Get selected value in dropdown list using JavaScript
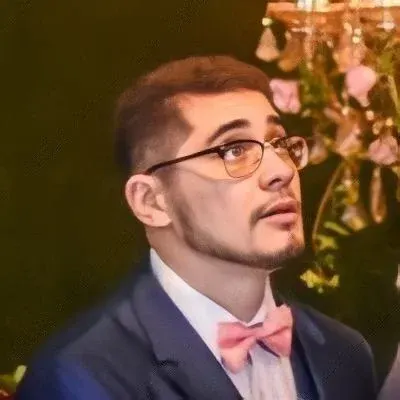
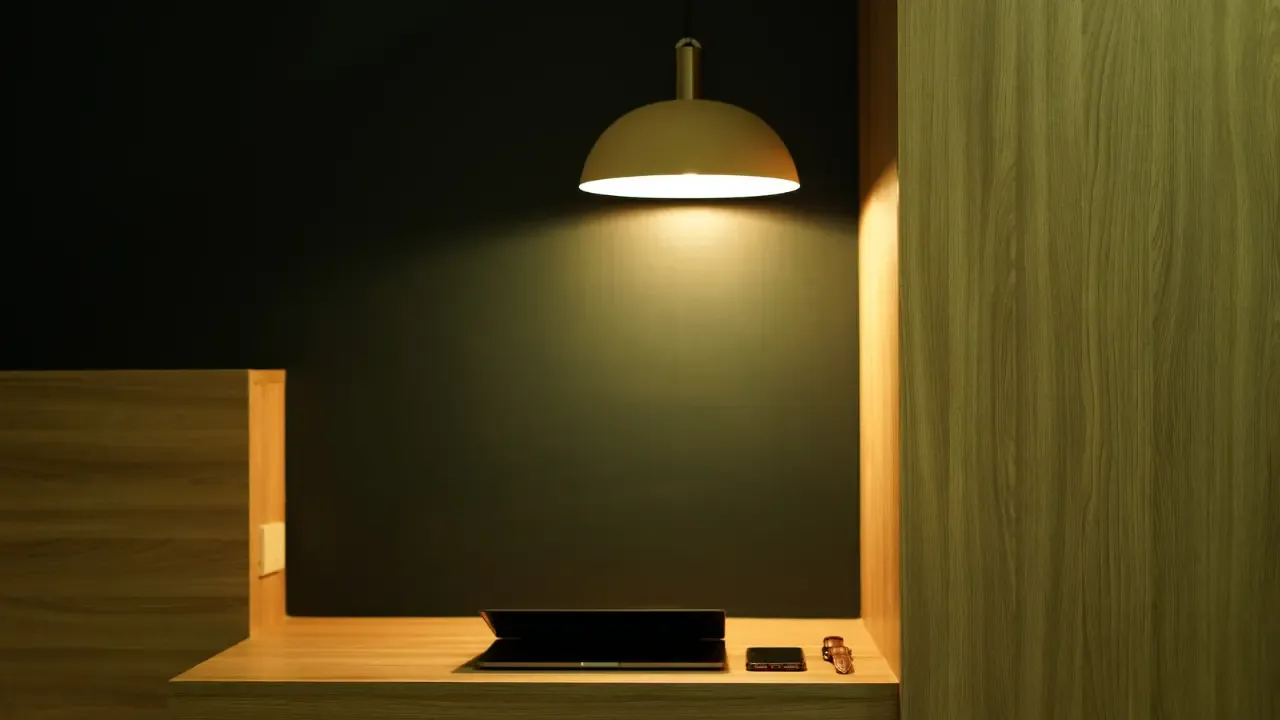
📝 Hey there, tech enthusiasts! Today, we are diving into a common JavaScript challenge: how to get the selected value from a dropdown list. 🤔 Don't worry, we've got you covered! In this blog post, we will walk you through the process, provide easy solutions, and give you an exciting call-to-action at the end. Let's get started! 🚀
The Problem
So, you have an HTML form with a dropdown list, and you want to retrieve the selected value using JavaScript. Here's an example to illustrate the context:
<form>
<select id="ddlViewBy">
<option value="1">test1</option>
<option value="2" selected="selected">test2</option>
<option value="3">test3</option>
</select>
</form>
Solution 1: Using Vanilla JavaScript
The simplest way to grab the selected value is by using plain JavaScript. We can fetch the value using the value
property of the selected <option>
element. Let's see it in action:
const dropdown = document.getElementById("ddlViewBy");
const selectedValue = dropdown.value;
console.log(selectedValue); // Output: 2
In the code above, we first obtain the dropdown element using getElementById()
, providing the ID of the dropdown. Then, with dropdown.value
