Get the index of the object inside an array, matching a condition
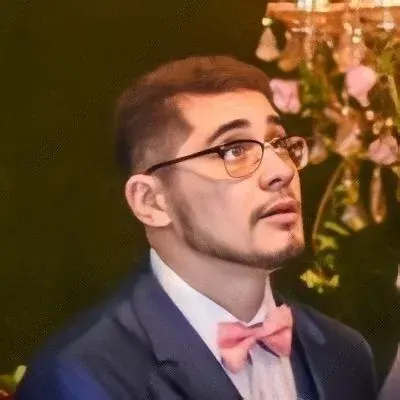
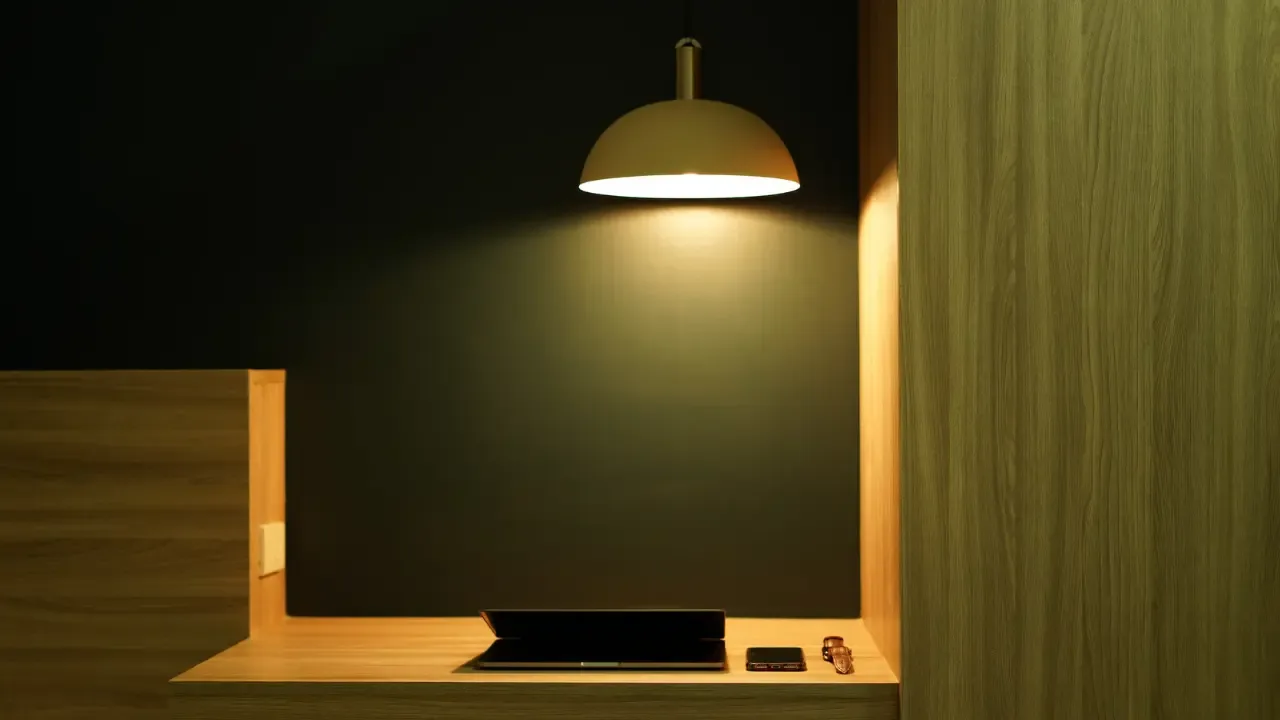
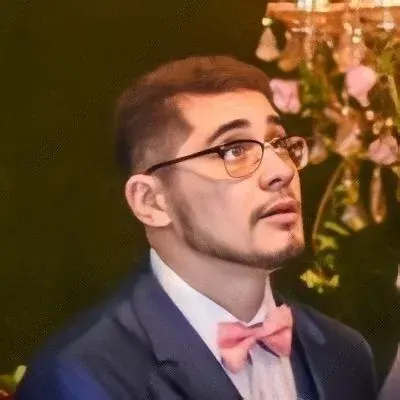
📝 Tech Blog: Easy Ways to Get the Index of an Object Matching a Condition in an Array 🤔
Are you tired of iterating over entire arrays just to find the index of an object that matches a specific condition? Look no further! In this blog post, we will explore easy solutions to this problem and save you precious time. Let's dive in! 💪
The Problem: Finding the Index without Iterating 🚀
So, you have an array of objects and you need to find the index of the object that matches a given condition. Let's say you have an array like this:
[{ prop1: "abc", prop2: "qwe" },
{ prop1: "bnmb", prop2: "yutu" },
{ prop1: "zxvz", prop2: "qwrq" }, ...]
You want to find the index of the object where prop2
is equal to "yutu"
. In this case, the expected output is 1
.
The Solution: Using Array.prototype.findIndex() 😎
You mentioned you came across the .indexOf()
method, but it only works for simple arrays like ["a1","a2",...]
and not nested objects. No worries! There is another powerful method called findIndex()
specifically designed to address this problem.
Here's how you can use it to get the index of the object that matches your condition:
const myArray = [
{ prop1: "abc", prop2: "qwe" },
{ prop1: "bnmb", prop2: "yutu" },
{ prop1: "zxvz", prop2: "qwrq" }, ...];
const index = myArray.findIndex(obj => obj.prop2 === "yutu");
console.log(index); // Output: 1
By using findIndex()
along with an arrow function, we can easily compare the prop2
value of each object in the array with our desired condition. Once a match is found, the index of that object is returned 🎉
Going the Extra Mile: Handling No Matches 🏁
Now, what if there are no objects in the array that match the given condition? Don't worry, JavaScript has got you covered! The findIndex()
method returns -1 if no match is found. You can use this knowledge to handle such scenarios in your code:
const index = myArray.findIndex(obj => obj.prop2 === "nonexistent");
if (index === -1) {
console.log("No object found matching the given condition.");
} else {
console.log(index);
}
This way, you can gracefully handle cases where no matching object is found and prevent any unexpected behavior.
Your Turn: Try it Out! 🚀
Now that you have learned an easy and efficient way to find the index of an object matching a condition, it's time to put it into practice! Pick a project, find a use case, and implement this handy solution. Share your experience in the comments below – we want to hear from you! ✨
That's all for this blog post! We hope you enjoyed and found it helpful. If you have any questions or suggestions, feel free to reach out. Happy coding! 😄✌️