Generate random number between two numbers in JavaScript
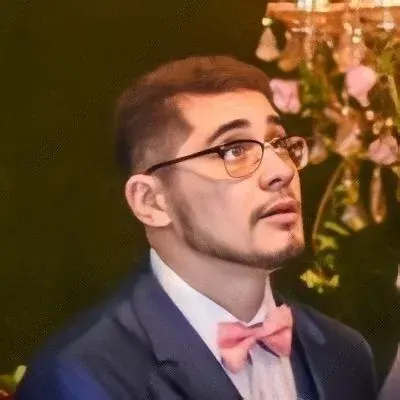
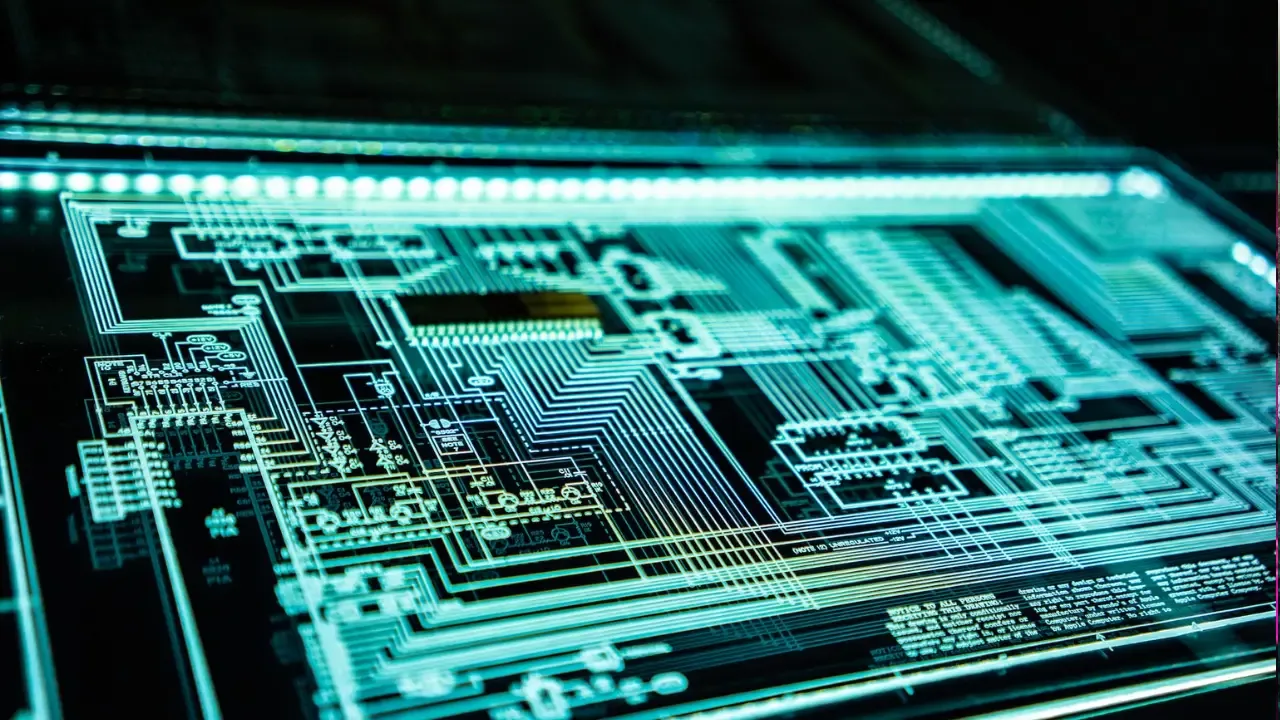
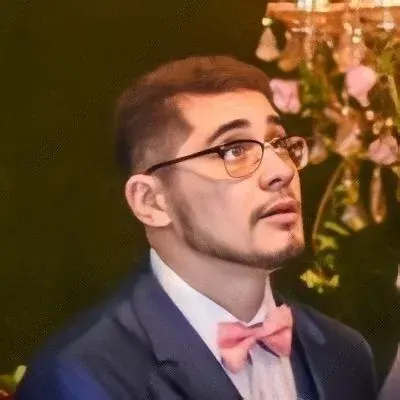
Generating Random Numbers between Two Numbers in JavaScript
๐ข Are you looking for a way to generate a random number within a specified range using JavaScript? Look no further! In this blog post, we'll dive into the common issue of generating random numbers between two values and provide you with easy solutions to solve this problem. ๐ก
The Scenario
๐ Let's say you want to generate a random number between 1 and 6, where the result could be any of the numbers 1, 2, 3, 4, 5, or 6. This is a common requirement in various applications, such as games, simulations, or randomized selection processes.
๐ญ Now, you might be thinking about how to approach this problem. Fear not! We've got you covered with some straightforward solutions.
Solution 1: Using Math.random() and Math.floor()
โน๏ธ Math.random() is a function in JavaScript that generates a random number between 0 and 1 (excluding 1). By multiplying it with the difference between the upper and lower bounds, we can obtain a random number within the desired range. However, the result might be a decimal number, so we need to round it down to the nearest whole number.
Here's an example of how you can implement this solution:
function getRandomNumber(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
const randomNumber = getRandomNumber(1, 6);
console.log(randomNumber); // Output: Random number between 1 and 6
๐งช Let's break down this code:
The
getRandomNumber
function takesmin
andmax
as parameters, representing the lower and upper bounds of the range.We calculate the range by subtracting
min
frommax
and adding 1 to include bothmin
andmax
in the possible results.Math.random()
generates a random decimal number between 0 and 1 (excluding 1).We multiply the random number with the range to obtain a random number within the desired interval.
By adding
min
to the result, we shift the range to start frommin
rather than 0.Finally,
Math.floor()
rounds down the random number to the nearest whole number, ensuring we get an integer as the result.
Solution 2: Using the RandomInt API (ECMAScript 2022)
๐ In ECMAScript 2022, a new RandomInt
API will be introduced. This API allows you to directly generate random integers within a specified range without the need for additional calculations.
โ ๏ธ Keep in mind that this solution is not yet widely supported in all browsers or JavaScript environments. Check for browser compatibility before using this approach in production code.
Even though this API is not widely available yet, here's a glimpse of how it will work:
const randomNumber = Math.RandomInt(1, 6);
console.log(randomNumber); // Output: Random number between 1 and 6
Get Randomizing!
๐ซ There you have it - two easy solutions to generate random numbers within a specified range using JavaScript. Now you can leverage these techniques in your projects, whether you're building games, simulations, or any application that requires randomization.
๐ What are you waiting for? Give it a try and add some randomness to your code! Feel free to share your experiences or other creative ways you've used random numbers in the comments below. Happy coding! ๐ป๐ฒ
โ What other JavaScript topics would you like us to cover? Let us know and stay tuned for more tutorials and helpful guides. Don't forget to share this post with your fellow developers!