Get the current URL with JavaScript?
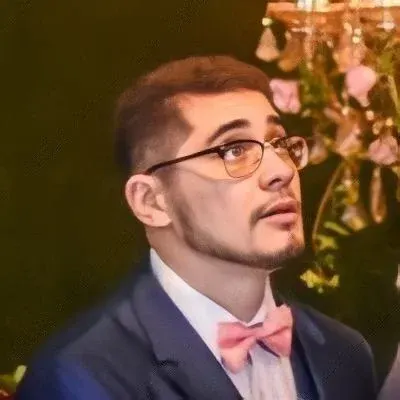
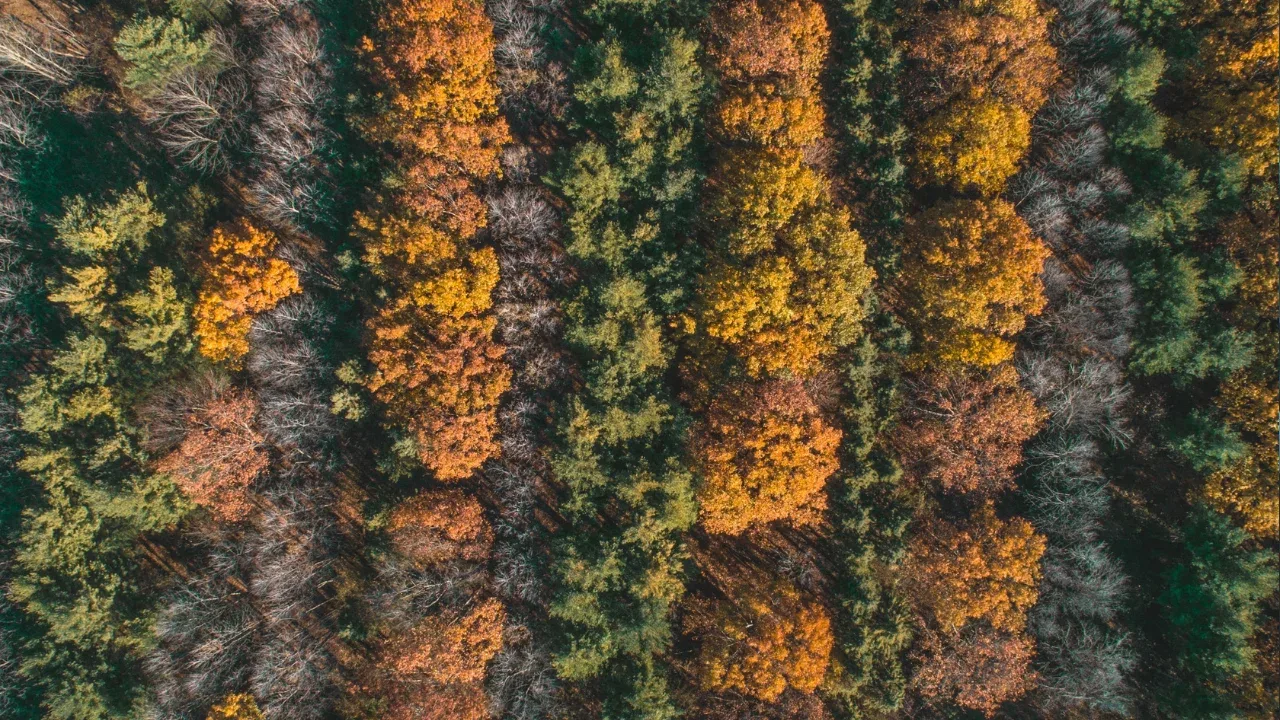
🌐 Get the current URL with JavaScript: A Simple Guide
Are you trying to fetch the current URL of your website using JavaScript? Look no further! In this guide, we will walk you through the process of getting the full URL, without relying on any links. Whether you're a beginner or an experienced developer, we've got you covered. Let's dive in! 🏊♂️
💡 The Problem
As mentioned in the context, you want to retrieve the full, current URL of your website. This can be useful for various purposes, such as tracking, analytics, or dynamically changing content based on the URL. However, it might not be immediately apparent how to tackle this challenge. Don't worry, it's simpler than you think! 🤓
🛠️ The Solution
JavaScript provides a handy property called window.location
that contains information about the current URL. To obtain the full URL, you can access the href
property of window.location
. Here's how you can do it:
const currentURL = window.location.href;
console.log(currentURL);
That's it! 🎉 By logging currentURL
to the console, you'll see the complete URL of your website. Feel free to use this variable as needed in your code.
