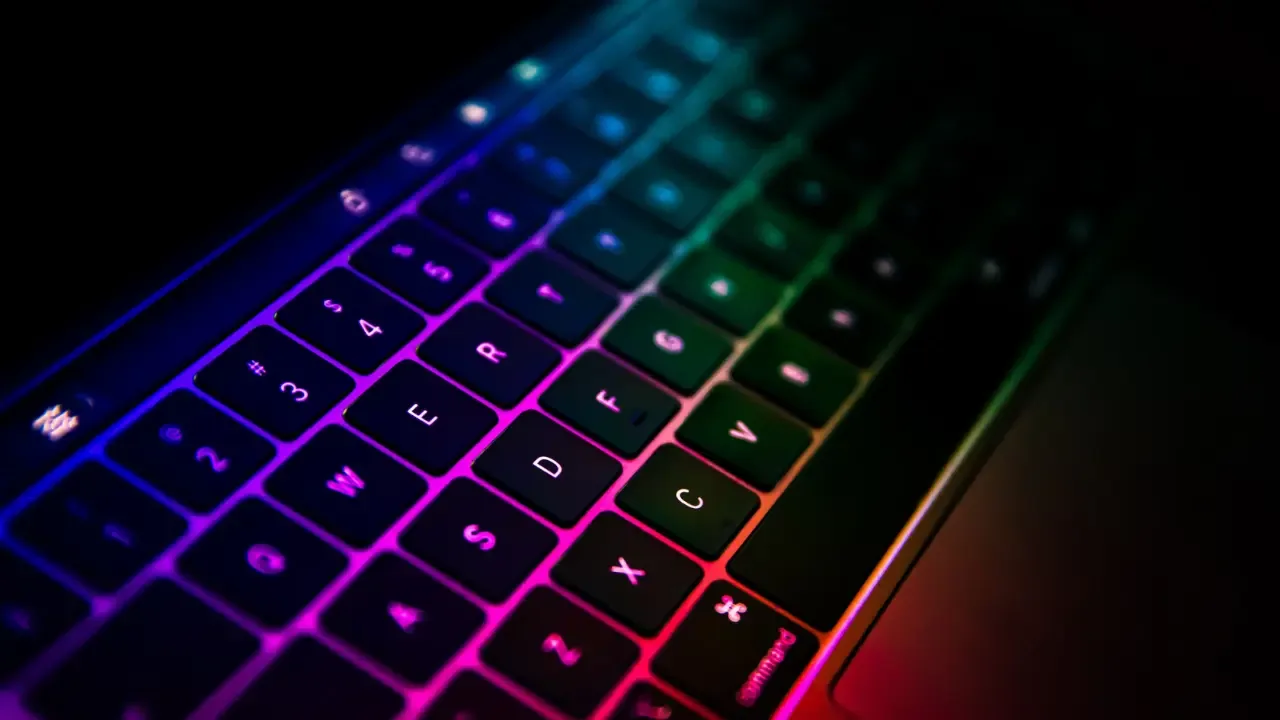
How to Handle File Download from Ajax Post
Are you struggling with handling file downloads from an Ajax POST request? Don't worry, you're not alone! Many developers face challenges when it comes to detecting a file in an Ajax response and offering the client a download dialog. In this blog post, we'll explore common issues and provide easy solutions to help you solve this problem efficiently.
The Challenge
Let's start by understanding the challenge you're facing. You have a JavaScript app that sends Ajax POST requests to a specific URL. The response you receive might be a JSON string or a file attachment. While you can easily detect the Content-Type and Content-Disposition in your Ajax call, you're unsure how to handle the file download and provide the client with a download dialog.
The Limitations
Before we dive into the solutions, it's important to address some limitations you've mentioned. You don't want answers suggesting not to use Ajax or to redirect the browser. Additionally, using a plain HTML form is not an option for you. We'll keep these constraints in mind while exploring the solutions.
