Generate random string/characters in JavaScript
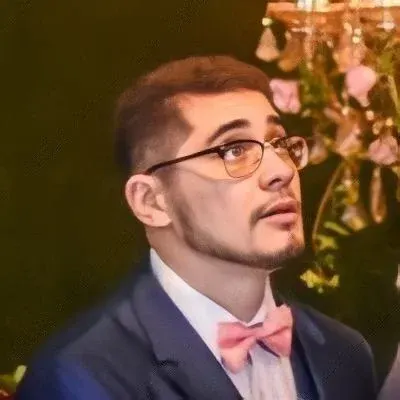
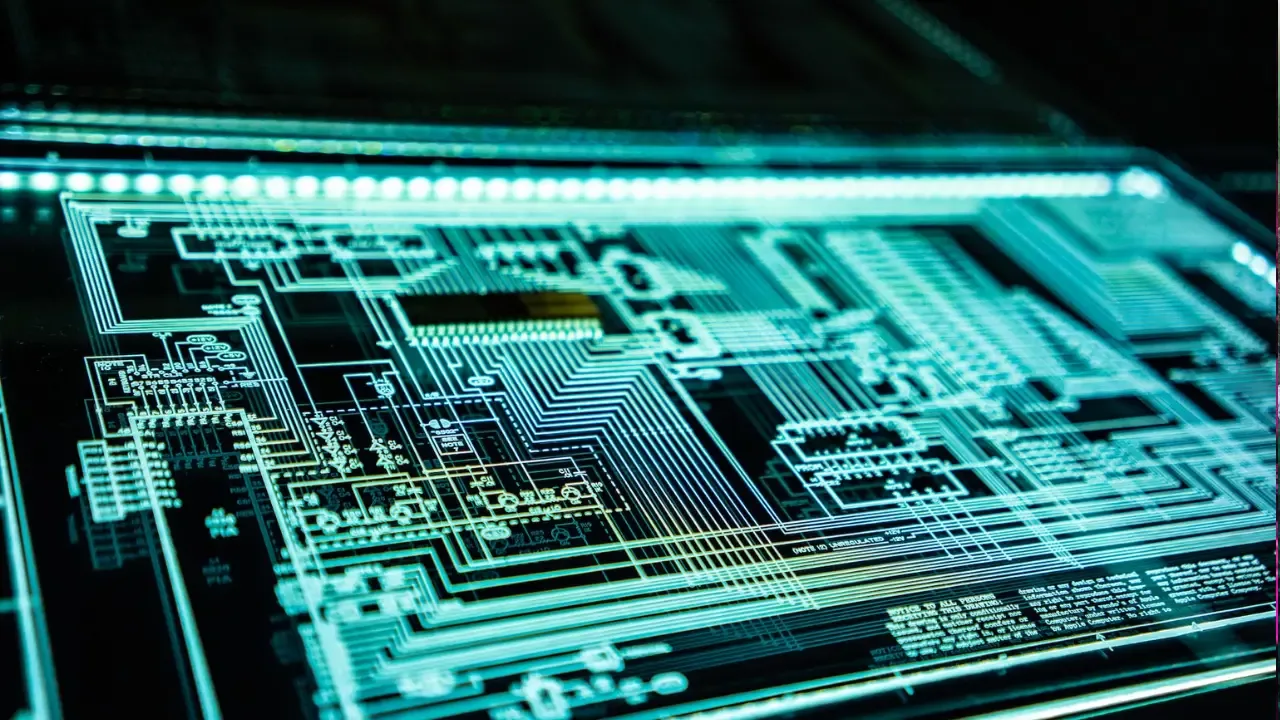
🎉 Easy Random String Generation in JavaScript! 🎉
So you want to generate a random string of 5 characters that includes uppercase letters, lowercase letters, and numbers. Let's dive right in and find the best approach using JavaScript!
The Challenge: Creating a Random String
The goal is to generate a random string by selecting characters randomly from the set [a-zA-Z0-9]
. We want to generate a 5-character long string, so let's get started.
