Get Substring between two characters using javascript
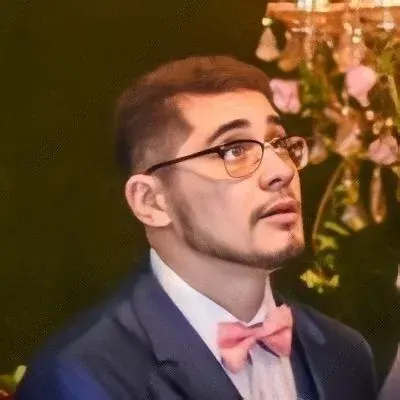
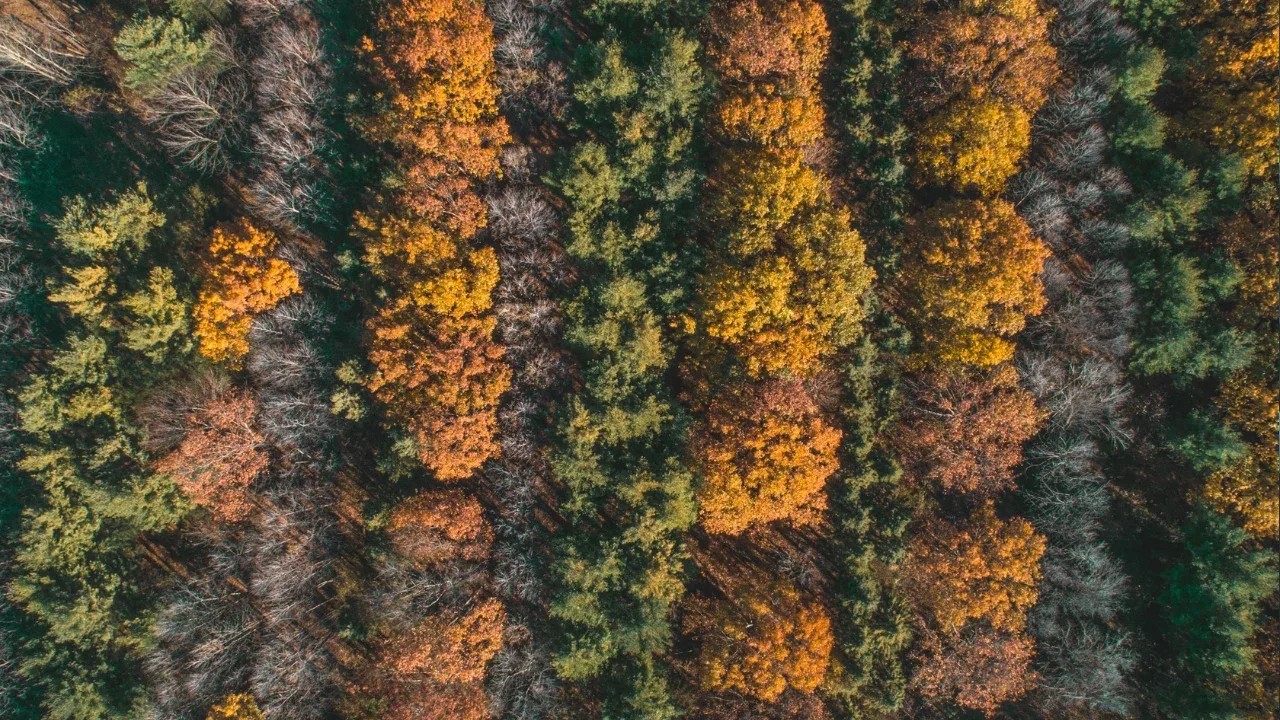
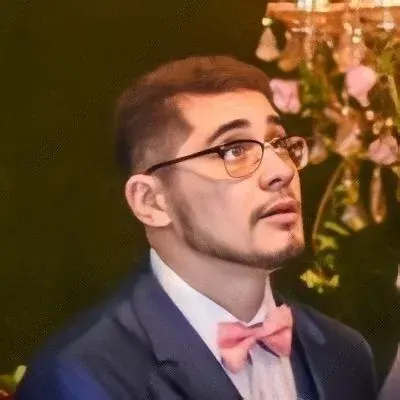
Get Substring between two characters using JavaScript: An Easy Solution
Are you struggling to extract a substring from a larger string? Specifically, are you trying to get everything between two specific characters, like ":" and ";"? We've got just the solution for you! In this blog post, we'll provide an easy and straightforward approach to achieve the desired output using JavaScript. So let's dive right in!
The Problem: Extracting a Substring
Here's the context of the problem: you have a long string, and you want to extract a specific substring from within that string. In your case, you're looking to extract the substring between a ":" and a ";".
Here's an example:
const str = 'MyLongString:StringIWant;';
Your desired output is to extract the substring "StringIWant".
The Solution: Using the substring and indexOf Methods
To extract the substring between the two characters, we can utilize the substring
and indexOf
methods in JavaScript. Here's the step-by-step process:
Step 1: Find the index of the first character (":" in this case). To do this, we can use the indexOf
method:
const startIndex = str.indexOf(':');
This will give us the index of the first occurrence of ":" within the string.
Step 2: Find the index of the second character (";" in this case). Again, we can use the indexOf
method:
const endIndex = str.indexOf(';');
This will provide us with the index of the first occurrence of ";" within the string.
Step 3: Extract the substring between the two indices. The substring
method will come in handy here:
const newStr = str.substring(startIndex + 1, endIndex);
The substring
method takes two arguments: the starting index (which we increment by 1 to exclude the ":" character) and the ending index (which includes the ";" character in this context).
That's it! You've successfully extracted the desired substring.
Putting It All Together: Code Example
To make it easier for you to understand and apply this solution, here's the complete code example:
const str = 'MyLongString:StringIWant;';
const startIndex = str.indexOf(':');
const endIndex = str.indexOf(';');
const newStr = str.substring(startIndex + 1, endIndex);
console.log(newStr);
When you run this code, it will output "StringIWant" in the console, which is the desired substring.
Conclusion: A Quick and Easy Solution!
In this blog post, we've shown you a straightforward solution to extract a substring between two characters in JavaScript. By using the substring
and indexOf
methods, you can easily obtain the desired portion of a string.
Remember, understanding how to manipulate strings and extract substrings is a valuable skill that can come in handy across various programming tasks.
Now it's your turn to put this into action! Give it a try and play around with different strings and characters to extract various substrings!
If you found this blog post helpful, please share it with your friends and colleagues. And don't forget to leave a comment below if you have any questions or suggestions. Happy coding! 💻🚀
[JavaScript]: A programming language often used for web development.