Solution 1: Using the window.location.href
One straightforward solution is to use JavaScript's window.location.href
to download the file. Here's how you can achieve this:
$.ajax({
url: 'your-url',
type: 'POST',
success: function(response, status, xhr) {
var contentType = xhr.getResponseHeader("Content-Type");
if (contentType === "application/octet-stream") {
var fileName = xhr.getResponseHeader("Content-Disposition").split('=')[1];
var downloadUrl = URL.createObjectURL(response);
var a = document.createElement("a");
a.href = downloadUrl;
a.download = fileName;
document.body.appendChild(a);
a.click();
}
}
});
In this solution, we first check if the Content-Type
of the response is application/octet-stream
. If it is, we extract the file name from the Content-Disposition
header and create a temporary download URL using URL.createObjectURL()
. We then create an <a>
element with the appropriate href
and download
attributes, append it to the body, and simulate a click to trigger the file download.
Solution 2: Using Fetch API
Another solution is to use the Fetch API, which provides a more modern and concise way to handle file downloads. Here's an example:
fetch('your-url', {
method: 'POST',
mode: 'cors',
})
.then(function(response) {
var disposition = response.headers.get('Content-Disposition');
if (disposition && disposition.indexOf('attachment') !== -1) {
return response.blob();
} else {
throw new Error('File download not found!');
}
})
.then(function(blob) {
var fileName = disposition.split('=')[1];
var downloadUrl = URL.createObjectURL(blob);
var a = document.createElement("a");
a.href = downloadUrl;
a.download = fileName;
document.body.appendChild(a);
a.click();
})
.catch(function(error) {
console.log('Error:', error);
});
In this solution, we fetch the URL using the Fetch API and then check if the Content-Disposition
header contains the word "attachment". If it does, we extract the file name and create a temporary download URL. The rest of the process is the same as in Solution 1.
Conclusion
Handling file downloads from Ajax POST requests can be challenging, but with the right solutions, you can offer a seamless download experience to your clients. In this blog post, we explored two possible solutions using window.location.href
and the Fetch API. Give them a try and let us know which one works best for you!
Have you faced similar challenges in your web development journey? How did you overcome them? Share your thoughts and experiences in the comments below. Let's learn and grow together!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
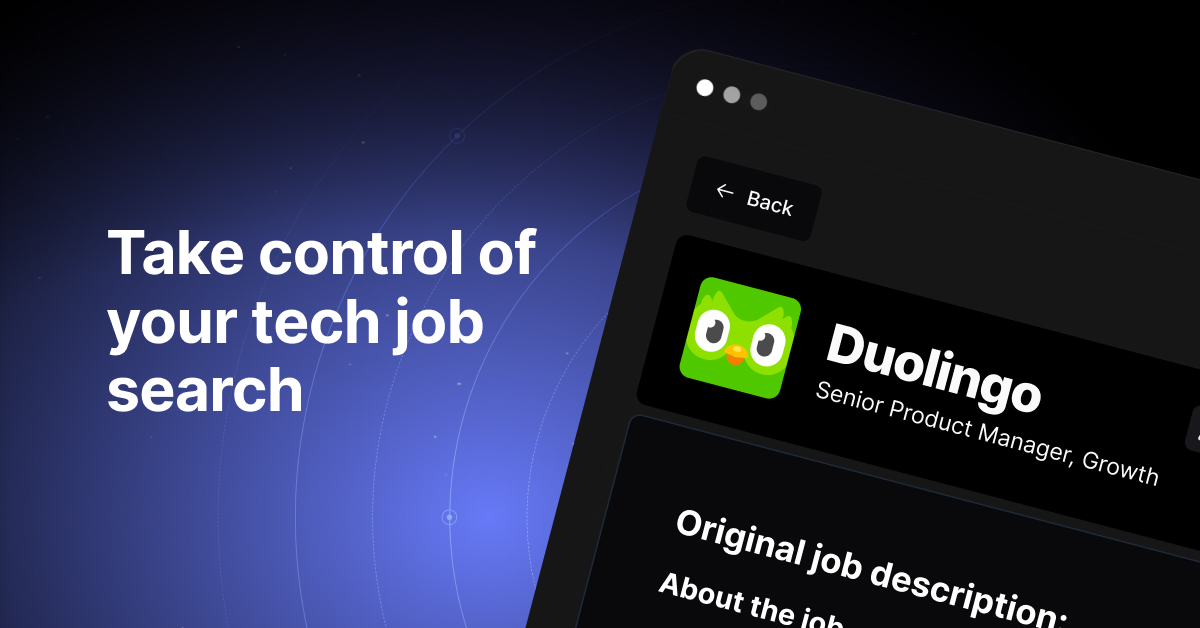