Get all unique values in a JavaScript array (remove duplicates)
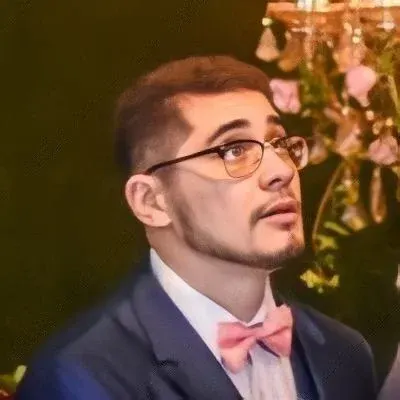
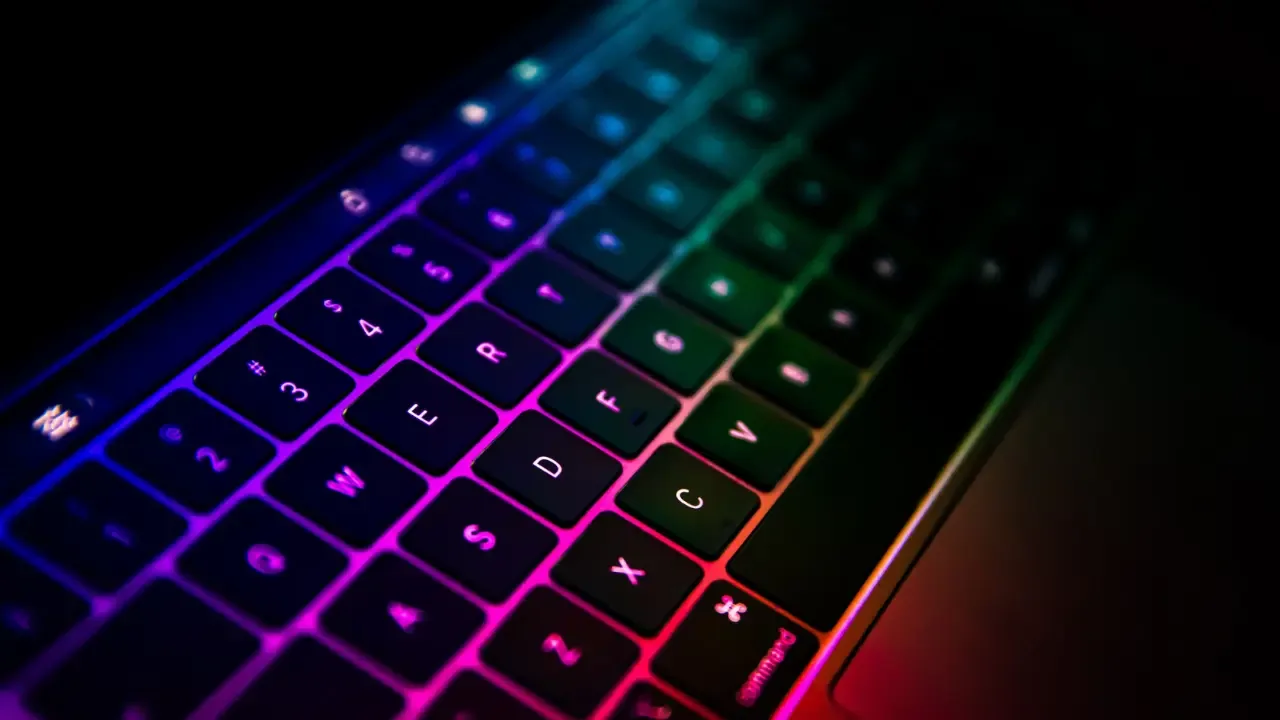
Getting all Unique Values in a JavaScript Array
š Welcome to my tech blog, where we'll be tackling an intriguing question: how can we retrieve all unique values from a JavaScript array, thus removing any duplicates? š
The Inquiry
š Our quest begins with a developer who encountered an issue while utilizing a particular code snippet from the internet. Their array of numbers became problematic when it contained a zero. Their curiosity piqued, they ingeniously turned to the Stack Overflow community for insight. š”
The Flawed Solution
š Let's take a look at the code snippet our intrepid developer found:
Array.prototype.getUnique = function() {
var o = {}, a = [], i, e;
for (i = 0; e = this[i]; i++) {o[e] = 1};
for (e in o) {a.push(e)};
return a;
}
š¤ While this solution appears promising, our developer discovered that it fails when processing an array that includes a zero. This leads us to investigate where the prototype script is going wrong. šµļøāāļø
Analyzing and Fixing the Issue
āļø To identify the problem, we need to understand the inner workings of the code snippet. Let's break it down step by step:
The code snippet extends the "Array" prototype with a new function called "getUnique".
