Get class list for element with jQuery
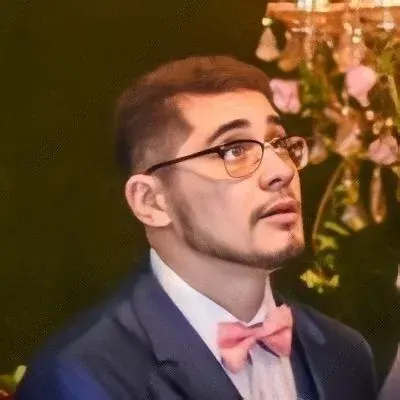
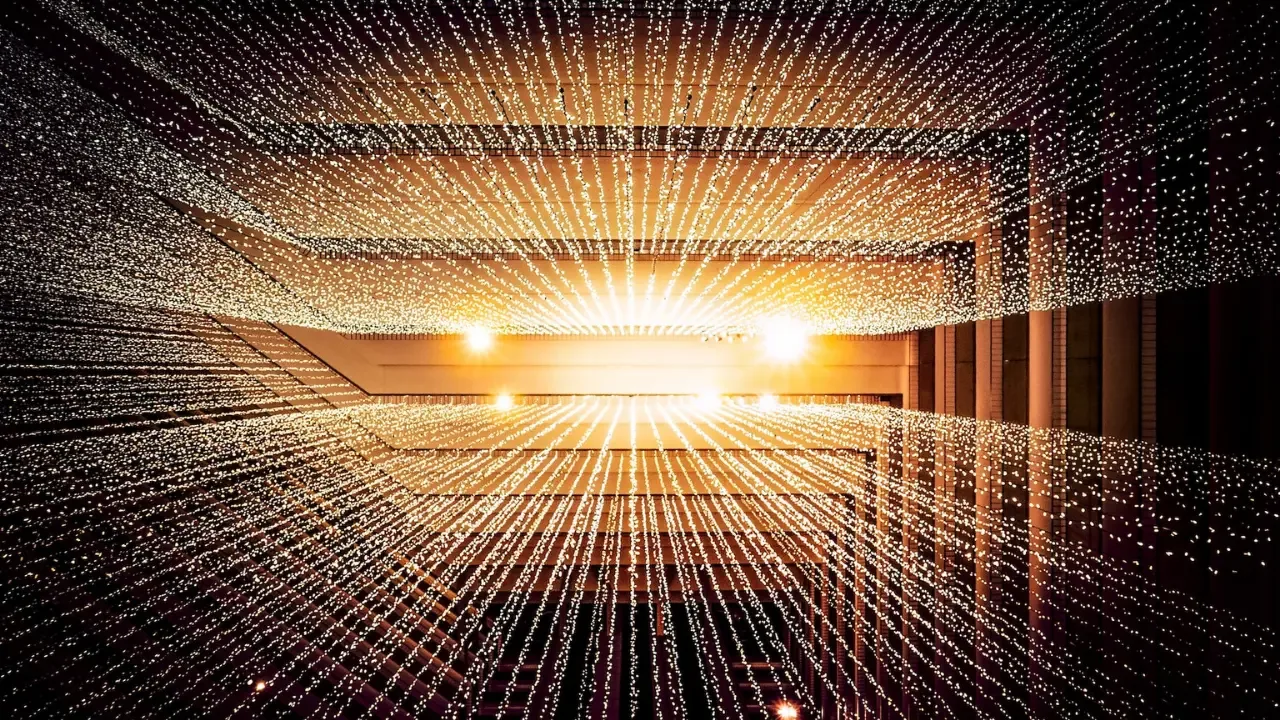
📝 Easily Get Class List for an Element with jQuery
Are you struggling to find a way to loop through or assign an array of all the classes assigned to a specific element using jQuery? Look no further! In this blog post, we'll address this common issue and provide you with easy solutions.
The Problem
Let's consider the following example:
<div class="Lorem ipsum dolor_spec sit amet">Hello World!</div>
In this example, we want to find the "special" class named "dolor_spec" without knowing the actual class name beforehand.
