Getting the ID of the element that fired an event
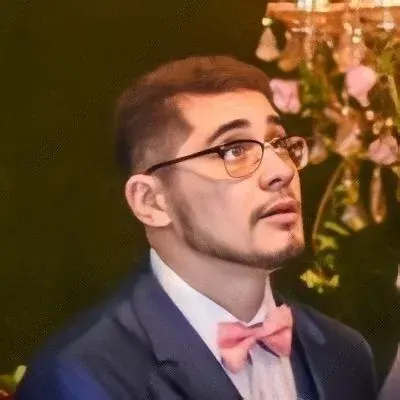
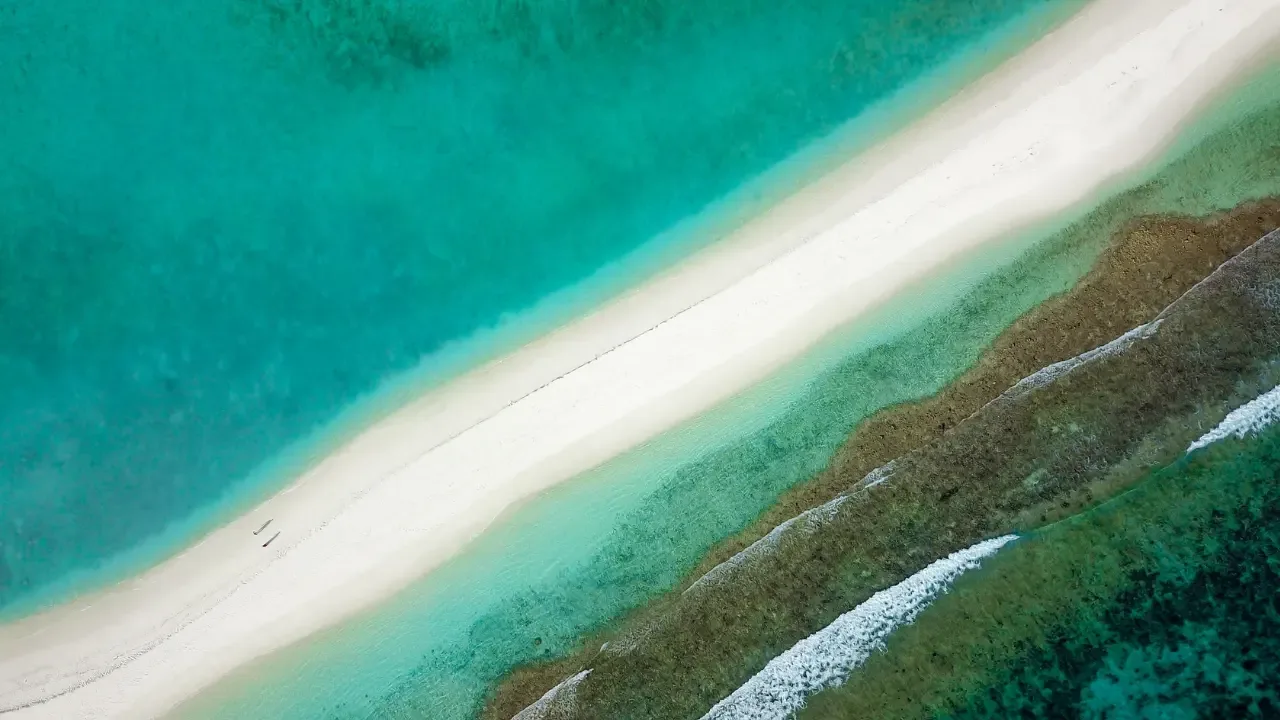
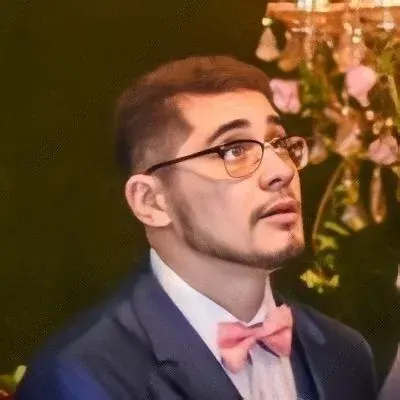
Getting the ID of the Element that Fired an Event 💥
Have you ever needed to find the ID of the element that triggered an event in JavaScript? 🤔 It can be tricky to figure out, but don't worry, we've got you covered! In this blog post, we'll address this common issue and provide you with easy solutions that will make your life easier. Let's dive in and find out how to get that elusive element ID! 🚀
The Problem 🕵️♀️
So, you're working on your project and you need to grab the ID of an element that has fired an event. Maybe you want to perform some specific logic based on the ID, or maybe you just want to get some information about the element. Whatever the reason, it's not always straightforward to determine the ID of the element that triggered the event.
In the given context, it seems like the goal is to obtain the ID of the element that was clicked within a click
event handler. Let's see what the code snippet looks like:
<script type="text/javascript" src="starterkit/jquery.js"></script>
<form class="item" id="aaa">
<input class="title"></input>
</form>
<form class="item" id="bbb">
<input class="title"></input>
</form>
<script>
$(document).ready(function() {
$("a").click(function() {
var test = caller.id;
alert(test.val());
});
});
</script>
In this example, we have two forms with IDs "aaa" and "bbb" respectively. When a link within the document is clicked, we want to display the ID of the form from which the event originated.
The Solution 💡
To solve this problem, we need to access the ID of the element that triggered the event within the event handler function. Unfortunately, there is no built-in property like caller.id
that gives us access to the ID directly. However, we can use the event object to extract the necessary information.
Here's an updated version of the code snippet that will correctly alert the ID of the clicked form:
$(document).ready(function() {
$("form").click(function(event) { // Changed from 'a' to 'form'
var test = event.target.id;
alert(test);
});
});
In this solution, we modify the event handler to listen for clicks on form
elements instead of a
elements (as per the original code snippet). Then, within the event handler function, we access the ID of the event target using event.target.id
. This will give us the ID of the element that was clicked.
Now, when you click on the first form, it will alert "aaa", and when you click on the second form, it will alert "bbb".
Conclusion 🎉
Getting the ID of the element that fired an event might seem like a challenging task, but with the help of the event object, we can easily access the necessary information. Remember to use event.target.id
to retrieve the ID of the element within the event handler function.
Hopefully, this blog post has helped you solve the problem you were facing and provided you with a clear understanding of how to get the ID of the element that triggered the event. Now you can confidently handle IDs and create some awesome dynamic interactions on your web projects! 🙌
If you have any questions or other interesting insights to share, feel free to leave a comment below. We'd love to hear from you!
Happy coding! 💻✨