Get the last item in an array
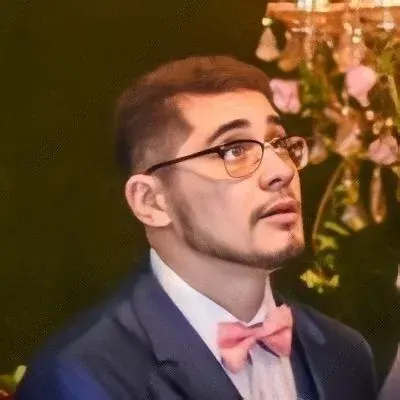
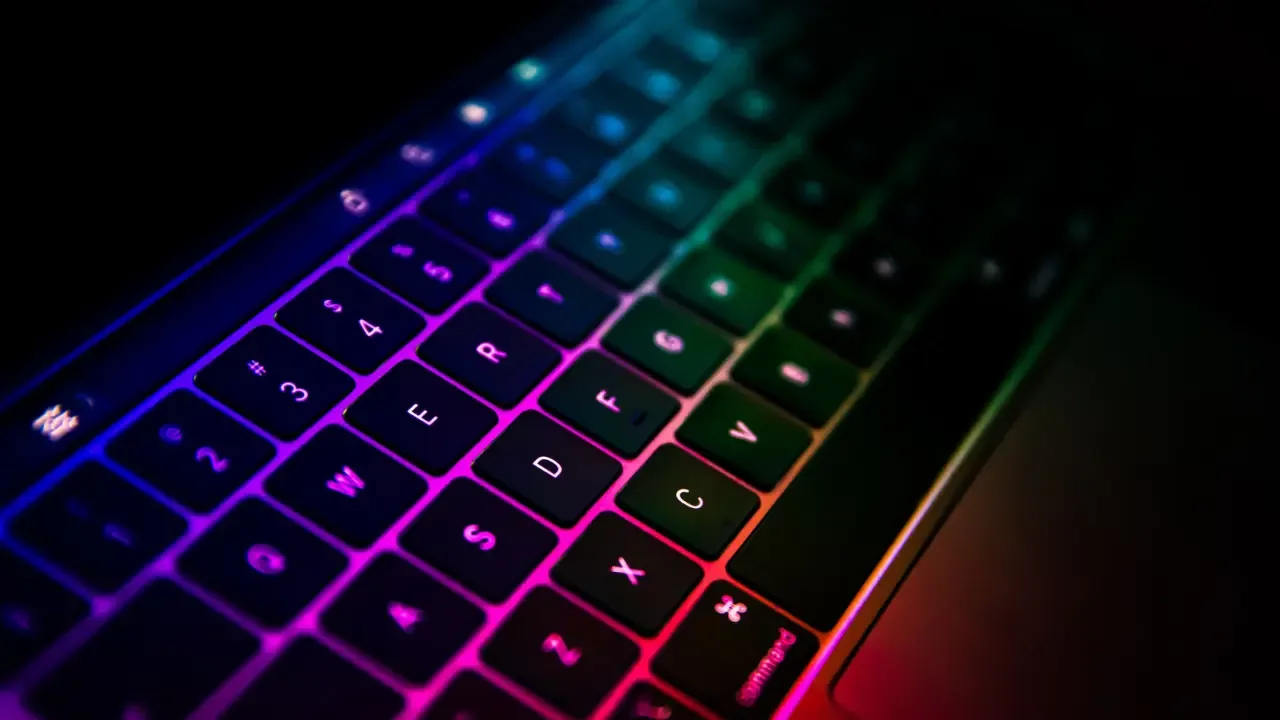
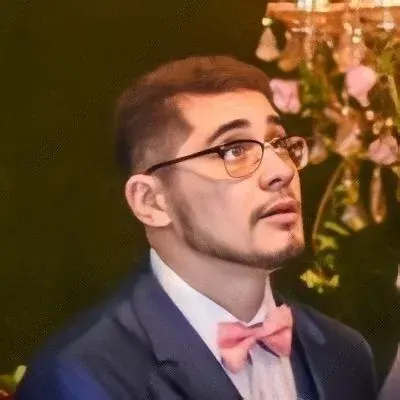
📝 The Ultimate Guide to Getting the Last Item in an Array in JavaScript 🚀
So, you're working on some JavaScript code and you need to get the last item in an array. But wait, there's a twist! You want to check if the last item is "index.html"
, and if it is, you want to grab the third to last item instead. Sounds tricky, but fear not! In this guide, we'll walk through the common issues, provide easy solutions, and empower you to conquer this problem. Let's dive in! 💪
🔄 Common Issues and Specific Problem
Based on the provided context, the code is already grabbing the second to last item in the loc_array
. However, you need to add a check for the last item to be "index.html"
before retrieving the third to last item. Here are a couple of things to keep in mind:
Array Indexing: JavaScript arrays are zero-indexed, meaning the first item is at index
0
, the second item is at index1
, and so on.Checking Array Length: To determine the last item in an array, you can subtract
1
from the array's length.
✅ Easy Solutions
Now, let's explore a couple of possible solutions to accomplish the desired outcome. Below, you'll find a modified version of the provided code with an added check. Take a look:
var linkElement = document.getElementById("BackButton");
var loc_array = document.location.href.split('/');
var lastItemIndex = loc_array.length - 1;
var thirdToLastItemIndex = lastItemIndex - 2;
if (loc_array[lastItemIndex] === "index.html") {
var newT = document.createTextNode(unescape(capWords(loc_array[thirdToLastItemIndex])));
} else {
var newT = document.createTextNode(unescape(capWords(loc_array[lastItemIndex])));
}
linkElement.appendChild(newT);
In the above code snippet, we:
Store the last item's index in the
loc_array
aslastItemIndex
.Calculate the index of the third to last item as
thirdToLastItemIndex
by subtracting2
fromlastItemIndex
.Add a conditional statement to check if the last item is
"index.html"
.If the condition is met, we grab the third to last item; otherwise, we grab the last item as before.
📣 Compelling Call-to-Action
Now that you have shiny new code to get the last item in an array, it's time to put it into action! Try implementing it in your project and see if it solves your problem. Remember, always test and debug your code to ensure it works as expected.
If you found this guide helpful, don't forget to share it with your fellow developers to save them from array woes! Also, feel free to drop a comment below with your thoughts, suggestions, or any other tricky coding problems you'd like us to cover in future blog posts. Keep coding and unleashing your creativity! 🎉