Getting all selected checkboxes in an array
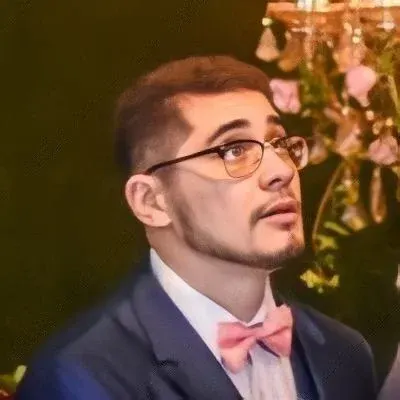

š Tech Blog Post: A Simple Solution to Get All Selected Checkboxes in an Array!
š Hey, fellow coders! š„ļø Are you struggling to get all the selected checkboxes in an array using JavaScript for your AJAX requests? š¤ Don't worry, I've got your back! In this blog post, we will address this common issue and provide you with an easy-peasy solution. Let's dive in! š¦
š§© Understanding the Problem
So, you have a bunch of checkboxes in your HTML code, just like this:
<input type="checkbox" name="type" value="4" />
<input type="checkbox" name="type" value="3" />
<input type="checkbox" name="type" value="1" />
<input type="checkbox" name="type" value="5" />
Your goal is to extract the values of all the selected checkboxes and store them in an array. This way, you can conveniently use them in your AJAX $.post
request, powered by jQuery, to send the selected checkbox values to the server. šŖ
š” Easy Solution: Using JavaScript and jQuery
To achieve this, let's follow these simple steps:
First, we need to ensure that our JavaScript code executes after the DOM is fully loaded. Wrap your code inside the
$(document).ready()
function provided by jQuery.Next, let's select all the checkboxes using a CSS selector and attach an event listener to the checkboxes to detect any change in their checked state. Here's an example code snippet:
// Wait for the DOM to be ready $(document).ready(function() { // Select all the checkboxes with the name "type" using the attribute selector $('input[name="type"]').on('change', function() { // Your code to handle the checkbox change event }); });
Inside the event listener, we can use the jQuery
:checked
selector to only consider the checkboxes that are currently checked. We will then iterate over these selected checkboxes and extract their values into an array. Here's the updated code:// Wait for the DOM to be ready $(document).ready(function() { // Select all the checkboxes with the name "type" using the attribute selector $('input[name="type"]').on('change', function() { // Create an empty array to store the selected checkbox values var selectedCheckboxes = []; // Iterate over the selected checkboxes and extract their values $('input[name="type"]:checked').each(function() { selectedCheckboxes.push($(this).val()); }); // Do whatever you want with the selected checkbox values console.log(selectedCheckboxes); }); });
Finally, within the code block where we handle the selected checkbox values, you can customize the code to suit your specific needs. For example, you can make an AJAX
$.post
request using jQuery to send the selected checkbox values to the server. š
š£ Your Call-to-Action: Engage & Share!
There you have it! A simple, yet effective solution to get all the selected checkboxes in an array using JavaScript and jQuery for your AJAX requests. š
I hope this guide has helped you solve your problem effortlessly. If you found this blog post helpful, don't be shy! Share it with your coding buddies and spread the knowledge. Let's help everyone streamline their checkbox handling! š
š„ Feel free to leave a comment and discuss any related issues or alternative solutions you've come across. Your thoughts and experiences matter! Let's grow together as a community. š±
Happy coding! š»āØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
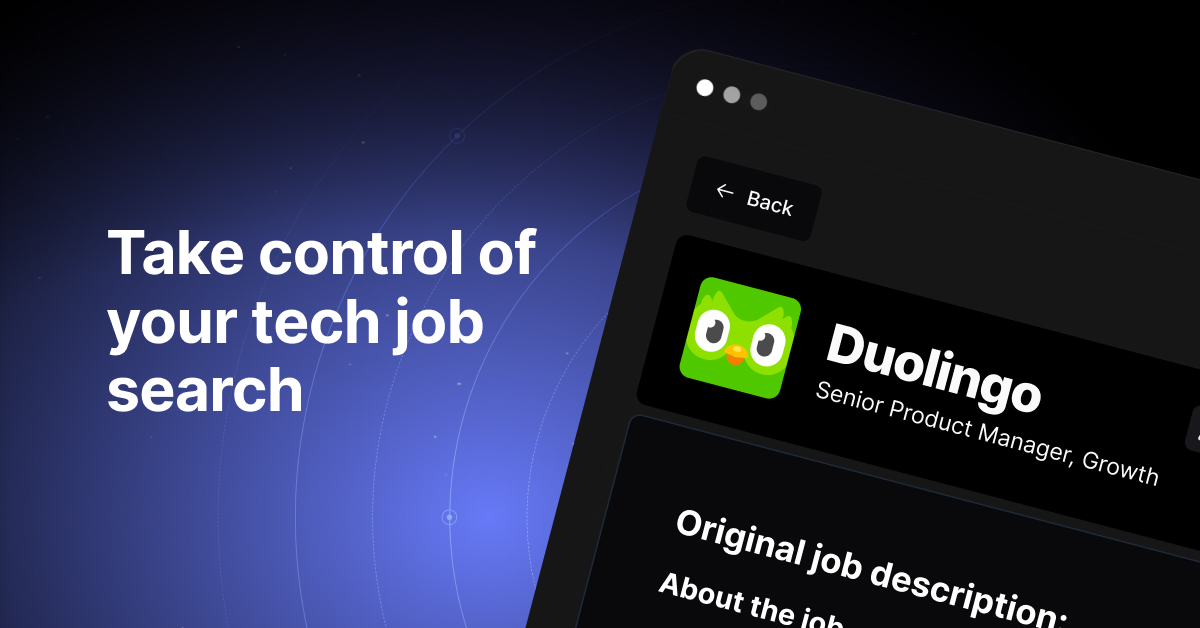