, we retrieve the selected value and assign it to the selectedValue
variable. Finally, with console.log()
, we can see the value in our browser's console. Easy peasy! 🙌
Solution 2: Using jQuery 🤩
If you're already using the jQuery library in your project, you'll love this solution. jQuery provides a simple and concise way to extract the selected value. Here's how you can achieve it:
const selectedValue = $("#ddlViewBy").val();
console.log(selectedValue); // Output: 2
In the code snippet above, we leverage the power of jQuery by using the val()
function. By targeting the dropdown's ID with $("#ddlViewBy")
, we efficiently retrieve the selected value. The selectedValue
variable captures the result, and console.log()
helps us see it in action. jQuery makes life easy, doesn't it? 😍
Conclusion
Now you know two handy solutions to fetch the selected value from a dropdown list using JavaScript. Whether you prefer the elegance of vanilla JavaScript or enjoy the convenience of jQuery, our guide has got you covered! 🎉
If you enjoyed this article and found it helpful, don't forget to share it with your fellow tech enthusiasts. Feel free to leave your thoughts and any alternative methods in the comments section below! 👇
Keep coding and keep learning! 💻💡
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
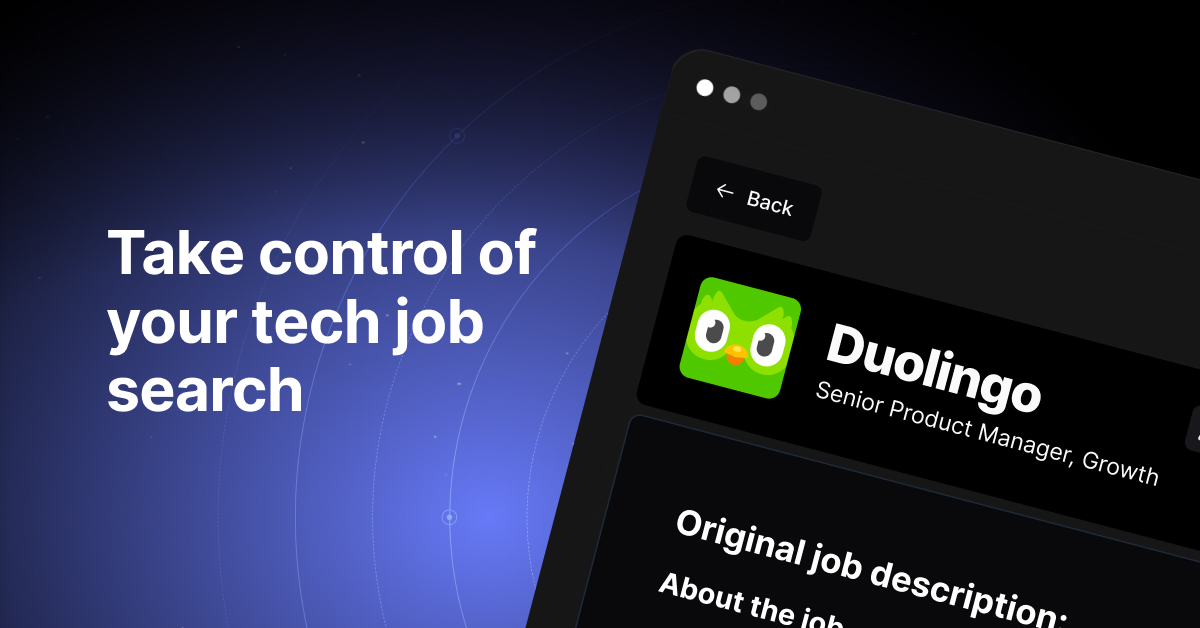