Get keys of a Typescript interface as array of strings
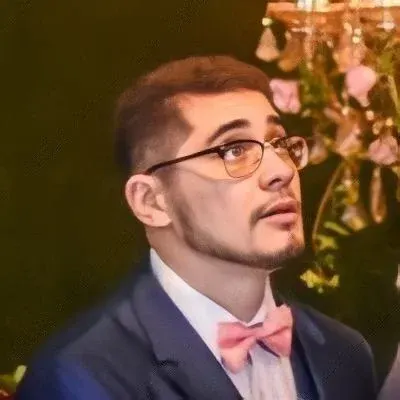
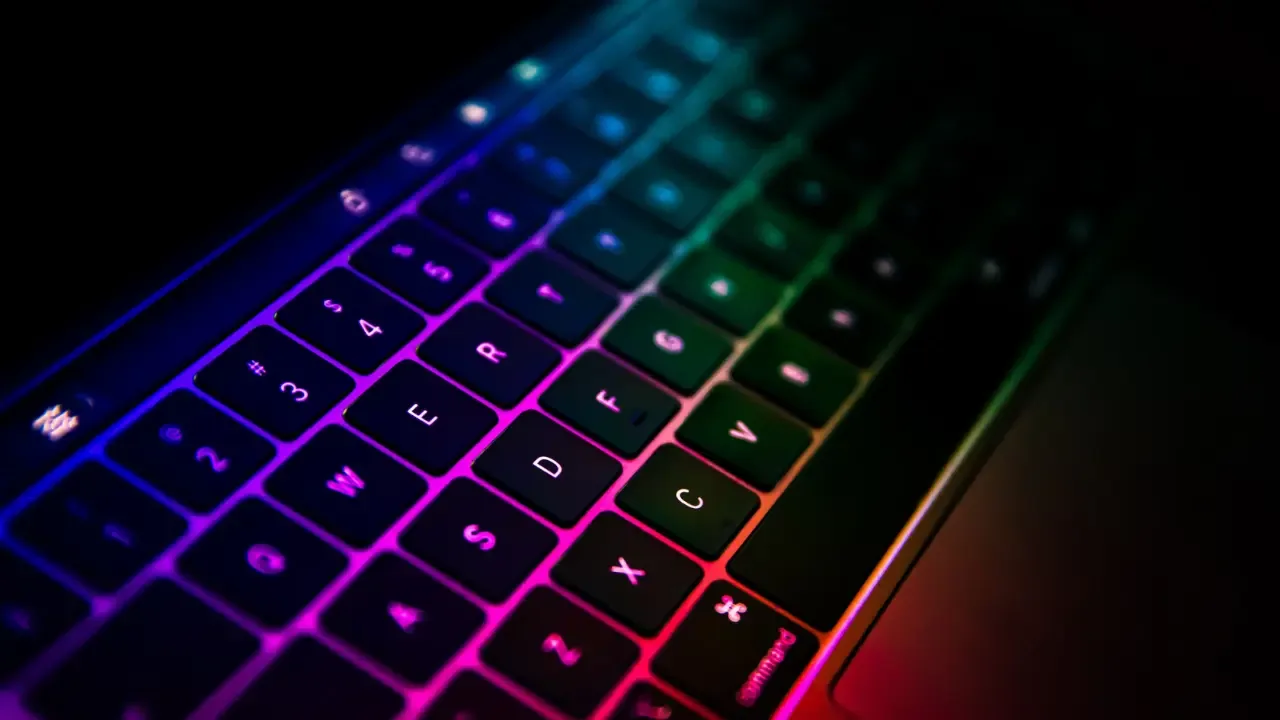
Getting Keys of a TypeScript Interface as an Array of Strings
Are you struggling to extract the property names of a TypeScript interface and store them in an array of strings? Don't worry, we've got you covered! In this blog post, we will address this common issue and provide you with easy solutions to achieve the desired result. 🚀
Understanding the Problem
Let's start by understanding the problem at hand. You have multiple tables defined in the Lovefield library, each with its respective interface containing the table's columns. You want to generate an array that contains the property names from each interface dynamically. This entails extracting the keys (property names) from the TypeScript interface and storing them in an array of strings.
Solution 1: Using keyof and typeof Operators
One way to achieve this is by utilizing the keyof
and typeof
operators in TypeScript. These operators allow us to perform type inference and access the keys of an interface.