The Solution
To solve this problem, we can leverage the power of jQuery and its built-in methods. Here's how you can easily get the class list for an element:
// Select the element using a specific identifier (e.g., ID, tag name, etc.)
const $element = $('#yourElementId');
// Get the class list as an array
const classList = $element.attr('class').split(' ');
// Loop through the array to find the desired class
$.each(classList, function(index, className) {
if (className.includes('special')) { // Replace 'special' with your desired class name filter
// Do something with the element that has the desired class
console.log("Found the desired class: " + className);
}
});
In the code above, we first select the element using its identifier. You can use different jQuery selectors to select the element you need. In this example, we're using an ID selector as #yourElementId
. Replace it with the appropriate selector for your use case.
Next, we retrieve the class attribute of the element using the attr
method and then split it into an array using the split
method. This gives us an array of all the classes assigned to the element.
Finally, we loop through the class list array using $.each
method. Inside the loop, we check if the class name includes the desired class filter (in this case, 'special'). If it matches, we can perform the desired action or manipulation on the element.
Remember to replace 'special' with the desired class name you are looking for. You can customize this code to fit your specific requirements.
Take it to the Next Level
Now that you know how to easily get the class list for an element using jQuery, why stop here? Embrace the power of JavaScript frameworks like React or Angular to build powerful web applications!
Share your experience with implementing this solution or let us know if you have any further questions. We love hearing from our readers!
📣 Your Turn!
Have you ever encountered difficulties when dealing with class lists in jQuery? How did you solve them? Share your thoughts, experiences, or questions in the comments below!
References:
Disclaimer: The code provided in this blog post is for illustrative purposes only and may require further customization to fit your specific use case.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
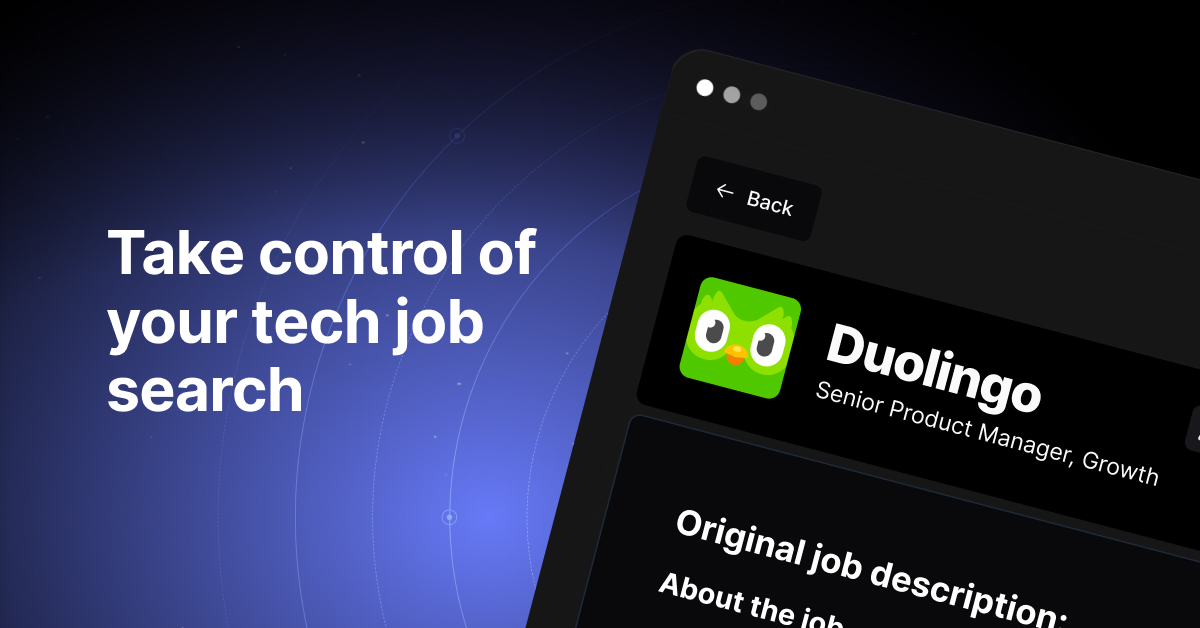