Generating random whole numbers in JavaScript in a specific range
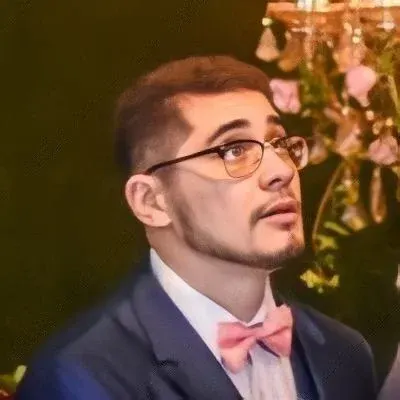
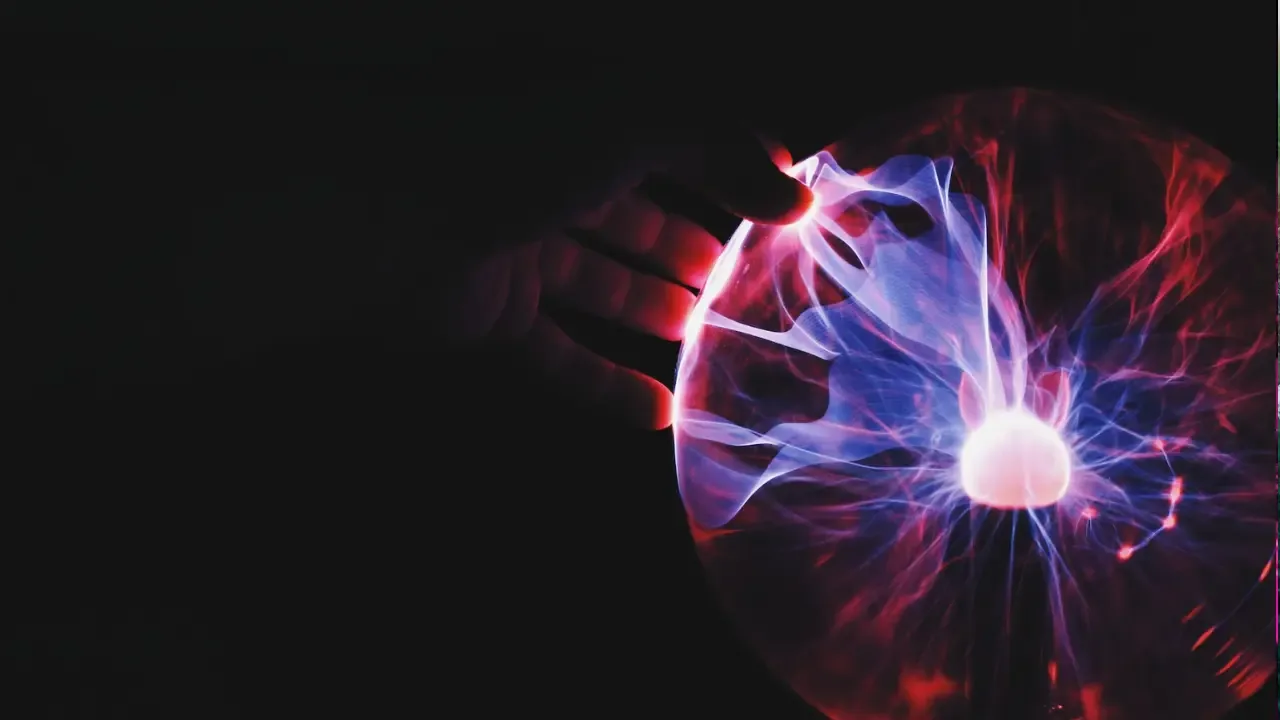
🎲 Generating Random Whole Numbers in JavaScript 🎲
So you want to generate some random whole numbers in a specific range in JavaScript, huh? 🤔
Generating random numbers is a pretty common need in programming, and luckily, JavaScript provides some nifty built-in functions to help us out!
🌌 The Problem: Generating Random Whole Numbers in a Range 🌌
Let's say you have two variables - x
and y
- and you want to generate a random whole number between those two values. For example, with x = 4
and y = 8
, you'd want to output any number from 4
to 8
, like 4, 5, 6, 7, 8
.
Sounds simple, right? But it can get a bit tricky if you don't know the right approach. Let's dive into some solutions!
