Get current url in Angular
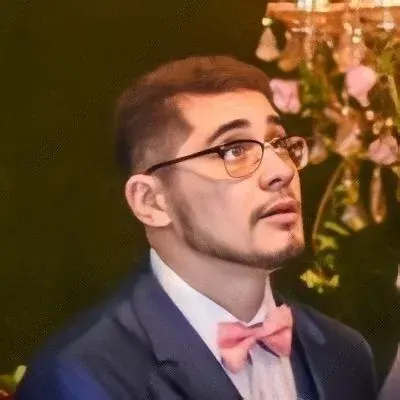
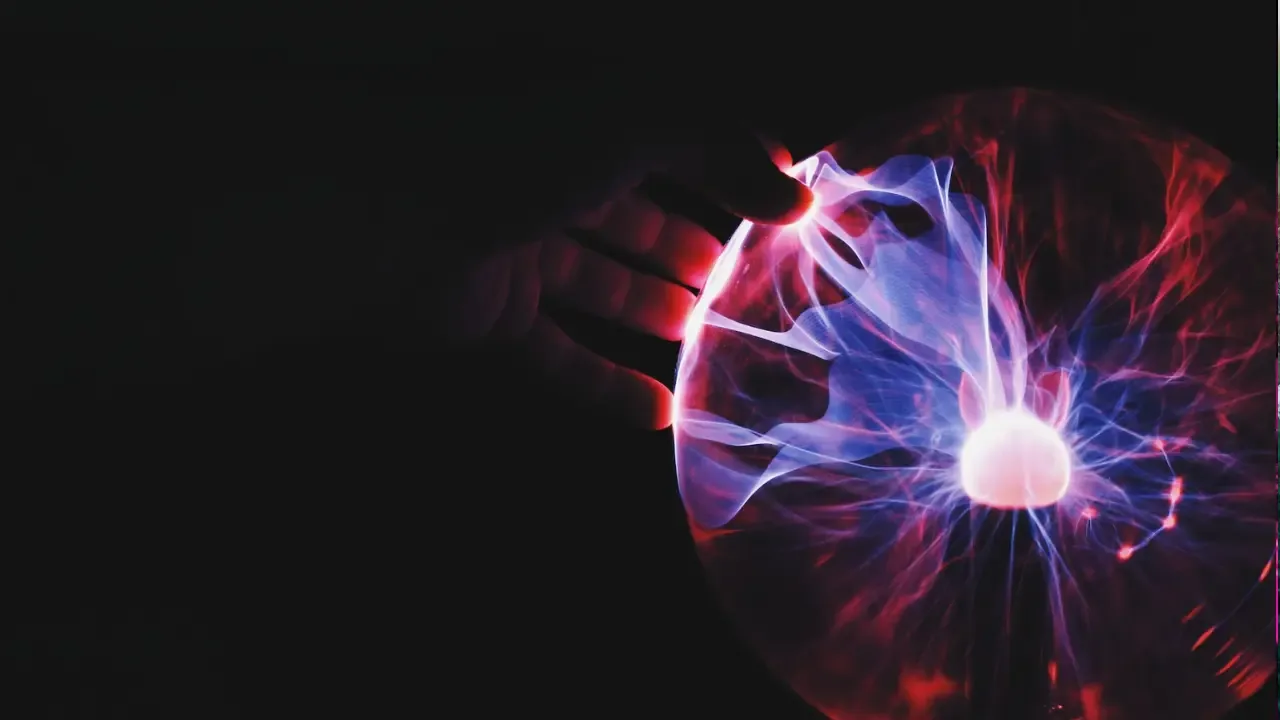
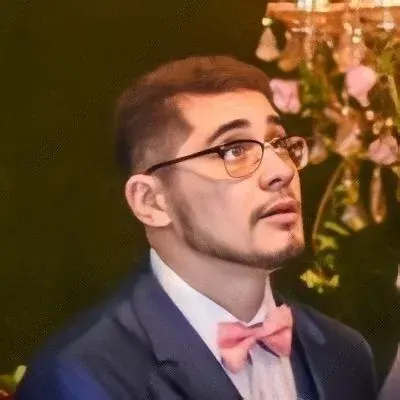
🌐 How to Get Current URL in Angular
Are you struggling to get the current URL in your Angular 4 project? You're not alone! Many developers face this challenge, but fear not! In this blog post, we'll explore the common issues and provide easy solutions to help you get the current URL. By the end, you'll be able to retrieve the current URL without breaking a sweat!