The function initializes an object "o" (acting as a dictionary) and an empty array "a".
A "for" loop iterates through each element "e" in the array.
The "for" loop populates the dictionary "o" by assigning a value of 1 to each element "e" found.
Another "for" loop is used to extract the unique elements from the dictionary "o" and push them into the "a" array.
Finally, the function returns the array "a" containing all the unique values.
š The flaw in this code lies in the fact that object keys in JavaScript are strings, even for numeric values. Consequently, when a zero is encountered, it becomes falsely identified as a duplicate. š
š Fear not! I have a modified solution that resolves this issue by utilizing the "Set" object introduced in ES6. Here's the updated code:
Array.prototype.getUnique = function() {
return Array.from(new Set(this));
}
š Now, let's explain the modifications made to the original code:
The code snippet no longer creates a separate object or array.
It uses the "Set" object, which automatically eliminates duplicates, as it only retains unique values.
The "Set" object is initialized by passing our array using the "this" keyword.
To convert the "Set" object back into an array, we use the "Array.from" method.
š ļø With a few adjustments, the updated solution provides a more reliable and concise approach to obtain unique values from an array. No more false positives! š«š
Further Explorations
šµļøāāļø If you're still hungry for more knowledge, you might find the following Stack Overflow posts relevant and interesting:
š„ Now that we've successfully tackled the issue of retrieving all unique values from a JavaScript array, you can use this knowledge to optimize your code and ensure data accuracy. Keep exploring, stay curious, and happy coding! šāØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
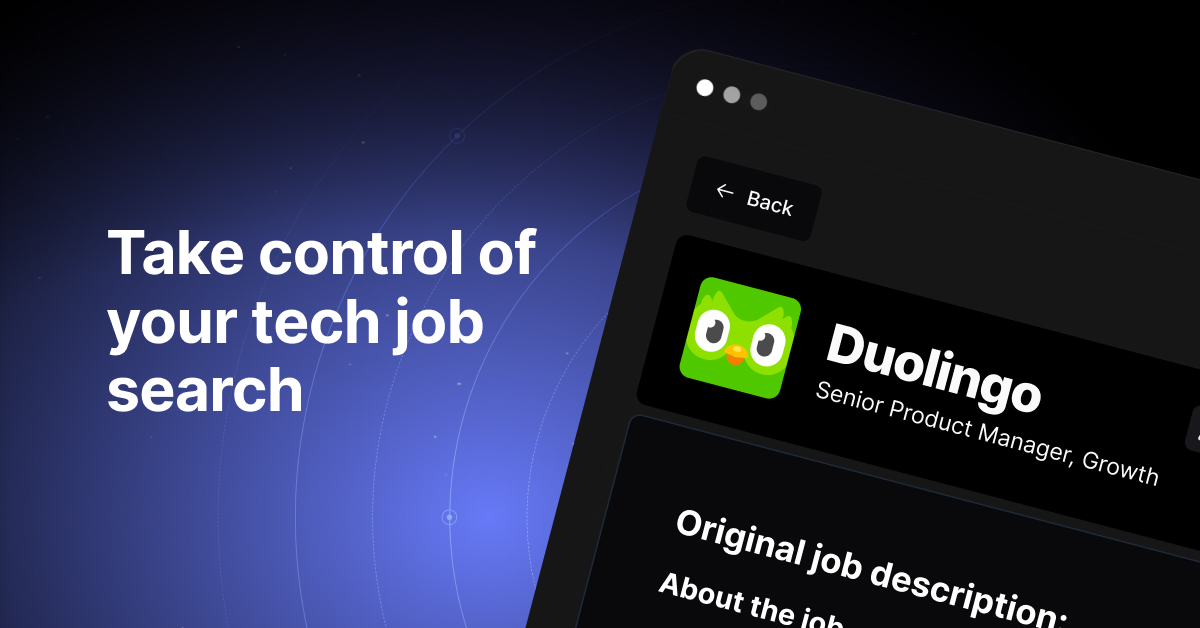