get size of JSON object
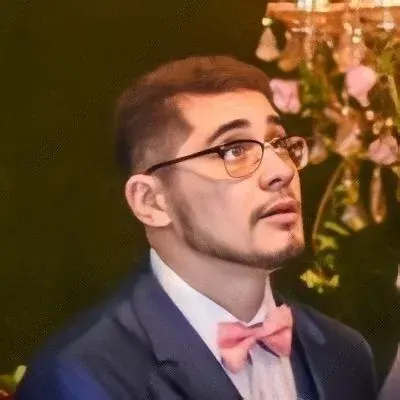
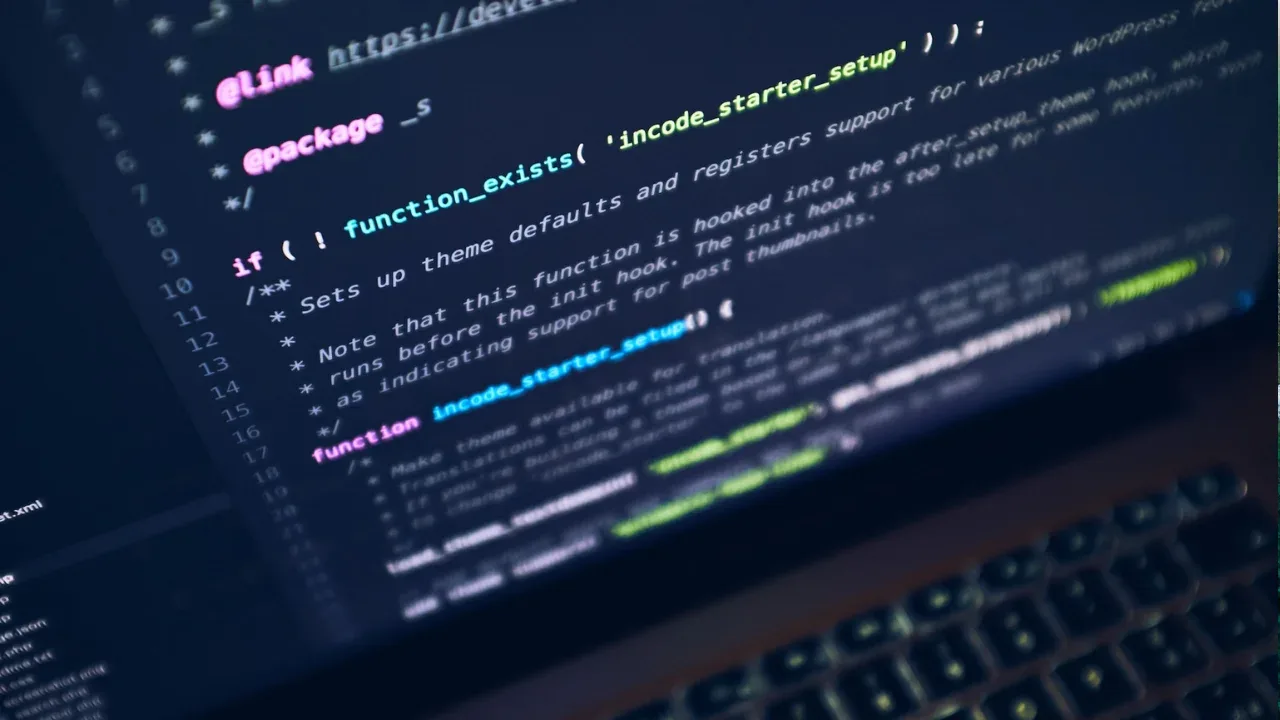
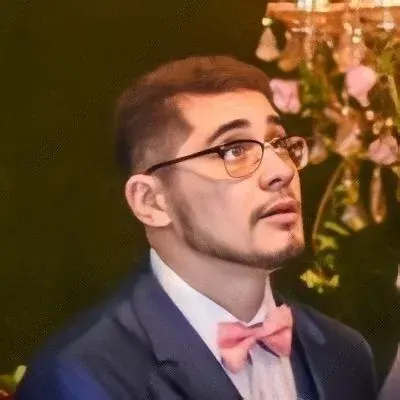
How to Get the Size of a JSON Object: A Complete Guide 📚🔍
So you're trying to get the size of a JSON object returned by an AJAX request, but no matter how hard you try, it keeps returning undefined
. Frustrating, right? But don't worry, because today I'm here to demystify this problem and provide you with easy solutions!
First, let's take a look at the code snippet you shared:
console.log(data.length);
console.log(data.phones.length);
When you try to use the length
property on a JSON object like data
, it's no surprise that you're getting undefined
. The reason is simple: JSON objects don't have a built-in .length
property like arrays do. However, you can still achieve what you're looking for by using different approaches.
Approach 1: Using the Object.keys() Method 💡🔑
One way to get the size of a JSON object is by using the Object.keys()
method. It returns an array of a given object's own enumerable properties. By then accessing the .length
property of that array, you can get the size you're looking for. Here's an example of how you can modify your code to use this method:
console.log(Object.keys(data).length);
console.log(Object.keys(data.phones).length);
🔍 Explanation: The Object.keys(data)
returns an array with all the property names of data
. By accessing the .length
property of that array, we get the size of the JSON object. Similarly, Object.keys(data.phones)
does the same for the phones
property within data
.
Approach 2: JSON.stringify() and String.length 🏗️📏
Another approach is to convert the JSON object to a string using JSON.stringify()
and then get the size of that string using the .length
property. This method is especially useful if you want the total size of the JSON object, including all its properties and their values. Here's an example:
var jsonString = JSON.stringify(data);
console.log(jsonString.length);
🔍 Explanation: The JSON.stringify()
method converts the data
object into a JSON string representation. By accessing the .length
property of that string, we get the size of the JSON object.
A Word of Caution ⚠️
It's important to note that the size you'll get using these methods is approximate and may not accurately represent the actual size of the original JSON object. This is because JSON objects, especially those containing nested objects or arrays, can have different sizes in memory due to various factors like encoding and compression.
Take Action and Share Your Experiences! 🚀📢
Now that you know how to get the size of a JSON object, it's time to put this knowledge into action! Try implementing the code snippets I provided above and see if they solve your problem.
If you've encountered any other issues or have alternative solutions, please share them in the comments below. Your experiences and insights could help fellow developers who are facing similar challenges.
Remember, sharing is caring! Don't forget to hit that share button and spread the knowledge with your friends and colleagues. Happy coding! 😄👩💻👨💻