Get the current year in JavaScript
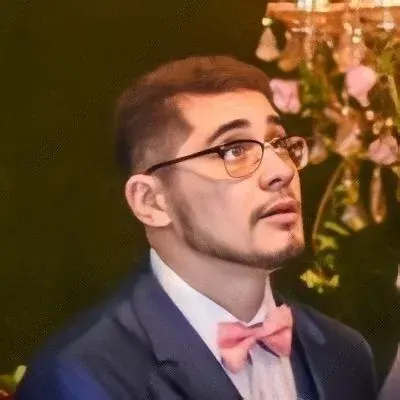
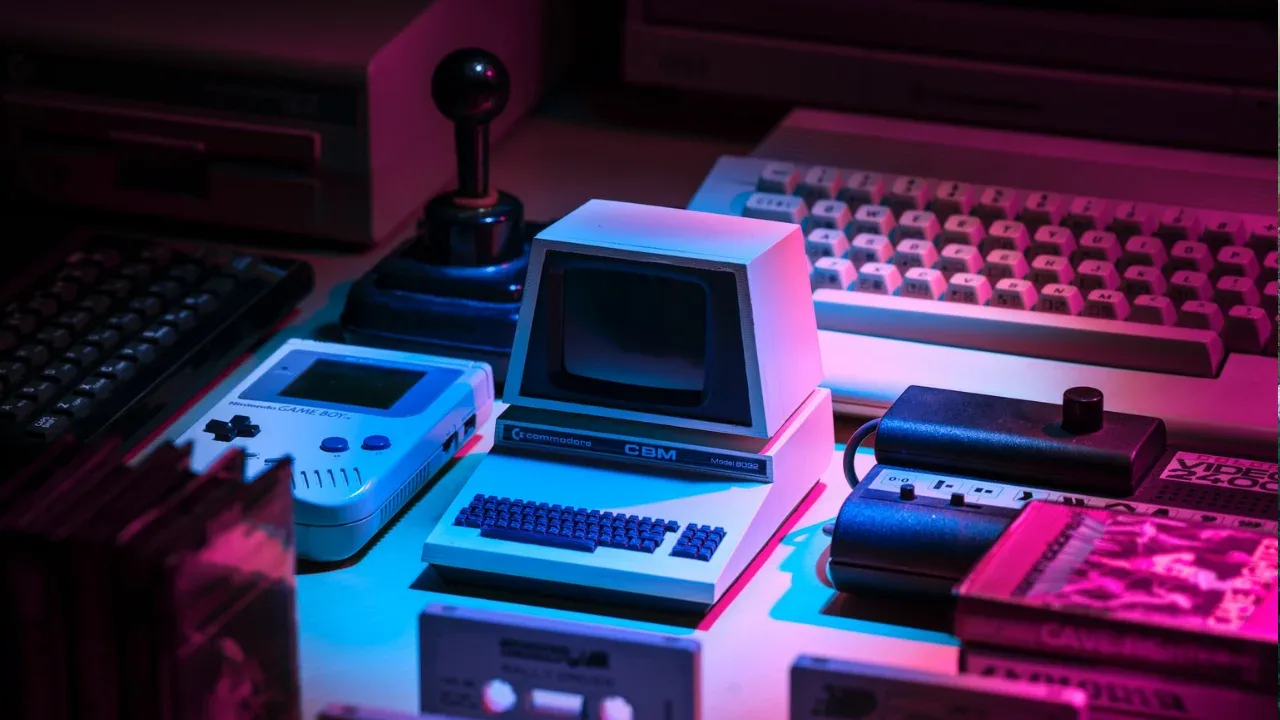
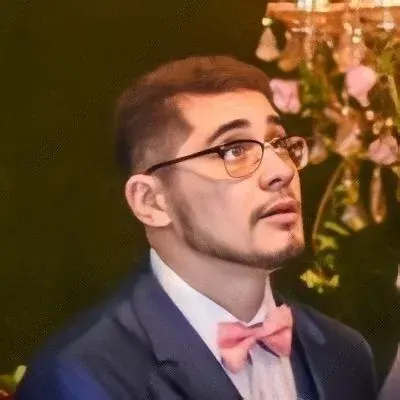
📝 🌟 Get the Current Year in JavaScript Like a Pro 🌟
Have you ever found yourself in need of the current year in your JavaScript code? 🤔 No worries, we've got you covered! In this blog post, we'll dive deep into this common question and equip you with easy solutions to fetch the current year effortlessly. 🚀
The Common Problem 🤷♀️
Before we start unraveling the solution, let's understand the underlying problem. Suppose you have a fancy website where you want to display the copyright year dynamically. Instead of manually updating it each year, wouldn't it be awesome if it could auto-update itself? 🤩 Here's where getting the current year in JavaScript becomes crucial!
Solution: The Date Object 📅
JavaScript provides the Date object, which is a built-in tool packed with tons of useful methods and properties. It allows you to work with dates, including fetching the current year.
Here's a simple code snippet that demonstrates how to fetch the current year using the Date object:
const currentDate = new Date();
const currentYear = currentDate.getFullYear();
console.log(currentYear);
In the above code, we create a new instance of the Date object called currentDate
. Then, we use the getFullYear()
method to extract the current year from currentDate
. Finally, we store the current year in the currentYear
variable and log it to the console. 🖥️
Run this code in your favorite console, and voila! 🎉 You'll see the current year displayed!
🤯 To UTC or Not? That's the Question
Now, let's dive into a slightly advanced topic. When working with the Date object, you have two options: local time or UTC (Coordinated Universal Time). By default, the getFullYear()
method returns the local year. But what if you want to obtain the UTC year instead? Easy-peasy! You just need to use the getUTCFullYear()
method instead.
const currentDate = new Date();
const currentYear = currentDate.getUTCFullYear();
console.log(currentYear);
Look at that! With a simple tweak, you can get the UTC year without breaking a sweat! ⏱️
🎉 Call-to-Action: Share Your Solutions! 💡
Now that you know how to get the current year in JavaScript, it's time to put your knowledge to the test! Feel free to experiment and explore different ways to tackle this challenge. Maybe you'll come up with a clever one-liner or discover an alternative approach. Whatever it is, we'd love to hear about it!
Head over to the comments section below and share your insights, solutions, or even questions. Let's spark a conversation and help each other become JavaScript masters! 👩💻👨💻
Stay tuned for more amazing JavaScript tips and tricks. Happy coding! 💻🚀