Get a random item from a JavaScript array
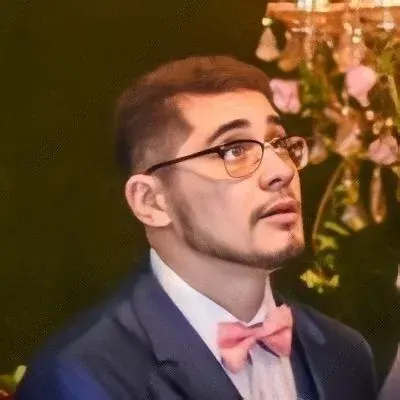
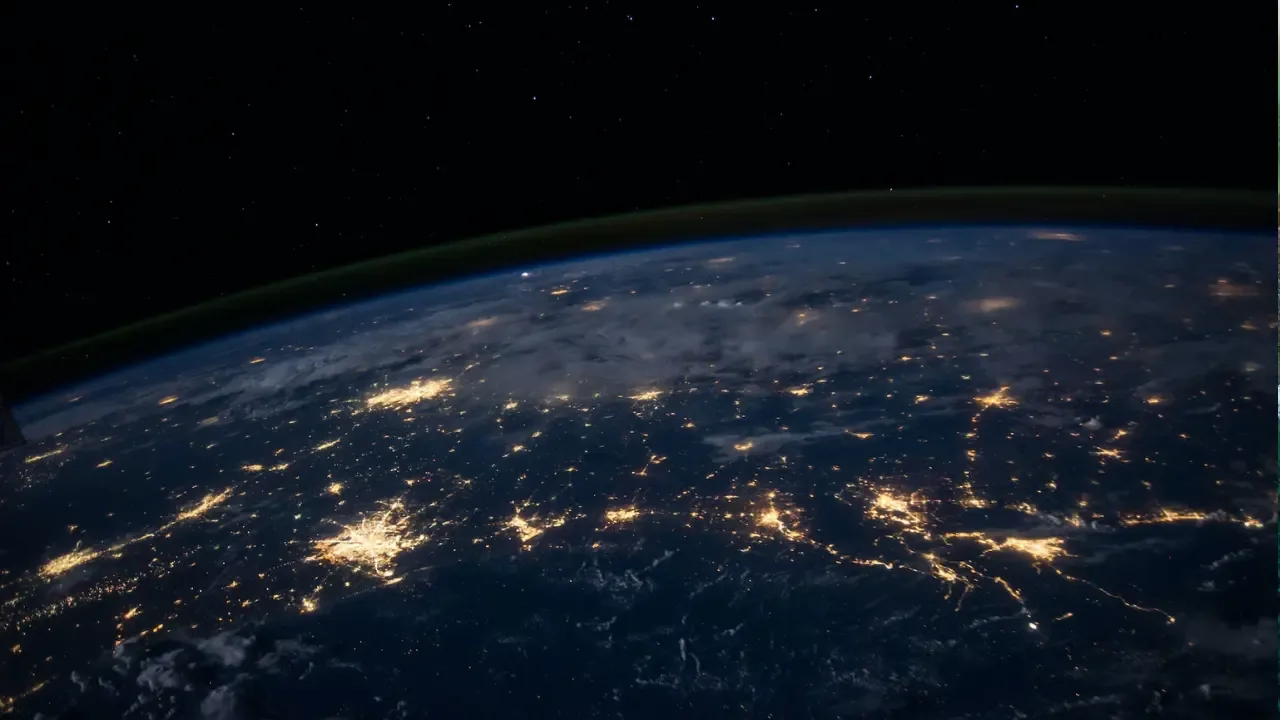
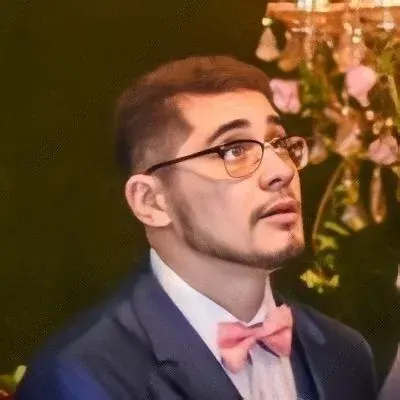
How to Get a Random Item from a JavaScript Array 🎲
So, you have an array in JavaScript and you want to pick a random item from it. Maybe you want to surprise your users with a random fact, or shuffle a deck of cards, or simply generate some random number for a game. Whatever the reason, we've got you covered! Let's dive right in and see how we can solve this problem. 🤓
The Problem 😕
Given the array items
with elements [523, 3452, 334, 31, ..., 5346]
, how can we randomly select an item from it? 🤔
The Solution 💡
Fortunately, JavaScript provides a convenient method called Math.random()
that returns a random floating-point number between 0 and 1 (exclusive). We can leverage this method to create a solution for randomly selecting an item from an array.
Here's a simple step-by-step solution:
var items = [523, 3452, 334, 31, ..., 5346];
var randomIndex = Math.floor(Math.random() * items.length);
var randomItem = items[randomIndex];
Let's break it down:
items
is the array containing your items. Replace[523, 3452, 334, 31, ..., 5346]
with the actual items you want to choose from.Math.random()
generates a random number between 0 and 1.Math.random() * items.length
multiplies the random number by the length of the array to get a random index within the valid range.Math.floor()
rounds down the calculated value to the nearest integer, ensuring it's a valid index.items[randomIndex]
retrieves the item at the randomly generated index.
And there you have it! 🎉 You can now randomly select an item from your JavaScript array.
Example Usage 🚀
Let's see this solution in action with a practical example. Suppose we have an array of positive affirmations and we want to display a random affirmation to the user.
var affirmations = [
"You are capable of great things!",
"You radiate positivity!",
"You deserve to be happy!",
"You are making a difference!"
// ... add more affirmations here
];
var randomIndex = Math.floor(Math.random() * affirmations.length);
var randomAffirmation = affirmations[randomIndex];
console.log(randomAffirmation);
In this example, we have an affirmations
array containing positive phrases. We generate a random index and retrieve a random affirmation from the array. We then print the random affirmation to the console, but you could display it in any way you like on your webpage or application.
Wrapping Up 🎁
Getting a random item from a JavaScript array is made easy with the power of the Math.random()
method and a few lines of code. Whether you're creating a game, building a random fact generator, or just adding an element of surprise to your application, this solution has got you covered.
Now it's your turn to try it out! Pick an array, replace the example items with your own data, and see the magic happen. Feel free to share your creative use cases and any questions you might have in the comments below. Happy coding! 💻🎲
📣 Call-to-Action:
Did you find this article helpful? Let us know in the comments section below. Share your thoughts and creative uses of the random item selection technique in JavaScript. Don't forget to hit the "Like" button and share this article with your fellow developers!
Follow us for more exciting JavaScript tips and tricks. See you in the next blog post! 😉🚀