💡 Solution 1: Math.random() and Math.floor() Combo 💡
One common approach is to use the Math.random()
method, which generates a random decimal between 0 (inclusive) and 1 (exclusive). To convert this decimal into a whole number within your desired range, you can multiply it by the difference between the upper and lower bounds and then add the lower bound value.
const x = 4;
const y = 8;
const randomNum = Math.floor(Math.random() * (y - x + 1)) + x;
console.log(randomNum); // Output: Random whole number between 4 and 8
Here's what's happening 🔎:
(y - x + 1)
calculates the range betweenx
andy
, inclusive.Math.random()
generates a random decimal between 0 and 1.Multiplying
Math.random()
by the range gives us a decimal within the desired range.Math.floor()
rounds down the decimal to the nearest whole number.Finally, we add
x
to shift the range to the desired position.
Voila! 🎉 You have your random whole number within the specified range!
💡 Solution 2: A Custom Function for Convenience 💡
If you find yourself needing to generate random numbers within a specific range frequently, you can create your own reusable function. This way, you won't have to repeat the above code snippet every time.
function getRandomNumberInRange(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
const x = 4;
const y = 8;
const randomNum = getRandomNumberInRange(x, y);
console.log(randomNum); // Output: Random whole number between 4 and 8
Ahh, much cleaner! Now you can simply call getRandomNumberInRange()
with your desired range whenever you need a random whole number.
📣 Your Turn: Let's Get Random! 📣
Now that you know how to generate random whole numbers in JavaScript, go ahead and try it out in your own projects! Experiment with different ranges and incorporate randomness into your code like a pro! 🎩✨
And don't forget to share your experience and any cool use cases in the comments below! Let's build a vibrant community of random-loving JavaScript developers! 🚀💬
Happy coding! 😄👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
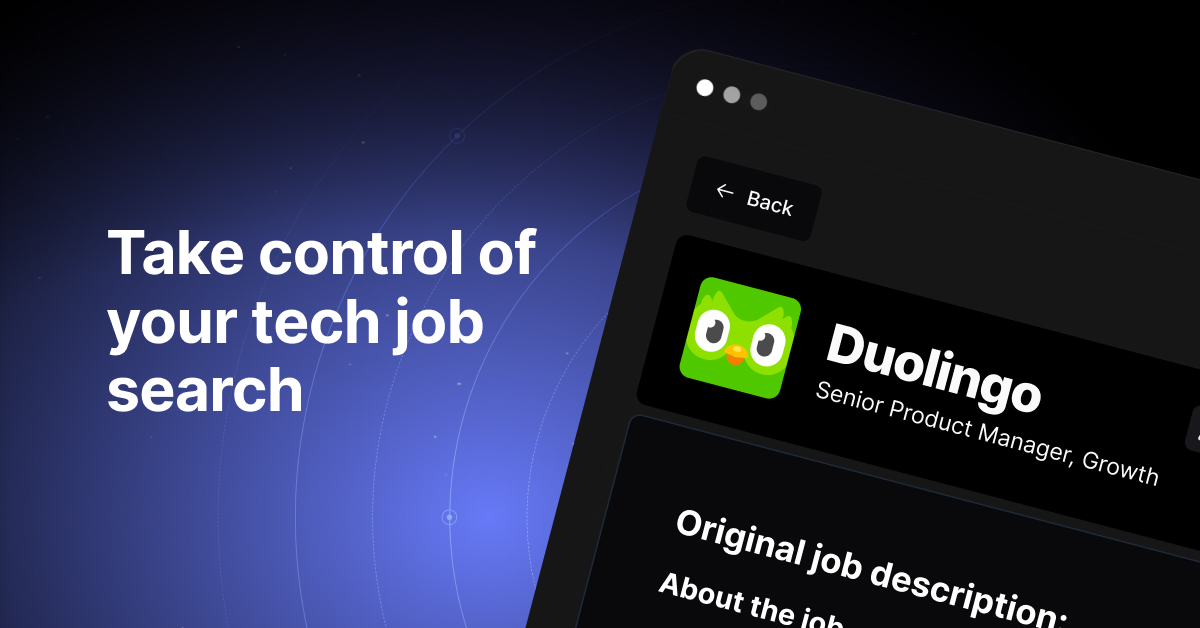