But wait, there's more! Did you know that window.location
also contains other useful information about the URL? Let's explore a couple of examples:
If you only require the protocol (e.g., "https:"), you can access the
protocol
property:
const protocol = window.location.protocol;
console.log(protocol);
If you need the domain or host (e.g., "www.example.com"), you can access the
hostname
property:
const domain = window.location.hostname;
console.log(domain);
📣 Get Creative!
Now that you know how to obtain the current URL, the possibilities are endless! Here are a few ideas to inspire you:
Dynamically update page content based on different parts of the URL.
Generate customized sharing links including the current URL.
Track user navigation patterns and behavior using analytics tools.
Feel free to experiment and let your creativity shine! 🌈💡
🤝 Join the Discussion
We hope this guide helped you fetch the current URL using JavaScript. If you have any questions, suggestions, or cool use cases, we'd love to hear from you! Leave a comment below and let's start a conversation. Your insights might inspire others in the community. 👥💬
Happy coding! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
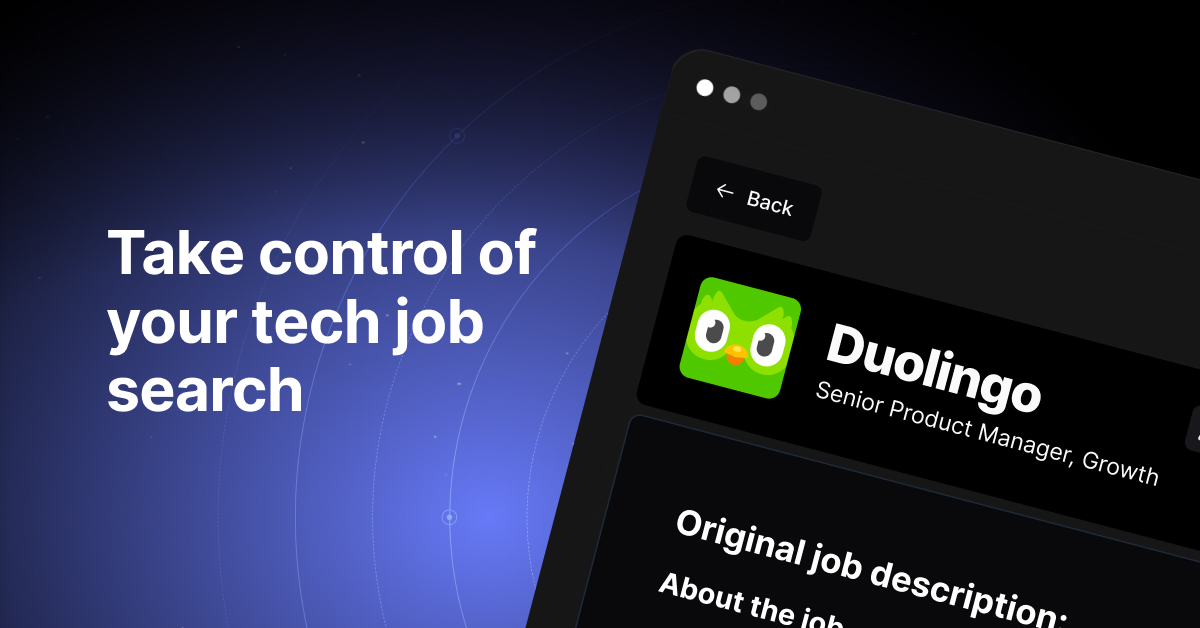