🚀 The Problem
One user shared their frustration on the internet, saying, "How can I get the current URL in Angular 4? I've searched the web a lot, but I can't find a solution."
🤔 Understanding the Context
To better understand the problem at hand, let's take a look at the provided code snippets.
app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { RouterModule, Router } from '@angular/Router';
import { AppComponent } from './app.component';
import { TestComponent } from './test/test.component';
import { OtherComponent } from './other/other.component';
import { UnitComponent } from './unit/unit.component';
@NgModule({
declarations: [AppComponent, TestComponent, OtherComponent, UnitComponent],
imports: [
BrowserModule,
RouterModule.forRoot([
{
path: 'test',
component: TestComponent
},
{
path: 'unit',
component: UnitComponent
},
{
path: 'other',
component: OtherComponent
}
])
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {}
app.component.html
<!-- The content below is only a placeholder and can be replaced -->
<div>
<h1>Welcome to {{ title }}!!</h1>
<ul>
<li><a routerLink="/test">Test</a></li>
<li><a routerLink="/unit">Unit</a></li>
<li><a routerLink="/other">Other</a></li>
</ul>
</div>
<br />
<router-outlet></router-outlet>
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Angular JS 4';
arr = ['abcd', 'xyz', 'pqrs'];
}
other.component.ts
import { Component, OnInit } from '@angular/core';
import { Location } from '@angular/common';
import { Router } from '@angular/router';
@Component({
selector: 'app-other',
templateUrl: './other.component.html',
styleUrls: ['./other.component.css']
})
export class OtherComponent implements OnInit {
public href: string = "";
url: string = "asdf";
constructor(private router: Router) {}
ngOnInit() {
this.href = this.router.url;
console.log(this.router.url);
}
}
test.component.ts
import { Component, OnInit } from '@angular/core';
import { Location } from '@angular/common';
import { Router } from '@angular/router';
@Component({
selector: 'app-test',
templateUrl: './test.component.html',
styleUrls: ['./test.component.css']
})
export class TestComponent implements OnInit {
route: string;
currentURL = "";
constructor() {
this.currentURL = window.location.href;
}
ngOnInit() {}
}
💡 The Solution - Router Injection
Looking at the code snippets, we can see that both the TestComponent
and OtherComponent
are using the Router
from @angular/router
to access the current URL. However, both components encounter issues, as mentioned in the provided error message.
The error message, ERROR Error: Uncaught (in promise): Error: No provider for Router!
, suggests that the Router
dependency has not been properly injected into the components.
To fix this issue, we need to update both TestComponent
and OtherComponent
to include the Router
in their constructor, allowing Angular to provide the necessary dependency.
other.component.ts
import { Component, OnInit } from '@angular/core';
import { Location } from '@angular/common';
import { Router } from '@angular/router';
@Component({
selector: 'app-other',
templateUrl: './other.component.html',
styleUrls: ['./other.component.css']
})
export class OtherComponent implements OnInit {
public href: string = "";
url: string = "asdf";
constructor(private router: Router) {}
ngOnInit() {
this.href = this.router.url;
console.log(this.router.url);
}
}
test.component.ts
import { Component, OnInit } from '@angular/core';
import { Location } from '@angular/common';
import { Router } from '@angular/router';
@Component({
selector: 'app-test',
templateUrl: './test.component.html',
styleUrls: ['./test.component.css']
})
export class TestComponent implements OnInit {
route: string;
currentURL = "";
constructor(private router: Router) {
this.currentURL = window.location.href;
}
ngOnInit() {}
}
🎉 Problem Solved!
By injecting the Router
using the private
keyword in both TestComponent
and OtherComponent
constructors, we have successfully resolved the issue of getting the current URL.
💪 Take Action!
Now that you have learned how to get the current URL in Angular, go ahead and implement this solution in your own project. Remember, getting the current URL is just a small part of Angular's capabilities. Keep exploring and experimenting with Angular's powerful features to level up your development skills!
👍 Share Your Success!
Did this solution help you? Share your success story on social media! Let others know how you overcame the challenge of getting the current URL in Angular 4. Use the hashtags #Angular and #CodingVictory to connect with other developers and inspire them with your achievements.
Keep coding! 🚀👩💻👨💻