Get all non-unique values (i.e.: duplicate/more than one occurrence) in an array
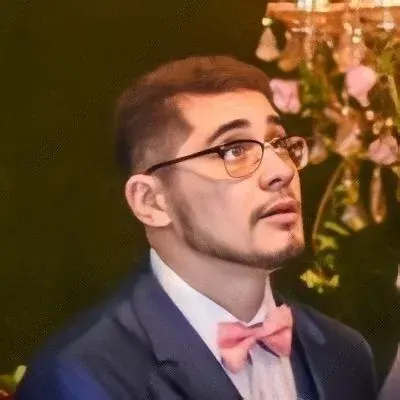
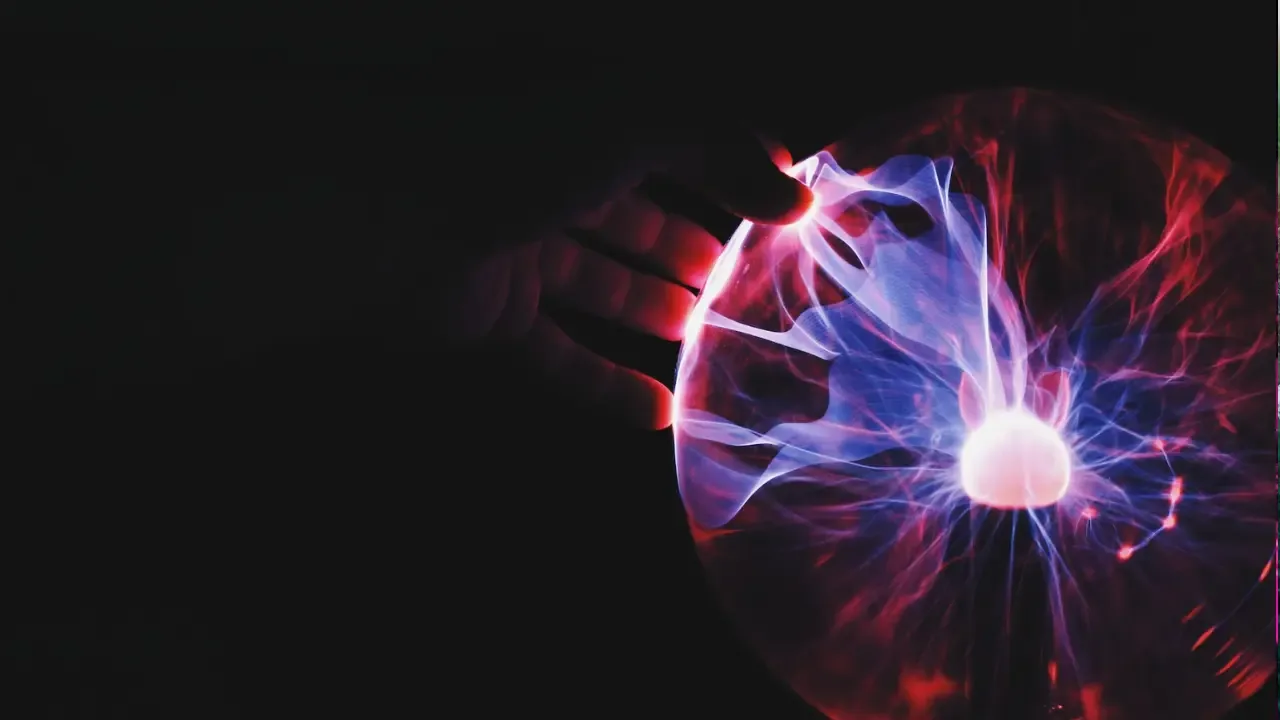
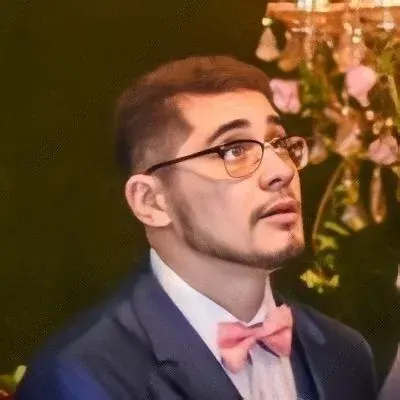
Easy-Peasy: How to Get All Non-Unique Values in an Array
Are you tired of manually checking for duplicate values in a JavaScript array? 🔄
Well, fret no more! 🛡️
In this blog post, we'll uncover the easiest way to detect and retrieve all those pesky non-unique values in your array 🕵️♀️ without the hassle of index-finding or tallying duplicate counts.
So, let's dive right in and get our array-game on! 💪
The Context
Our curious reader posed this intriguing question: "What's the easiest way to find duplicated values in a JavaScript array without the need for index information or counts?"
Good news! The JavaScript gurus have got you covered like a cozy array blanket. 🛌💤
The Solution 🌟
Introducing the Array.filter()
method! 🆒🔍
This magical function allows us to create a new array with only the elements that pass a given test. And conveniently, with this test, we can easily identify non-unique values. 🎉
Here's the snippet to bring smiles to your array-detecting dreams:
const array = [1, 2, 3, 4, 1, 3, 5, 6];
const nonUniqueValues = array.filter((value, index, self) => self.indexOf(value) !== index);
console.log(nonUniqueValues); // [1, 3]
And voilà! 🎩
After applying the .filter()
method along with a handy comparison of the index of each value, we swiftly retrieve all those duplicate entries. No indexes, no counting, just the non-unique values you've longed for! 😍
But wait, there's more! 📣
In the event that you have an array of objects or complex data structures, worry not my friend! 🚀
With a slight modification to our solution, you can conquer the arrays of objects too! 🏆
Here's an example using objects as array elements:
const people = [
{ name: 'John', age: 20 },
{ name: 'Jane', age: 30 },
{ name: 'John', age: 40 },
{ name: 'Jim', age: 20 },
];
const nonUniquePeople = people.filter((person, index, self) =>
self.findIndex((p) => p.name === person.name) !== index
);
console.log(nonUniquePeople);
// [
// { name: 'John', age: 20 },
// { name: 'John', age: 40 },
// ]
Boom! 💥
By combining .filter()
with .findIndex()
, we defeat the mighty object arrays too. We specifically target the duplicate objects based on a particular property, in this case, the name
. Flex that array power! 💪
One Last Thing... 🛎️
Learning is great, but utilizing knowledge is even better! 💡
Now that you have the power to easily identify non-unique values in arrays, go forth and create amazing things! 🌟 Share your newfound wisdom, inspire others, and remember, there's always something new to explore in the realm of coding. 🚀
So, let's hear from you! Have you ever encountered a tricky array situation, and if so, how did you resolve it? Share your stories, tips, and tricks in the comments below, and let's level up together! 👇💬