Solution 1: Utilizing Math.random() and charAt()
One approach is to take advantage of JavaScript's Math.random()
function, along with the String.charAt()
method. Here's the code:
function generateRandomString(length) {
const characters = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789';
let result = '';
for (let i = 0; i < length; i++) {
const randomIndex = Math.floor(Math.random() * characters.length);
result += characters.charAt(randomIndex);
}
return result;
}
const randomString = generateRandomString(5);
console.log(randomString); // Output example: "5xY9A"
In this solution, we create a string characters
that contains all the possible characters we want to choose from. Then, in a for loop, we generate a random index and use charAt()
to grab a character from the characters
string. We repeat this process length
number of times and concatenate each randomly chosen character to the result
string. Finally, we return the generated random string.
Solution 2: Utilizing Math.random() and Array.from()
Another option is to use Array.from()
and the Math.floor()
function to achieve a similar result. Here's the code:
function generateRandomString(length) {
const characters = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789';
const randomString = Array.from({ length }, () => {
const randomIndex = Math.floor(Math.random() * characters.length);
return characters.charAt(randomIndex);
}).join('');
return randomString;
}
const randomString = generateRandomString(5);
console.log(randomString); // Output example: "v3N0f"
In this solution, we use Array.from()
to create an array of length length
. We then use the second argument of Array.from()
to specify a function that generates a random character by following a similar process as Solution 1. Finally, we use join('')
to convert the array into a string.
Power in Simplicity! 🚀
Both solutions provide easy and effective ways to generate a random string using JavaScript. Feel free to choose the one that suits your preference or coding style!
🌟 Your Turn: Try it out! 🌟
Go ahead and try out the code snippets provided. Experiment with different lengths and characters to generate unique random strings. Let us know in the comments below what cool uses you find for random strings in your projects!
If you have any questions or alternative solutions, we'd love to hear from you. Happy coding! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
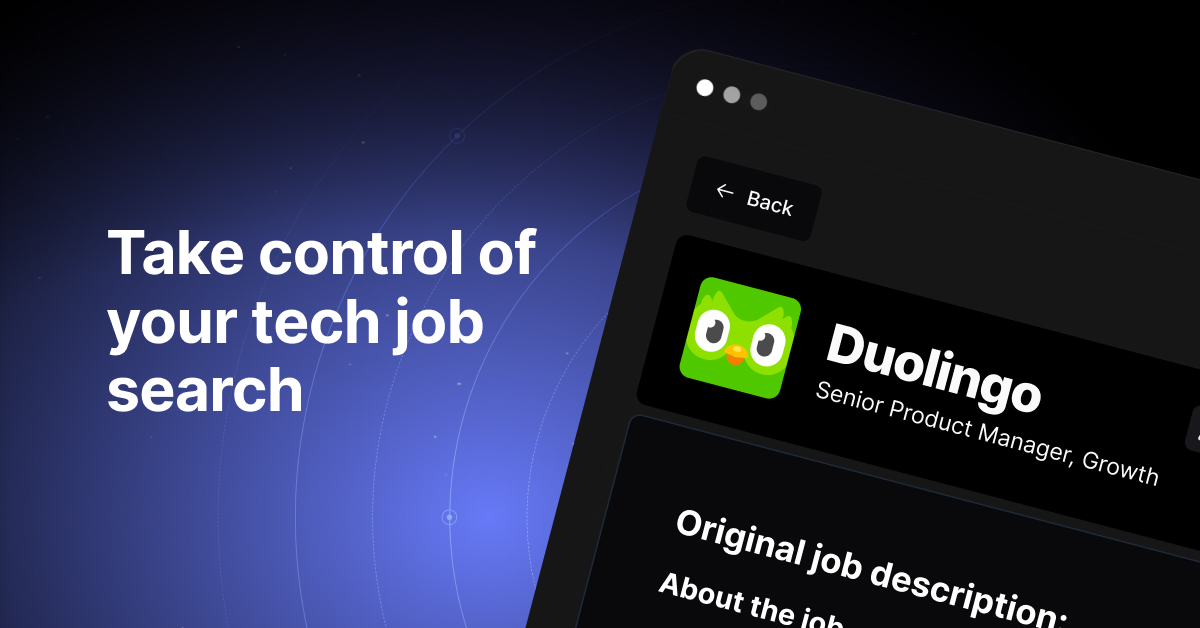