Get viewport/window height in ReactJS
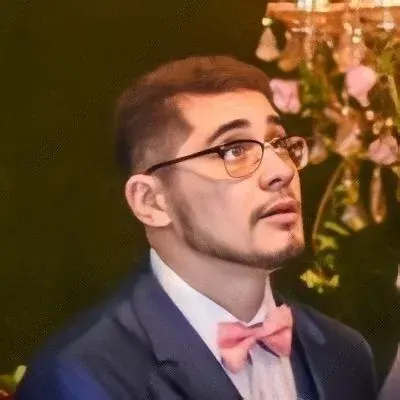
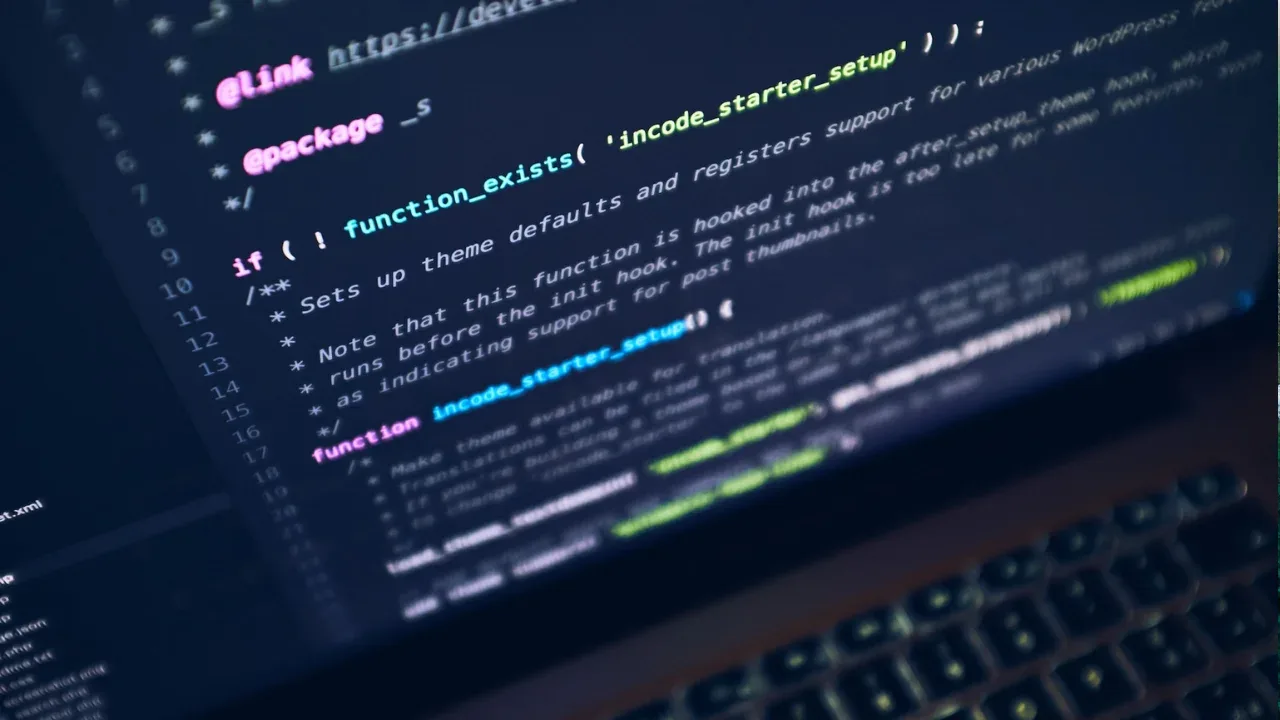
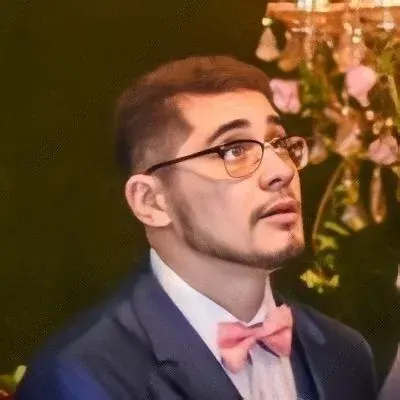
📱 How to Get Viewport/Window Height in ReactJS
Have you ever wondered how to get the viewport height in ReactJS? 🤔 It's a common question, especially when you want to dynamically adjust the layout or elements based on the available space. In this blog post, I'll walk you through the process and provide easy solutions to get the window height in ReactJS. Let's dive in! 💪
🧐 The Problem
Traditionally, in vanilla JavaScript, we can easily use window.innerHeight
to get the viewport height. However, things work a bit differently in ReactJS. If you try to use window.innerHeight
directly in your React component, you might run into some issues. 😓
window.innerHeight; // This won't work in ReactJS
💡 The Solution
To get the viewport height in ReactJS, we can leverage the power of the React ecosystem and utilize the useEffect
hook and the window
object. Here's a step-by-step solution:
Import the necessary dependencies:
import React, { useEffect, useState } from 'react';
Create a new functional component, let's call it
ViewportHeight
:
const ViewportHeight = () => {
const [height, setHeight] = useState(window.innerHeight);
useEffect(() => {
const handleResize = () => {
setHeight(window.innerHeight);
};
window.addEventListener('resize', handleResize);
// Clean up the event listener on component unmount
return () => {
window.removeEventListener('resize', handleResize);
};
}, []);
return <div>Viewport height: {height}px</div>;
};
export default ViewportHeight;
Use the
ViewportHeight
component anywhere in your project:
import React from 'react';
import ViewportHeight from './ViewportHeight';
const App = () => {
return (
<div>
<h1>Welcome to My Awesome App</h1>
<ViewportHeight />
{/* Add more components here */}
</div>
);
};
export default App;
That's it! 🎉 With this solution, the ViewportHeight
component will dynamically update the height whenever the window is resized. Isn't that cool? 😎
📝 Explaining the Solution
Let's break down the solution step by step:
We import the necessary dependencies, including
React
,useEffect
, anduseState
.Inside the
ViewportHeight
component, we create a state variableheight
using theuseState
hook. We initialize it with the initial value ofwindow.innerHeight
.We use the
useEffect
hook to add an event listener to theresize
event on thewindow
object. Whenever the window is resized, thehandleResize
function will be called, updating theheight
state variable.To prevent memory leaks, we clean up the event listener on component unmount by returning a cleanup function from the
useEffect
hook.Finally, we render the
height
value in the JSX of theViewportHeight
component, wrapped in a<div>
element.
By following these steps, you can easily get the viewport height in ReactJS and keep it in sync with window resizes. Cool, right? 😄
🔥 Get Creative!
Now that you know how to get the viewport height in ReactJS, you can unleash your creativity and build amazing user interfaces that adapt to different screen sizes. 🚀
📣 Your Turn!
I hope this guide was helpful and easy to understand! Now, I encourage you to try it out yourself and let me know how it goes. Have you encountered any challenges with getting the viewport height in ReactJS? What creative solutions have you come up with? Share your experiences and thoughts in the comments section below! Let's learn from each other. 👇
Happy coding! 💻✨