Here's how you can implement this solution:
export interface IMyTable {
id: number;
title: string;
createdAt: Date;
isDeleted: boolean;
}
const keysOfIMytable: Array<keyof IMyTable> = Object.keys({}) as Array<keyof IMyTable>;
In this solution, we defined an interface called IMyTable
. We then created a variable called keysOfIMyTable
which is an array of keyof IMyTable
. By using Object.keys({}) as Array<keyof IMyTable>
, we ensure that the resulting array contains only the keys of the IMyTable
interface.
Solution 2: Using Type Assertion
Alternatively, you can achieve the desired result by employing type assertion:
const IMyTableKeys = Object.keys({}) as Array<keyof IMyTable>;
In this solution, we directly assign the resulting array of keys to a variable called IMyTableKeys
. By utilizing type assertion (as Array<keyof IMyTable>
), we inform TypeScript of the expected type of the variable.
Solution 3: Using mapped Types
TypeScript provides mapped types as a powerful way to create new types based on existing ones. We can utilize a mapped type to extract the keys of an interface.
type KeysOf<T> = {
[K in keyof T]: K;
};
const IMyTableKeys: Array<keyof IMyTable> = Object.keys({}) as Array<keyof IMyTable>;
In this solution, we defined a generic KeysOf
type that, when applied to an interface, maps each key to itself. We then use Object.keys({}) as Array<keyof IMyTable>
to obtain an array of keys, as seen in previous solutions.
Conclusion
Congratulations! You've learned multiple solutions to extract the keys of a TypeScript interface and store them as an array of strings. By using the keyof
and typeof
operators, type assertion, or mapped types, you can easily achieve the desired result.
Now it's time for you to put this knowledge into action! Try out these solutions with your Lovefield tables or any TypeScript interfaces you're working with.
Do you have any other TypeScript-related questions or challenges? Let us know in the comments below, and we'll be more than happy to help you out. Happy coding! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
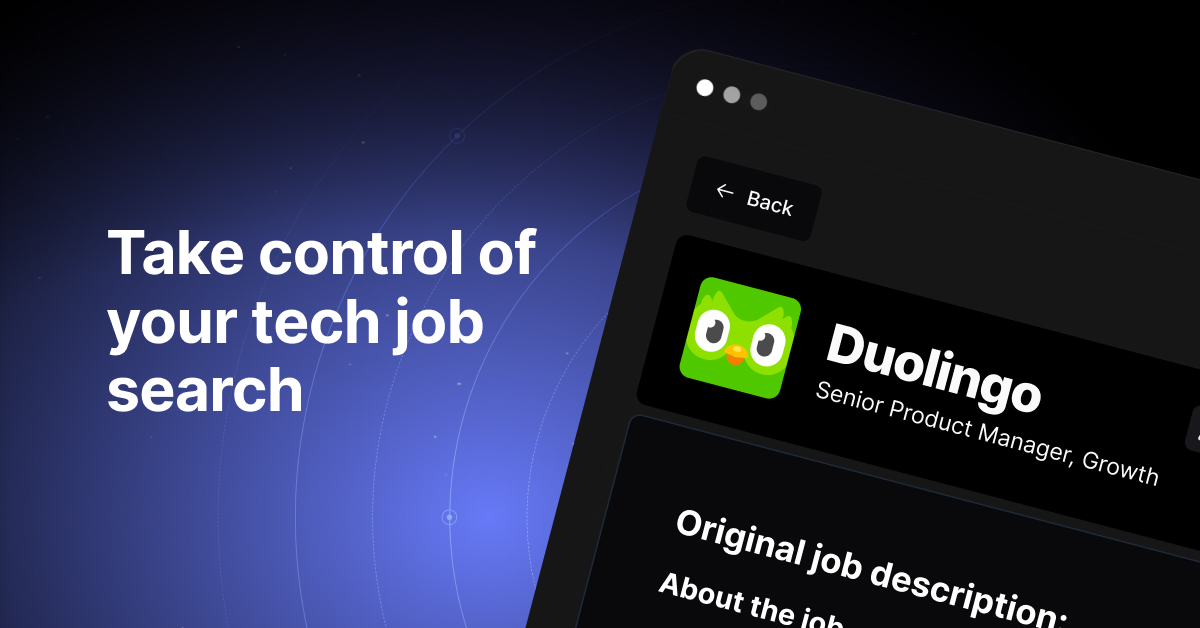