Get local IP address in Node.js
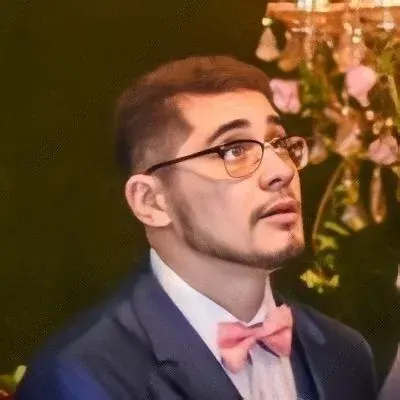
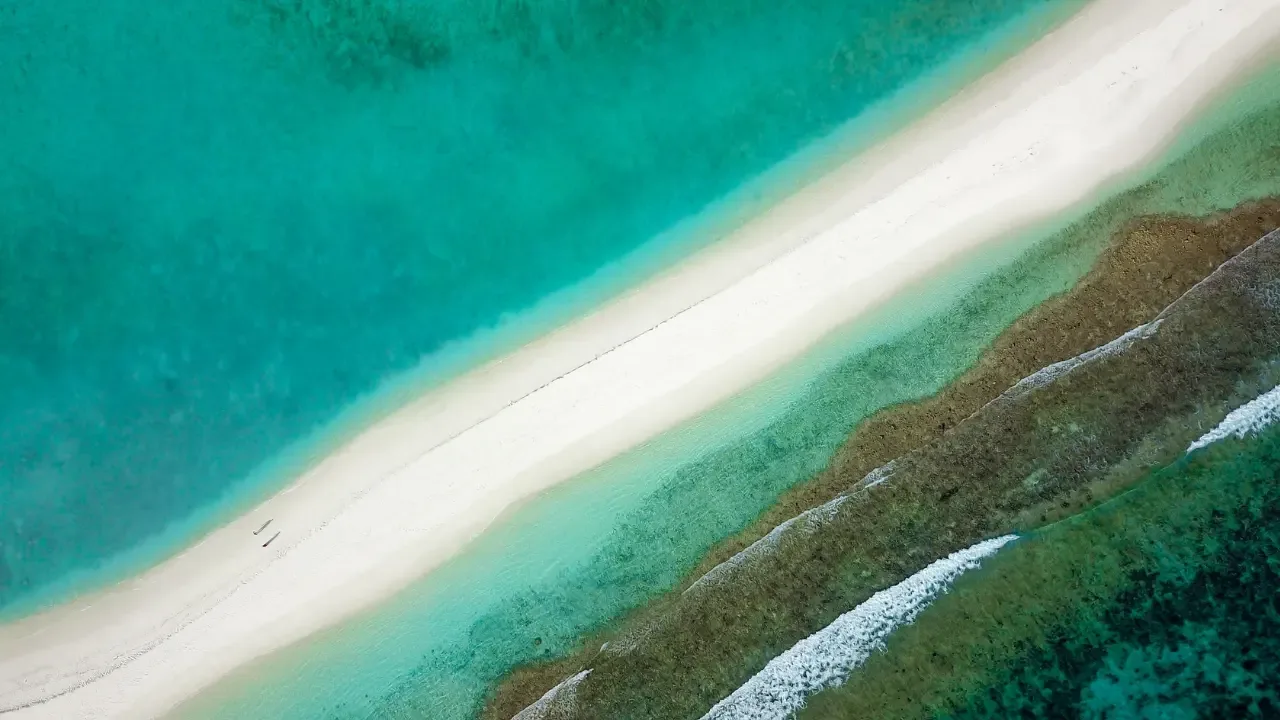
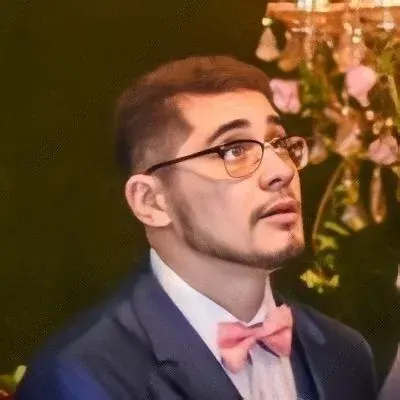
How to Get the Local IP Address in Node.js? 🌐💻
Are you working on a cool Node.js project and need to fetch the local IP address of the machine running your program? No worries, we've got you covered! 🤩 In this guide, we'll walk you through a step-by-step process to retrieve the local IP address using Node.js. Let's dive in! 💨📝
The Common Problem 🤔❓
So, you have a simple Node.js program up and running, and you're wondering how to acquire the IP address of the machine it's currently executed on. This is a common issue faced by many developers, especially when dealing with network-related tasks or building applications that require machine specifics.
The Easy Solution 💡🛠️
To fetch the local IP address using Node.js, we can utilize the os
module, which provides various methods for interacting with the operating system. Specifically, we'll use the networkInterfaces()
method to retrieve network interface information, including IP addresses.
Here's a simple code snippet that demonstrates how to get the local IP address:
const os = require('os');
const networkInterfaces = os.networkInterfaces();
// Iterate over network interfaces
Object.keys(networkInterfaces).forEach(interface => {
// Filter out non-internal IPv4 addresses
const address = networkInterfaces[interface].find(
address => !address.internal && address.family === 'IPv4'
);
// Output the local IP address
if (address) {
console.log(`Local IP Address: ${address.address}`);
}
});
In the above example, we use os.networkInterfaces()
to obtain an object containing network interface details. We iterate over each interface, filter out non-internal IPv4 addresses, and output the IP address using console.log()
.
Voila! 🎉 By running this code, you should see the local IP address displayed in your console. Isn't that awesome? 😄
Other Considerations 🤔🔍
While the solution mentioned above should work for most scenarios, there are a few additional points worth considering:
Multiple IP Addresses 🌐🌐
It's possible for a machine to have multiple IP addresses due to different network interfaces or virtualization technologies. Be aware that the provided code snippet retrieves only the first non-internal IPv4 address found. If your use case requires handling multiple addresses, you may need to modify the code accordingly.
IPv6 Addresses ⚙️🔢
The example code focuses on retrieving IPv4 addresses since they are the most commonly used. However, if your application requires fetching IPv6 addresses or both, you can modify the code to include those as well. Simply adjust the filter condition to match the desired IP address family.
Time to Implement! ⚡👩💻👨💻
Now that you have the solution at your fingertips, go ahead and integrate it into your Node.js project. Fetching the local IP address will surely come in handy for tasks like binding to a specific network interface or displaying machine-specific information in your application. 💪🏻🔌
Feel free to experiment, build upon the code, and let us know how it works out for you. We'd love to hear about the cool projects you're working on! 🚀💬
That's all for now! We hope this guide has been helpful to you. If you have any questions or suggestions, please leave a comment below. Happy coding! 😊🎉